Nodemon using TypeScript can help developers to have a more enhanced experience in terms of development by ensuring that they have an effective and automated way of monitoring and restarting their Node.js applications whenever changes are made.
In this Node.js tutorial, we will walk you through setting up Nodemon using TypeScript in your Node.js projects.
Also Read: How to use TypeScript in NodeJS and ExpressJS Project
Introduction to Nodemon
Nodemon is a tool that assists in restarting your Node.js script whenever you make changes in your source code. This can be of great use during development as it saves you from having to stop and start your server manually every time you change something.
When using Nodemon, you replace the “node” command with “nodemon” to automatically monitor changes in your files and restart the Node.js server.
Server starting with Node.js:
node index.js
Server starting with Nodemon:
nodemon index.js
We recommend for an in-depth exploration of Nodemon and its features, visit – Update Code Without Restarting Node Server
Setup Nodemon for TypeScript
Let’s create a very simple express project to demonstrate the use of Nodemon.
Prerequisites:
- Basic knowledge of Node.js, Express.js & TypeScript
- Node.js version 12 or above
Step 1: Initialize your Node.js project:
npm init -y
Step 2: Installing dependencies:
npm install express
npm install typescript ts-node @types/node @types/express --save-dev
In these steps, we have installed an express framework for our project. We are also installing TypeScript-related dependencies as devDependencies, as you may have seen. Therefore, since we are just utilizing TypeScript during development, we do not necessarily need to utilize it during runtime.
Important Note: The ” –save-dev ” flag indicates that these packages are development dependencies.
Step 3: Installing Nodemon using the following command:
npm i -g nodemon
We recommend you install it globally so that you don’t have to manually install it on each Node.js project directory.
Step 4: Unlike other packages, TypeScript is not yet usable even when you installed it. Now we have to generate “tsconfig.json” file and configure it.
tsc --init
This above command will generate a “tsconfig.json” file with default settings. You have to open this file and make the required changes for your server.
You can also create a file by the name of “tsconfig.json” and copy and paste the following code snippets into it:
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"rootDir": "./src",
"moduleResolution": "node",
"outDir": "./dist",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true
},
"include": ["./src/**/*"]
}
Step 5: Now we will create an “src” folder in your project directory and an “app.ts” file inside it as shown in the below image.
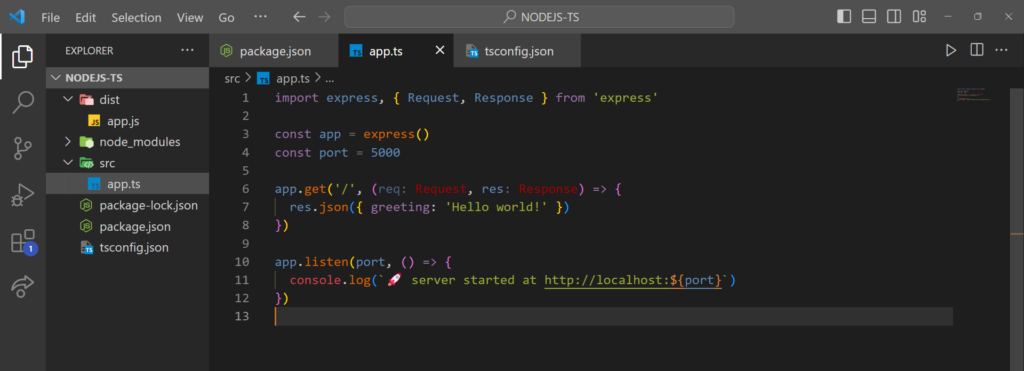
In the above example, we have created a simple server using Express.js. In which we created a basic route for the path (‘/’) using the “app.get” method. When the root path is made, the server responds with a JSON object and then a server is created on the specified port (5000). Finally, the server is set to listen on the specified port (5000). Once the server starts, a log message is printed to the console indicating that the server is running and accessible at “http://localhost:5000”.
Step 6: Update Package.json:
"scripts": {
"start": "nodemon --exec ts-node src/index.ts"
}
The script “start”: “nodemon –exec ts-node src/index.ts” in your package.json file is a convenient way to set up an npm script for starting your nodemon.
“–exec ts-node src/index.ts” – This section of the script instructs Nodemon to use the ts-node command to run your “src/index.ts” file. With the help of ts-node, a TypeScript execution environment for Node.js, you can run TypeScript code straight away without having to manually convert it to JavaScript.
Step 7: Run the project with the following command:
npm run dev
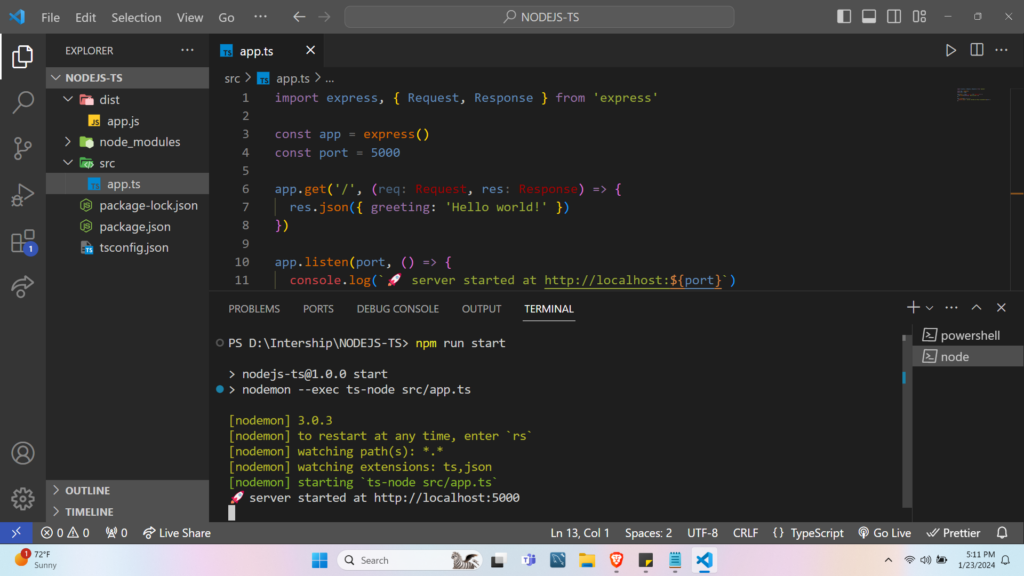
You are ready to go with the Nodemon using TypeScript setup for the Node.js project. ENJOY!!!
Conclusion
In this article, you learned that setting up Nodemon using TypeScript is a valuable and efficient process for enhancing development. Finally, we successfully set up a Nodemon + TypeScript project with an Express server.
If you are new to Node.js and Express.js, you can read this article to enhance your knowledge – Express Tutorial for Beginners: A Node.js Web Framework
Reference
https://www.npmjs.com/package/nodemon