Welcome to another tutorial of our Tkinter series. Here, we will try to create a Random Mobile Number Generator using Tkinter. Tkinter has proved to be an excellent library for creating GUI applications. In this tutorial, we will see a step-by-step approach for creating an application using the same which generates a random mobile number. Sounds interesting? Let’s get started!
Steps to Create a Random Mobile Number Generator Application
As it is already clear that we are going to use the random function, let’s see how we can implement the logic and combine it with Tkinter to create this fun application. Let’s see step by step process.
Step 1: Importing Modules
Firstly, we will import the necessary modules for building the GUI and generating random numbers:
- tkinter: Provides tools for building graphical user interfaces.
- random: Allows generation of random numbers.
import tkinter as tk
import random
This import will only work if Tkinter is installed. If not, you can do so by running the given command:
pip install tk
Step 2: Define a Function for Generating Mobile Number
Then we will define a function called generate_number() to generate a random mobile number:
- random.choice(‘123456789’): Select a random digit from 1 to 9 for the first digit (ensuring it’s not zero).
- random.choices(‘0123456789’, k=9): Generates a string of 9 random digits.
- result_label.config(text=”Generated Mobile Number: ” + mobile_number): Updates the label text to display the generated mobile number.
def generate_number():
mobile_number = random.choice('123456789') + ''.join(random.choices('0123456789', k=9))
result_label.config(text="Generated Mobile Number: " + mobile_number)
Step 3: Create the Main Window
Now we will create the main window for the GUI application:
- tk.Tk(): Creates the main window.
- root.title(“Random Mobile Number Generator”): Sets the title of the window.
- root.configure(bg=”#CADCFC”): Sets the background color of the window.
root = tk.Tk()
root.title("Random Mobile Number Generator")
root.configure(bg="#CADCFC")
Step 4: Create a Label for Prompting User Action
Next, we will create a label prompting the user to take action:
- tk.Label(root, text=”Click on the button below”, bg=”#CADCFC”, font=(“Arial”, 12)): Creates a label widget.
- prompt_label.pack(pady=10): Packs the label onto the window with vertical padding.
prompt_label = tk.Label(root, text="Click on the button below", bg="#CADCFC", font=("Arial", 12))
prompt_label.pack(pady=10)
Step 5: Create a Button for Generating Mobile Number
Then we will create a button to trigger the generation of mobile numbers:
- tk.Button(root, text=”Generate Mobile Number”, command=generate_number, bg=”#00246B”, fg=”white”, font=(“Arial”, 12)): Creates a button widget.
- generate_button.pack(pady=5): Packs the button onto the window with vertical padding.
generate_button = tk.Button(root, text="Generate Mobile Number", command=generate_number, bg="#00246B", fg="white", font=("Arial", 12))
generate_button.pack(pady=5)
Step 6: Create a Label for Displaying Generated Mobile Number
Now we will create a label to display the generated mobile numbers:
- tk.Label(root, text=””, bg=”#CADCFC”, font=(“Arial”, 12)): Creates a label widget.
- result_label.pack(pady=10): Packs the label onto the window with vertical padding.
result_label = tk.Label(root, text="", bg="#CADCFC", font=("Arial", 12))
result_label.pack(pady=10)
Step 7: Run Event Loop
Finally, we will start the Tkinter event loop, which listens for user actions and updates the GUI accordingly:
- root.mainloop(): Runs the main event loop until the user closes the window, keeping the GUI application responsive.
root.mainloop()
Complete Code
import tkinter as tk
import random
def generate_number():
# Generating a random mobile number with 10 digits, ensuring the first digit is not zero
mobile_number = random.choice('123456789') + ''.join(random.choices('0123456789', k=9))
result_label.config(text="Generated Mobile Number: " + mobile_number)
# Creating the tkinter window
root = tk.Tk()
root.title("Random Mobile Number Generator")
root.configure(bg="#CADCFC")
# Creating a label prompting the user to click the button
prompt_label = tk.Label(root, text="Click on the button below", bg="#CADCFC", font=("Arial", 12))
prompt_label.pack(pady=10)
# Creating a button to generate a random mobile number
generate_button = tk.Button(root, text="Generate Mobile Number", command=generate_number,
bg="#00246B", fg="white", font=("Arial", 12))
generate_button.pack(pady=5)
# Creating a label to display the generated mobile number
result_label = tk.Label(root, text="", bg="#CADCFC", font=("Arial", 12))
result_label.pack(pady=10)
# Running the tkinter event loop
root.mainloop()
Output
When you run the code, a GUI window will appear with a light blue background.
The window will display a label prompting you to click a button below. Beneath the label, there will be a button labelled ‘Generate Mobile Number’.
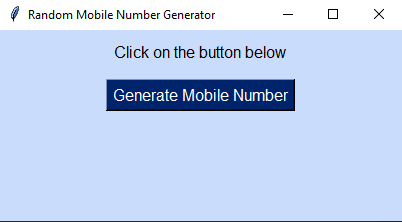
Clicking this button will generate a random 10-digit mobile number, ensuring the first digit is not zero. The generated number will appear below the button. You can click the button multiple times to generate different numbers, with each new number replacing the previously generated one.
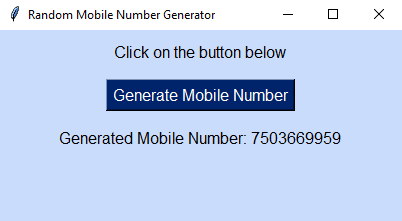
Conclusion
So that concludes this article. We hope this demonstration provided you with a real-life implementation of the random function in Python. Thanks to Tkinter, we were able to create an application using this logic. Now, we trust that you can create your own random number generators.
If you liked this one, consider reading –
Reference
https://stackoverflow.com/questions/72440892/printing-random-phone-numbers-in-a-range