The file system is used to interact with the files. Operations like creating, reading, and deleting files are done using File System in Node.js. Node.js File System is an inbuilt module that we can import inside our project directly to use it. It is not required to install unlike other Node.js modules like zlib etc.
In Node, it usually recommends using Asynchronous operation. Asynchronous means non-blocking i.e the operation does not block the execution of other operations. But sometimes we want a Synchronous approach such as we want to block other operations until a file is completed read so in this case it is recommended to use a Synchronous operation.
File System in Node.js has both Synchronous and Asynchronous methods to interact with files.
NodeJS File System Operations
- Read Files
- Write Files
- Append Files
- Delete Files
Setting Up The Environment
- Make sure that you have Node.js installed on your pc.
- Create a folder
- Inside this folder create a file app.js
- Inside this folder create a file hello.txt & write some content on it.
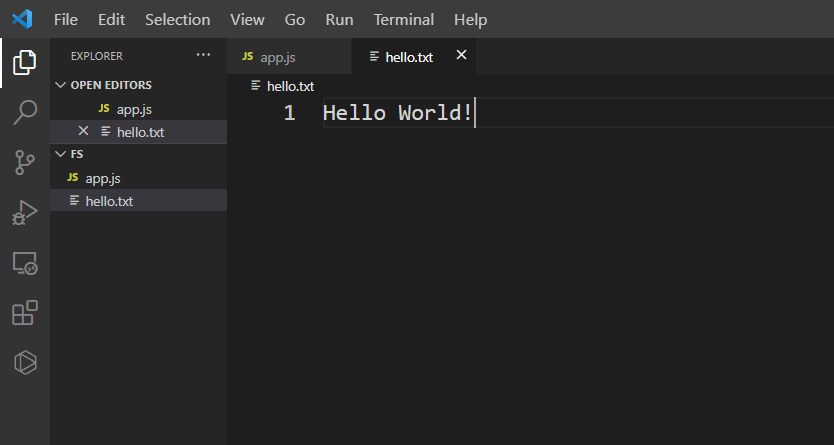
- Open app.js inside the code editor
- Input File System using the following syntax
Syntax:
const fs = require(“fs”);
Synchronous Methods in the NodeJS File System
1. readFileSync
This method is used to read the data of a file synchronously.
Syntax:
readFileSync(path, encoding);
Example:
const fs = require('fs');
const data = fs.readFileSync('hello.txt', 'utf-8');
console.log(data);
Output:
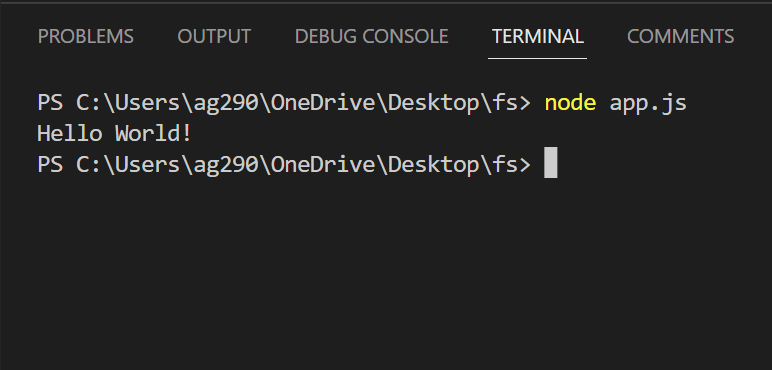
2. writeFileSync
This method is used to write data on a file synchronously. If the file does not exist it creates that file and then writes on it. If the file exists, then it will override the data.
Syntax:
writeFileSync(path, data, encoding);
Example:
const fs = require('fs');
const data = fs.writeFileSync('hello2.txt', 'Writing on File', 'utf-8');
Output:
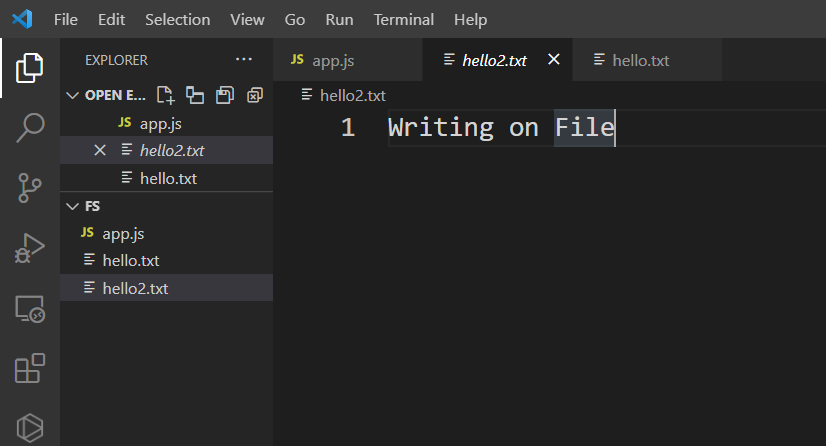
3. appendFileSync
This method is used to append data to a file synchronously, unlike the writeFileSync method which overwrites the data of a file.
Syntax:
appendFileSync(path, data, encoding);
Example:
const fs = require('fs');
const data = fs.appendFileSync('hello.txt', 'Writing on File', 'utf-8');
Output:
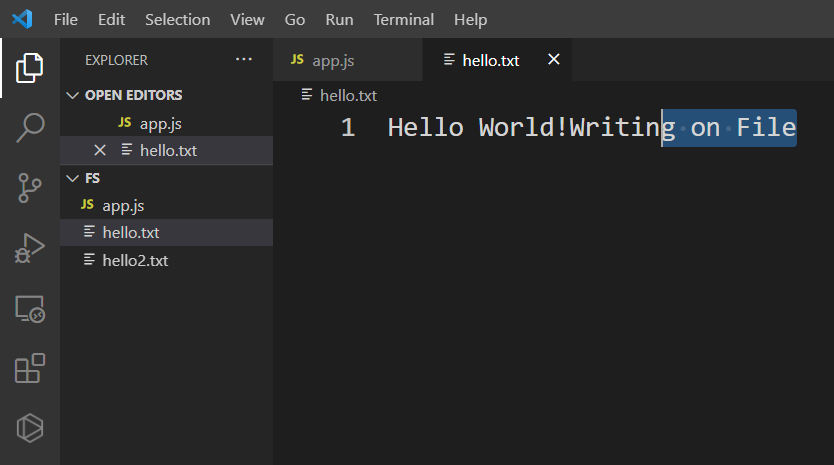
4. unlink
This method deletes the file synchronously.
Syntax:
unlinkSync(path);
Example:
const fs = require('fs');
const data = fs.unlinkSync('hello2.txt');
console.log("File Deleted");
Output:
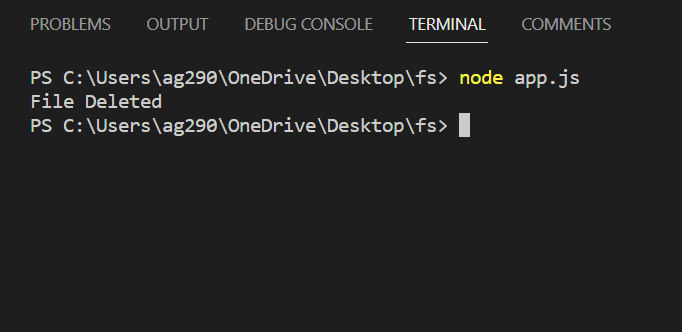
Asynchronous Methods in NodeJS File System Operations
Let’s now look at some of the async methods of working with files on NodeJS. These methods perform similar actions to the above synchronous methods.
1. readFile
This method is used to read the data of a file asynchronously.
Syntax:
readFile(path, encoding, callback);
Example:
const fs = require('fs');
const data = fs.readFile('hello.txt', 'utf-8', function(err, result){
if(err){
console.log(err)
}
else {
console.log(result)
}
});
Output:
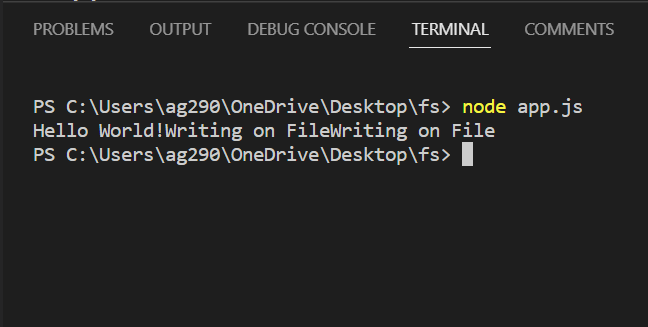
2. writeFile
This method is used to write data on a file asynchronously. If the file does not exist it creates that file and then writes on it. If the file exists, then it will override the data.
Syntax:
writeFile(path, data, encoding, callback);
Example:
const fs = require('fs');
const data = fs.writeFile('hello3.txt', 'Writing on File', 'utf-8', function(err){
if(err){
console.log(err);
}
});
console.log(data);
Output:
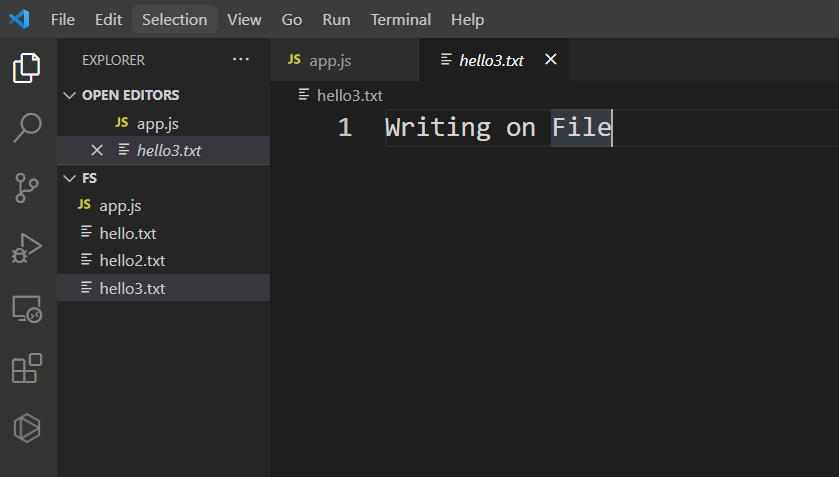
3. appendFile
This method is used to append data to a file asynchronously, unlike the writeFileSync method which overwrites the data of a file.
Syntax:
appendFile(path, data, encoding, callback);
Example:
const fs = require('fs');
const data = fs.appendFile('hello.txt', 'Writing on File', 'utf-8', function(err){
if(err){
console.log(err);
}
});
console.log(data);
Output:
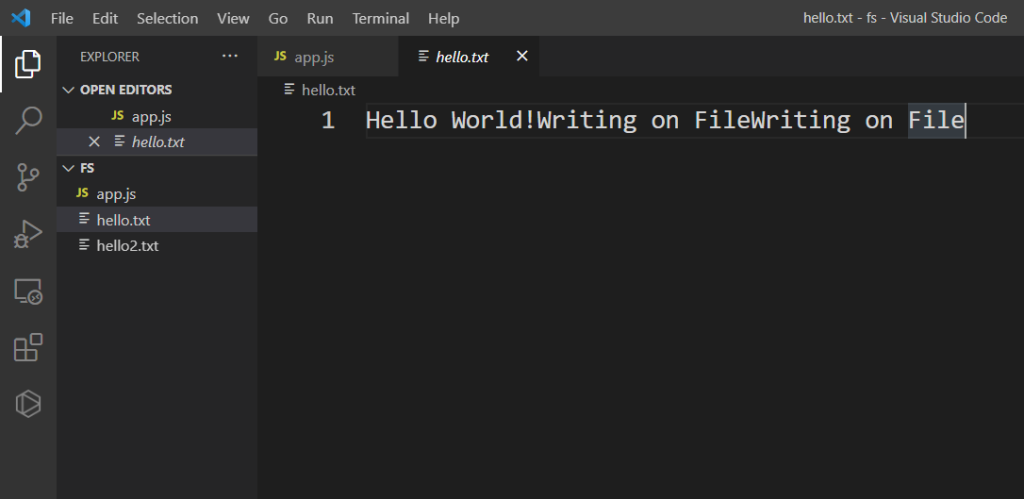
4. unlink
This method deletes the file asynchronously.
Syntax:
unlink(path, callback);
Example:
const fs = require('fs');
const data = fs.unlink('hello2.txt', function(err){
if(err){
console.log(err)
}else {
console.log("File Deleted");
}
});
Output:
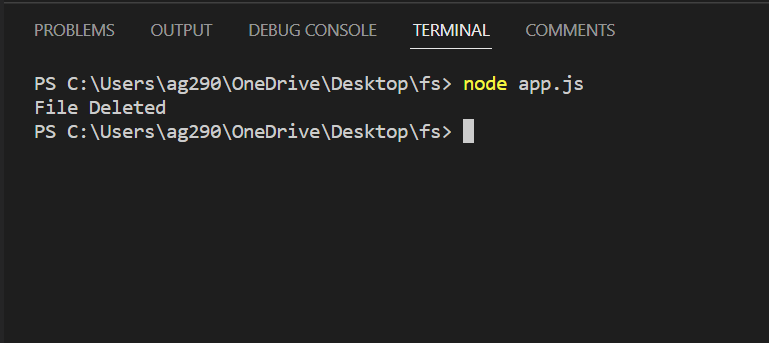
Summary
When creating any sort of application, the code has to interface with files and the NodeJS file system makes it extremely simple to create that interface. When done right, the NodeJS file system can be an easy way to create interactive and stateful applications. We hope this tutorial helped you understand the file system better.
Reference
https://nodejs.org/api/fs.html#file-system