When we work with Python, sometimes we need to use features from the computer’s operating system. The ‘os’ module in Python helps us do this. It lets us do things like find out the current directory, list files and folders, create or delete folders, and more.
In this article, we’ll look at some useful ‘os’ module functions that can be helpful for your projects.
OS Module in Python
Before getting into its most used methods, we first need to understand what the OS module is. The OS module in Python helps you work with your computer’s operating system. It lets you do things like accessing files and folders, managing processes, and executing commands.
You can use it to get information about directories, create or delete folders, and perform other system tasks. Basically, it makes it easier to handle system-related stuff in your Python programs.
15 Highly Used Methods of OS Module
Now that we know about the OS module, let’s see what methods it has to offer and for what they are used for.
1. os.getcwd()
This method returns the current working directory as a string. The working directory is the directory from which your Python script is currently running.
Example:
import os
current_dir = os.getcwd()
print("Current directory is:", current_dir)
Output:

2. os.chdir(path)
This method changes the current working directory to the specified path.
Example:
import os
os.chdir('C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Intro Streamline')
print(f"Current working directory changed to: {os.getcwd()}")
Output:

3. os.listdir(path)
This method returns a list containing the names of the entries in the directory given by path. If no path is specified, it defaults to the current directory.
Example:
import os
files_in_current_dir = os.listdir()
print("Files in current directory:", files_in_current_dir)
Output:
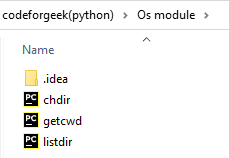

4. os.mkdir(path)
This method helps us to create a directory named path.
Example:
import os
path = 'C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\New directory'
os.mkdir(path)
print(f"Directory '{os.path.basename(path)}' created at: {os.path.abspath(path)}")
Output:

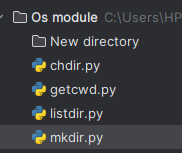
5. os.path.splitext(path)
This method splits the given path into its base filename and file extension, returning a tuple with two elements.
Example:
import os
path = 'C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\chdir.py'
root, ext = os.path.splitext(path)
print("Root:", root)
print("Extension:", ext)
Output:

6. os.rmdir(path)
This method removes the directory path. However, it can only delete directories that are empty. If the directory specified by the path contains any files or other directories, os.rmdir() will raise an error.
Example:
import os
path = 'C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\New directory'
os.rmdir(path)
print(f"Directory '{os.path.basename(path)}' deleted at: {os.path.abspath(path)}")
Output:

7. os.path.getsize(path)
This method returns the size of the file specified by path in bytes.
Example:
import os
size = os.path.getsize('C:/Users/HP/Desktop/codeforgeek(python)/Os module/chdir.py')
print("size of the file in bytes is :", size)
Output:

8. os.path.exists(path)
This method checks whether a file or directory exists at the specified path. If it exists, it returns True, otherwise, it returns False
Example:
import os
exists = os.path.exists('C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\mkdir.py')
print("Exists:", exists)
Output:

9. os.path.isfile(path)
This method returns True if path is an existing regular file.
Example:
import os
is_file = os.path.isfile('C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\mkdir.py')
print("Is file:", is_file)
Output:

10. os.path.isdir(path)
This method checks if the given path corresponds to a directory. It returns True if it’s a directory, otherwise False.
Example 1:
import os
is_dir = os.path.isdir('C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Streamlit')
print("Is directory:", is_dir)
Output:

Example 2:
import os
is_dir = os.path.isdir('C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module')
print("Is directory:", is_dir)
Output:

11. os.path.join(path1, path2, …)
This method combines multiple paths together to form a single path. It joins the paths using the appropriate separator for the operating system, such as
Example:
import os
path = os.path.join('C:\\Users\\HP\\Desktop\\codeforgeek(python)', 'Os module', 'mkdir.py')
print("Joined path:", path)
Output:

12. os.path.abspath(path)
This method returns the absolute version of the path. An absolute path gives the complete location of a file or directory from the root of the file system.
Example:
import os
absolute_path = os.path.abspath('chdir.py')
print("Absolute path:", absolute_path)
Output:

13. os.path.basename(path)
This method returns the base name of the file or directory specified by path, stripping any leading directory components.
Example:
import os
filename = os.path.basename(' C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\chdir.py')
print("Filename is :", filename)
Output:

14. os.path.dirname(path)
This method returns the directory component of the given path, excluding the last element.
Example:
import os
directory = os.path.dirname('C:\\Users\\HP\\Desktop\\codeforgeek(python)\\Os module\\chdir.py')
print("Directory is :", directory)
Output:

15. os.path.split(path)
This method separates the directory path and the filename from the given path, returning them as two strings in a tuple.
Example:
import os
head, tail = os.path.split(' C:/Users/HP/Desktop/codeforgeek(python)/Os module/chdir.py')
print("Head of the path is :", head)
print("Tail of the path is :", tail)
Output:

Summary
And we have reached the end of this article, I hope you now have a clear understanding of the os module in Python. It makes it easy for us to work with paths and directories, helping us to add, delete, check, split, and perform many such operations on directories with ease. Now that you know the 15 most commonly used os methods in Python, you can use them easily in the future.
If you like this one, do check out more of our Python articles –
- Calculating the Mean of Pandas DataFrame in Python
- Python PDFKit Module: Convert HTML, URL, and Text to PDFs
Reference
https://docs.python.org/3/library/os.html