Node.js provides an error object which indicates different types of errors. A programmer can throw the error in the console to debug the program. It helps to indicate which type of error occurs like syntax, reference, and type errors. It also gives a description of that particular error.
An error object is more useful when we are creating a callback for functions, it is recommended to first check for an error, and if it occurs then throw it and stop the flow of execution of the program if no error occurs then execute the rest of the program.
Example:
functionName(arguments, callback(error){
if(error){
throw error;
} else {
// callback body
}
} )
6 Types of Errors in NodeJS
Node.js provides a way to check the specific type of error and then perform different actions according to that. Let’s see them one by one.
1. Range Error
This error represents that the specified type of error is due to the particular value being more than the allowed range.
Example:
const arr = []
arr.length=123456*123456;
Here we are trying to increase the length of an array to outside its range.
Output:
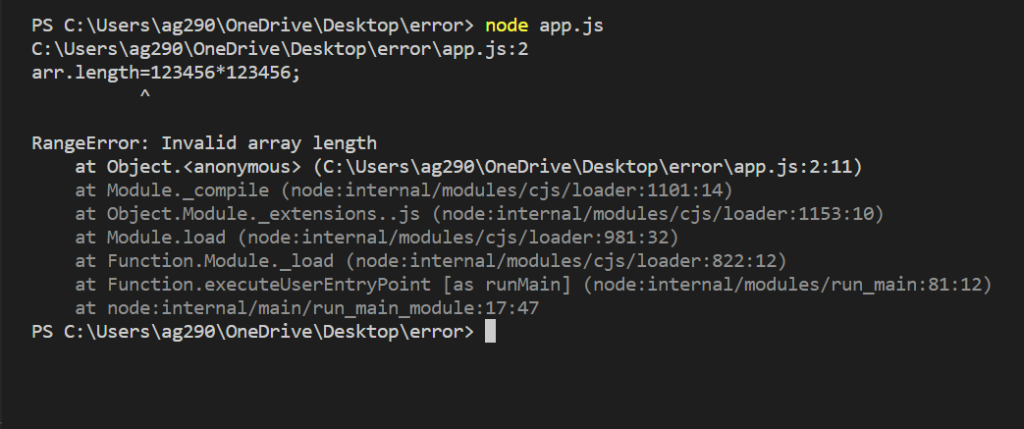
2. Reference Error
This error represents that the variable/object tried to access does not exist i.e wrong reference.
Example:
const first = "hello";
console.log(second);
Here we defined a constant first and set it to the string “hello”, but in the console, we mistakenly try to log the constant second which does not exist.
Output:
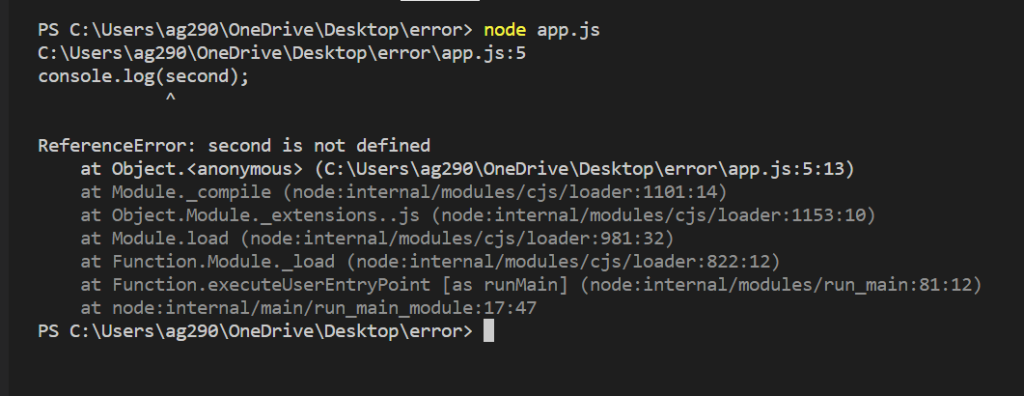
3. Syntax Error
This error represents that the syntax used is not supported in Node.js or is invalid.
Example:
constant first = "hello";
Here we are trying to initiate a constant by using the constant keyword rather than const, so Node.js is not able to understand it and returns a syntax error.
Output:
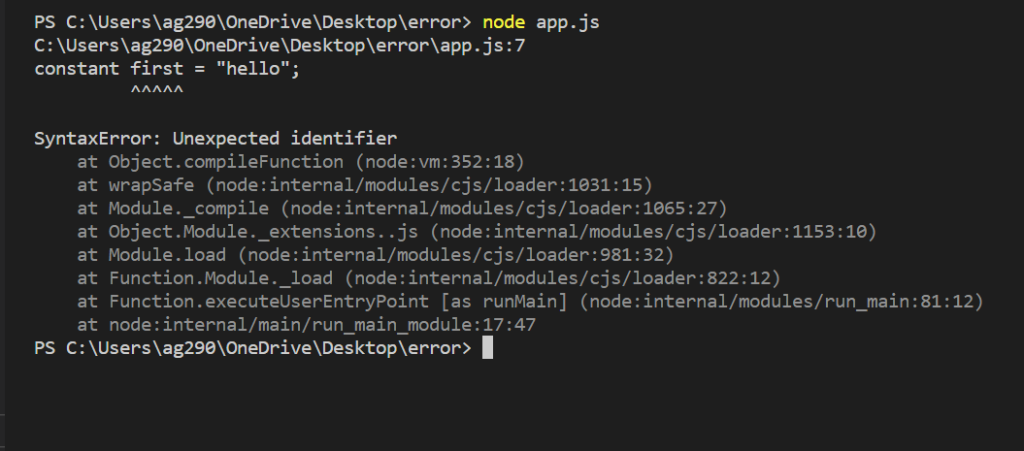
4. Type Error
This error represents that the type used for a particular operation differs from the type required for that operation.
Example:
const num = 123;
num.toUpperCase();
Here we are trying to convert a number into uppercase by using the toUpperCase method which requires a string.
Output:
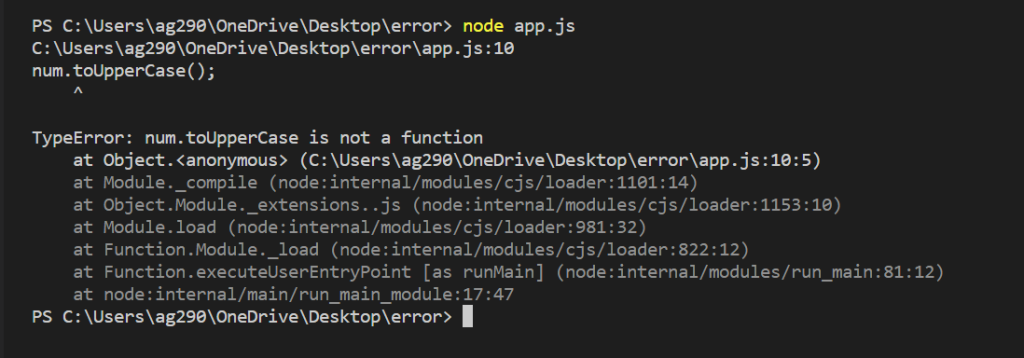
5. URI Error
This error represents that the way global URI handling functions are implemented is not valid.
Example:
decodeURI("%");
Here we are passing the “%” instead of a URI in the URI handling functions.
Output:
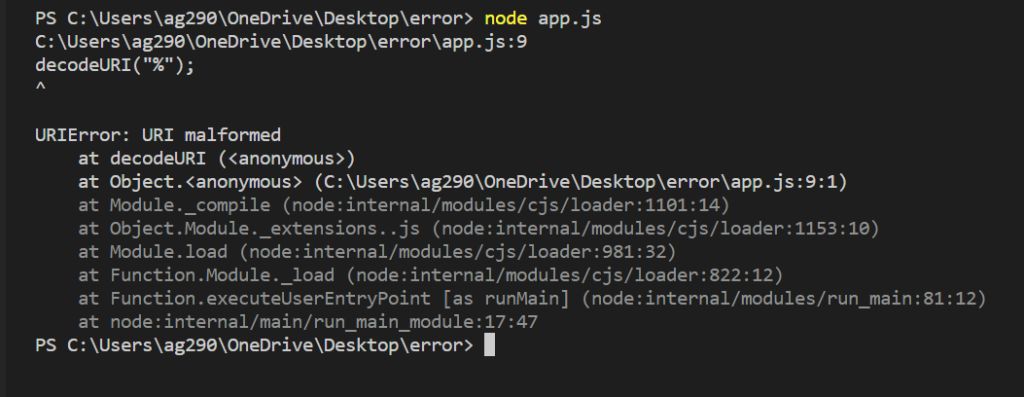
5. Internal Error
This error happens internally in the NodeJS engine when it is unable to handle the execution due to much data and the stack is crossed its maximum limit.
6. Custom Error
In Node.js custom error can be thrown by using the error constructor of the error class. The custom error passed during the creation of an error object from the error class.
Example:
const errorObj = new Error("Invalid");
errorObj.name = "Invalid URI";
errorObj.code = 404;
throw errorObj;
Output:
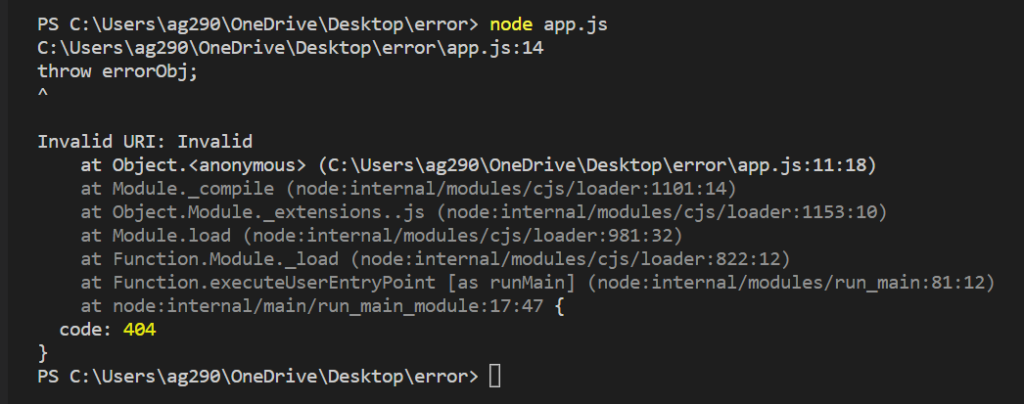
Summary
Error object used to throw the error. It shows the type of error that occurs which helps to detect the error specifically and debug the problem that causes the error. A program can define its own custom error using the Error class which contains an error constructor used to initial the value of the message, name, code, etc regarding the error. Hope this article helps you to understand the error in Node.js.
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Error
https://nodejs.org/api/errors.html