In our last articles, we learned how to use Streamlit to make websites with Python. We covered how to install it and show data in cool ways. Now, it’s time to put what we’ve learned into action. In this tutorial, we’ll make a basic calculator app using Streamlit. Let’s start!
Steps to Create a Calculator Using Streamlit
Now we will create an application using Python’s Streamlit library. This application is a simple calculator that operates within a web browser. With Streamlit, we can build interactive web apps effortlessly.
The purpose of this calculator app is to allow users to input a mathematical expression and obtain the result instantly. It’s a straightforward tool designed for quick calculations.
Here’s how it works: Users enter a mathematical expression in the provided text box, such as “2+3*4”, and then click the “Calculate” button. The app will evaluate the expression and display the result on the screen.
Let’s see the procedure step by step.
Step 1: Importing Streamlit
import streamlit as st
This line brings in Streamlit, a tool we’ll use to create our web app. We call it st to make it easier to use later.
Step 2: Customizing Layout
st.set_page_config(
page_title="Simple Calculator",
page_icon=":1234:",
layout="centered",
initial_sidebar_state="expanded",
)
Here, we set up how our web page will look. We give it the title “Simple Calculator”, add a small icon, and decide to center everything on the page. We also made the sidebar initially expanded.
Step 3: Adding Title and Input Field
st.title("🧮 Simple Calculator")
input_exp = st.text_input("Enter a valid calculation:", help="e.g., 2*3+5")
This part creates a big title at the top of our app saying “Simple Calculator“. Then, it adds a box where users can type in their math problems. The help part gives an example to guide users.
Step 4: Defining Calculation Function
def calculate(input_text):
try:
output = eval(input_text)
return output
except Exception as e:
return f"Error: {e}"
Here, we make a function called calculate. It takes the user’s math problem as input and tries to solve it using Python’s eval function. If there’s any problem, it catches it and shows an error message.
Step 5: Adding Calculate Button
if st.button("Calculate"):
with st.spinner("Calculating..."):
result = calculate(input_exp)
st.success("Done!")
st.write(f"**Result:** {result}")
This last part adds a button labelled “Calculate”. When users click it, the app calculates the answer to the math problem they typed. While it’s calculating, it shows a spinning icon. When it’s done, it shows the result on the screen. If there’s any problem, it shows an error message instead.
Complete Code
Here is the complete code of the created application:
import streamlit as st
# Customizing Streamlit layout
st.set_page_config(
page_title="Simple Calculator",
page_icon=":1234:",
layout="centered",
initial_sidebar_state="expanded",
)
# Title and input
st.title("🧮 Simple Calculator")
input_exp = st.text_input("Enter a valid calculation:", help="e.g., 2*3+5")
# Function to calculate the input expression
def calculate(input_text):
try:
output = eval(input_text)
return output
except Exception as e:
return f"Error: {e}"
# Button to trigger calculation
if st.button("Calculate"):
with st.spinner("Calculating..."):
result = calculate(input_exp)
st.success("Done!")
st.write(f"**Result:** {result}")
Output:
We will execute this program by running the following command:
streamlite run Calculate.py
A webpage like this will appear:
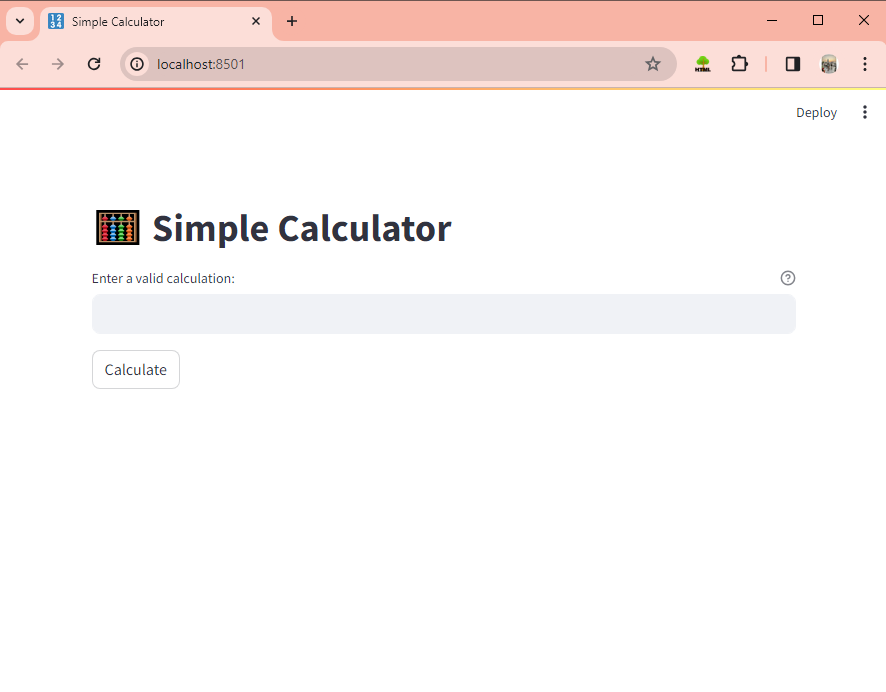
We will see a big title at the top saying “Simple Calculator“. Below the title, there’s a box where you can type in a math problem, like “2+3*4”. There’s also a hint inside the box suggesting an example calculation.
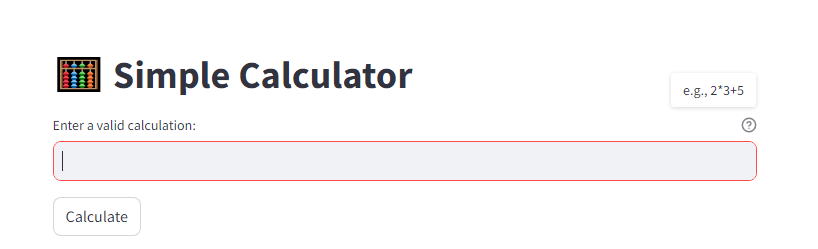
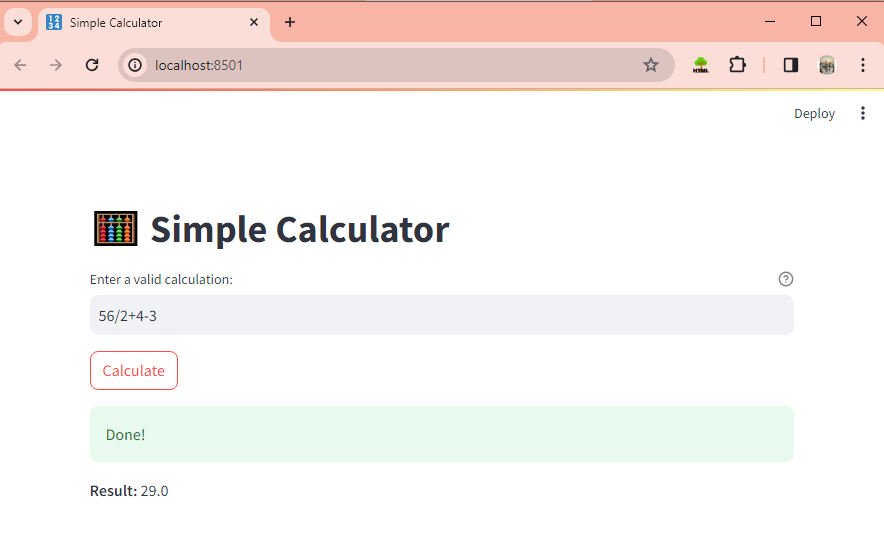
As you input your math problem and hit enter or press the calculate button, the result will swiftly appear below, allowing for quick and easy calculations on the fly.
Summary
Hope you have followed each step! We learned to build a basic calculator app using Streamlit in Python. Users input a math problem, and the app swiftly calculates the result. We customized the layout, added a text box for input, and implemented a calculation function. Finally, we ran the program and interacted with the calculator on a web page.
Further Reading –
Reference
https://stackoverflow.com/questions/72443689/python-basics-calculator-in-streamlit