Some words in each language are taboo to be used in the mainstream. These words rarely come by unless you are amidst a hostile situation. These kinds of words in programming are called Keywords.
What do they mean? The words falling under this category are given a special privilege, the means by which they could only be summoned by the program & not by the programmer.
The programmer should refrain from imposing their own values on these words such as declaring them as variables. Why you ask? Because these words have been entrusted with their own set of instructions to be carried out.
Below in this article are some notable keywords explained with their corresponding functions. Beware, some of these keywords are sensitive – case sensitive!
Most Common Python Keywords
Some of the basic keywords needed to perform common tasks are given below. We’ll focus mainly on those required to perform fundamental operations, and you’ll of course encounter them through a Python program.
and
This keyword is a logical operator that combines conditional statements and returns True if both statements are true.
Example:
if 5 > 3 and 5 < 10:
print("Both statements are True")
else:
print("At least one of the statements are False")
Output:
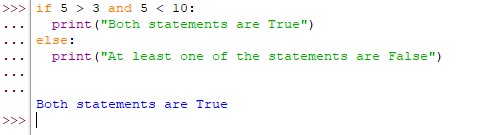
as
Having the desire to create an alternate for variables or modules or whatsoever you like? You might be looking for an as.
Example:
import calendar as c
print(c.month_name[1])
Output:
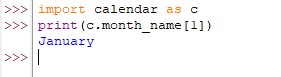
break
When there is a need to break out of iterations such as the for loop or the while loop, then break is the keyword to be used. Also to be noted are the words for and while, which stand for running iterations.
Example 1:
for i in range(10):
if i > 5:
break
print(i)
Output:
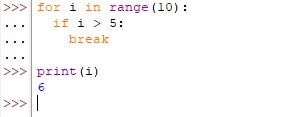
Example 2:
i = 2
while i <=10:
print(i)
if i == 4:
break
i += 1
Output:
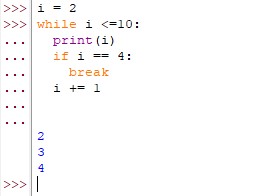
assert
Assert is the keyword to be used when one likes to debug a code. It will test a condition to verify whether it is True, or else it gifts us an assertion error.
Example:
a = -3
b = 10
perimeter = 2*(a+b)
assert a>0 and b>0
Output:
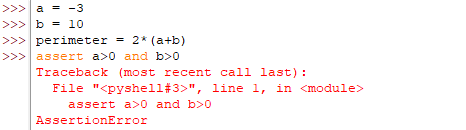
class
This keyword is used to define a class which is then used to construct an object which would make it way easier for the coder to call its constituents elsewhere throughout the program.
Example:
class Poland:
continent = "Europe"
Capital = "Warsaw"
Output:
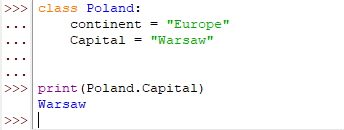
continue
The continue keyword is used to tell Python to skip the current iteration in a for or a while loop and continue to the next iteration, usually when a particular condition is met.
Example:
for i in range(5):
if i == 2:
continue
print(i)
Output:
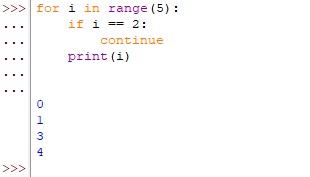
def
When one is after creating a custom-defined function, then that can be done in Python only by using the keyword def.
Example:
def perimeter(x,y):
print("Perimeter of rectangle:", 2*(x+y))
a = 10
b = 5
perimeter(a,b)
Output:
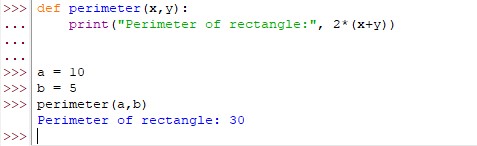
else
When a given condition is not met & one would like the program to execute a set of instructions then the keyword that comes to the rescue shall be else.
Example:
a = 10
if a>5:
print("Value is too big")
else:
print("Area of square:",a^2)
Output:
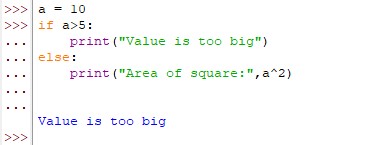
try, except & finally
These words – try and except fall under the category of error handlers where they lay the terms for what ought to be done when encountering with an error. The word finally executes the set of instructions whatsoever the outcome might be.
Example:
try:
ip = input("Number of your choice:")
ip = ip/10
print(ip)
except:
print("Invalid Input")
finally:
print("Thanks! Come again")
Output:
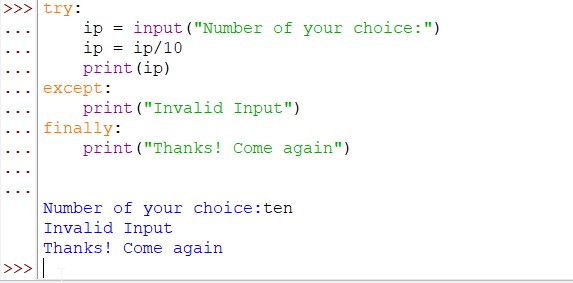
import & from
This is an interesting keyword that is used to extract and slice out only that which is needed from a lumpsum pile such as importing time from the datetime module for instance. That being said, import is another keyword that facilitates the extraction.
Example:
from datetime import time
x = time(hour=17)
print(x)
Output:
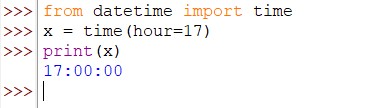
in
This keyword is used to verify whether a value is present in the given sequence. At times, it also finds usage to iterate through the for loop.
Example:
vegetables = ["carrot", "radish", "cucumber"]
if "carrot" in vegetables:
print("yes")
Output:
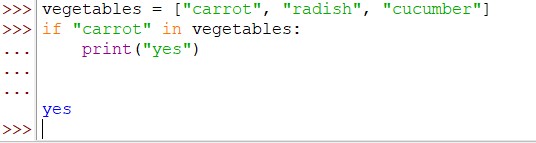
Conclusion
Now that we have reached the end of this article, hope it has elaborated on some of the notable keywords used in Python. Here’s another article that can be our definitive guide to the map function in Python. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help for you to gain valuable insights. Carpe diem!