The world is changing day by day and so is our Node.js. With the upcoming newer versions of Node, legitimate changes are made to add-on improvements. Due to this while using certain packages and modules we need to be very careful with the new syntax that is brought by the new version of the Node or else we will end up getting errors. “Cannot find module ‘node:fs'” is also a result of such incompatibility. Let’s see how to resolve this error. Since this error is directly related to the Node fs module, let’s first see a short introduction to it.
Introduction to Node.js File System Module
The fs module in Node.js is all about dealing with the file system. It provides an API for interacting with the file system and lets you do things like reading from or writing to files, creating or deleting files and folders, and adjusting file permissions. Overall it’s a handy tool for managing your system’s files.
Import Syntax:
const fs = require('fs');
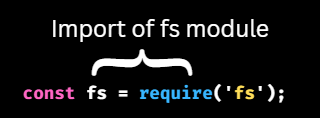
Here are some things where the fs module proves to be efficient:
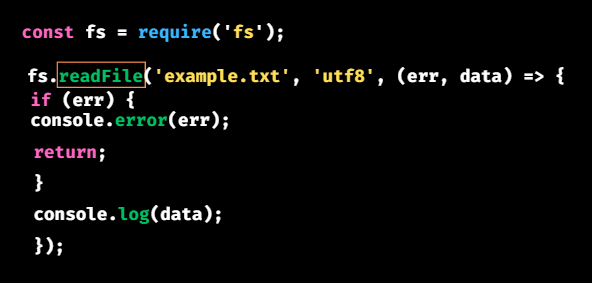
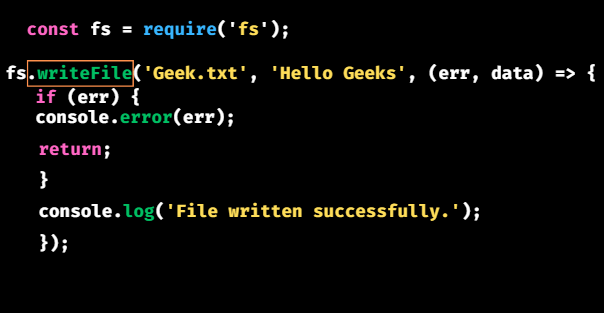
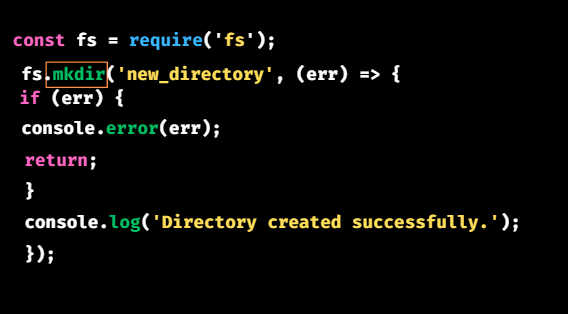
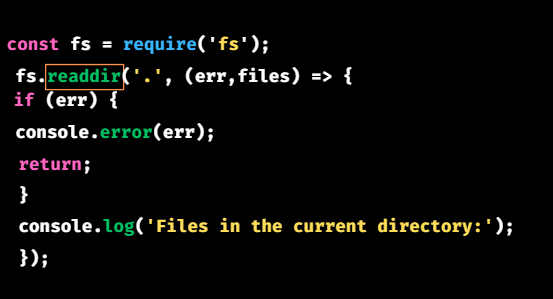
You may look here for more such methods of fs: Node.js File System
Understanding the Error Message
The message “Cannot find module ‘node:fs'” means your Node.js app is looking for the ‘fs’ (file system) module but can’t find it. For better understanding let’s take an example.
Consider the following JavaScript code:
const fs = require('node:fs');
fs.writeFile('Geek.txt', 'Hello and welcome to CodeForGeeks!', (err) => {
if (err) {
console.error(err);
return;
}
console.log('File written successfully.');
});
Output:
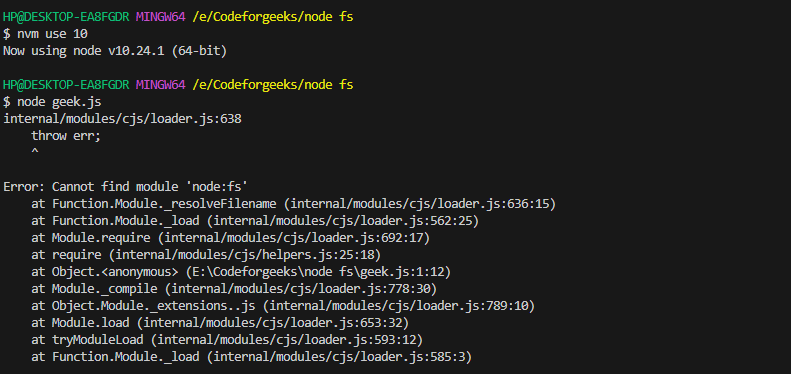
We get the “Error: Cannot find module ‘node:fs'”. This happens when you try to use the ‘node:’ prefix to import the ‘fs’ module in Node.js, but newer Node.js versions don’t allow this syntax.
Using ‘node:’ as a prefix to import the ‘fs’ module in Node.js is not a standard practice and can cause errors or unexpected behaviour.
This error occurs here because we have used node version 10 which is not compatible with the given syntax of require(‘node:fs’). Now let’s see how to solve it.
Fixing Cannot find module ‘node:fs’ Error
There are two major methods you can try to fix this error.
Method 1: Changing the Syntax of FS
Suppose your program is like a person asking for a tool in a toolbox. Instead of saying “Can I have the tool called ‘fs’?” (which is correct), it’s mistakenly saying “Can I have the tool called ‘node:fs’?” (which is incorrect).
To fix this, your program needs to ask for the ‘fs’ tool in the right way. So, instead of saying “node:fs” it should just say “fs”. In code language, it means changing const fs = require(‘node:fs’); to const fs = require(‘fs’);. This way, Node.js can understand what tool your program is asking for, and it won’t give you that error anymore.
const fs = require('fs');// changed syntax
fs.writeFile('Geek.txt', 'Hello and welcome to CodeForGeeks!', (err) => {
if (err) {
console.error(err);
return;
}
console.log('File written successfully.');
});
Output:
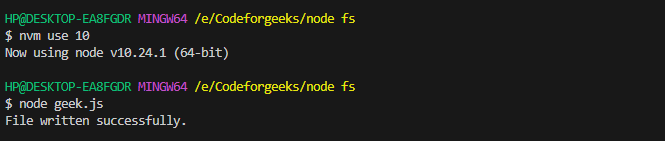
Method 2: Changing the Node.js Version
If the first method doesn’t work, consider using a different version or switching to a compatible version of Node.js. Sometimes, the version you’re using might not understand how you’re asking for something, like ‘fs’. Switching to a more suitable version can help your code align with the language features and support the modules by the runtime. However, you need to check your application’s compatibility with the chosen Node.js version before switching.
If you want to learn how to change the node version then do check out: How to Downgrade Node Version: 3 Easy Ways
Let’s see the result after changing Node.js to a compatible version:
const fs = require('node:fs');//no change in syntax
fs.writeFile('Geek.txt', 'Hello and welcome to CodeForGeeks!', (err) => {
if (err) {
console.error(err);
return;
}
console.log('File written successfully.');
});
Output:
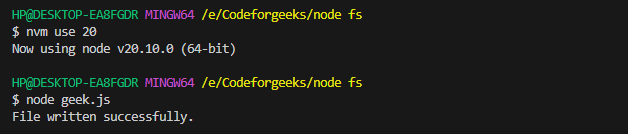
Here, instead of changing the syntax we have changed the Node version.
Conclusion
And we come to an end! In short, with the evolution of Node.js, compatibility issues may arise with newer versions, leading to errors such as “Cannot find module ‘node:fs’. This specific error is linked to the Node.js File System (fs) module, an important module for file system interactions. In this article, we tried to emphasize the importance of following standard practices while importing modules to avoid errors and bugs in our project. I hope this piece of information makes you clear about the fs modules and the solution to their error.
If you liked this explanation consider reading more of our troubleshooting content:
- How to fix “ReferenceError: primordials is not defined” in Node.js
- NodeJS Errors: List of 6 Types of NodeJS Errors