Python’s NumPy Library has always been a boon to developers. One of its very useful tools is the cumsum method, which helps us find the cumulative sum of a number. Don’t know what a cumulative sum is? Want to know why we need to calculate cumulative sums? Don’t worry, you will find all the answers to these questions in this article, where we will also see examples of how we can use the cumsum method of NumPy. So let’s start.
What Are Cumulative Sums?
Cumulative sums are a way of adding up numbers in a sequence as you go along. Imagine you have a list of numbers like [1, 2, 3, 4]. A cumulative sum of these numbers would look like this: 1, 1 + 2 = 3, 1 + 2 + 3 = 6, 1 + 2 + 3 + 4 = 10
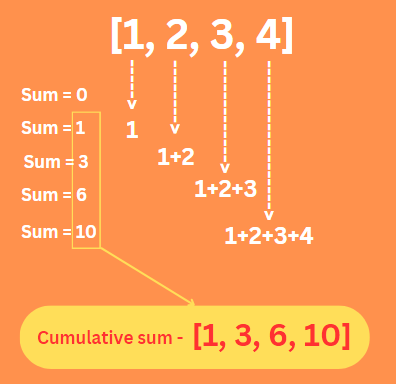
So, the cumulative sum at each step adds the current number to the sum of all the numbers that came before it. It’s like keeping a running total as you move through the list.
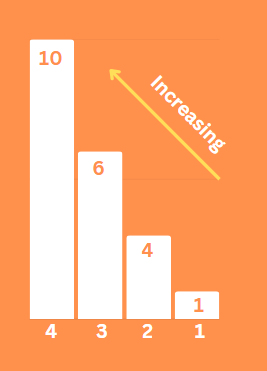
In simpler terms, if you have a list of numbers, the cumulative sum tells you the running total of those numbers at each step. It’s useful for tracking how something changes over time or for understanding the overall trend of a set of values.
Some uses of cumulative sums are listed before:
- Cumulative sums show how money grows or decreases over time in investments, savings, or expenses.
- This helps us see how things like weather, sales, or test scores change over time by adding up the data little by little.
- It helps figure out how the volume of sound changes in recordings over time.
- It also helps businesses track their stock by adding up what they get and sell over time.
Introducing NumPy.cumsum()
NumPy’s cumsum function adds up numbers in an array one by one, showing the total at each step. The syntax of this function is quite simple.
Syntax:
numpy.cumsum(a, axis=None, dtype=None, out=None)
Now, let’s understand what each parameter means.
- a: This is the input array. It could be a list or a NumPy array containing the numbers you want to calculate the cumulative sum for.
- axis: You can decide which way to add the numbers with this optional parameter. If you don’t choose, it adds them all together in a line.
- dtype: You can choose what type of numbers you want the result to be with this optional parameter. If you don’t choose, it figures it out based on what you gave it.
- out: This parameter allows you to specify an alternate output array in which to place the result. It’s optional.
Finding Cumulative Sums Using NumPy.cumsum()
Now that we know about the concept of cumulative sums and its Python method, let’s experiment with it in some code.
Example 1:
If we provide a 1-dimensional array, cumsum will calculate the cumulative sum along the specified axis (or flattened by default). For example, if we have [1, 2, 3, 4], it will return [1, 3, 6, 10].
import numpy as np
numbers = input("Enter a list of numbers separated by spaces: ")
numbers_list = list(map(int, numbers.split()))
numbers_array = np.array(numbers_list)
cum_sum = np.cumsum(numbers_array)
print("The Original array:", numbers_array)
print("The Cumulative sum of the given data:", cum_sum)
Output:
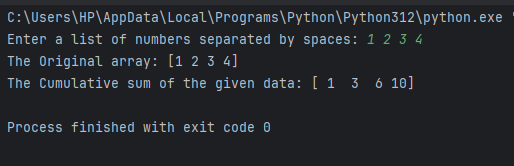
Example 2:
If we provide a 2-dimensional array, we can specify the axis along which the cumulative sum is computed. If we have [[1, 2], [3, 4]], and we choose axis=0, it will return [[1, 2], [4, 6]] because it calculates the cumulative sum along the rows. If we choose axis=1, it will return [[1, 3], [3, 7]], as it calculates along the columns.
import numpy as np
array_2d = np.array([[1, 2],
[3, 4]])
sum_rows = np.cumsum(array_2d, axis=0)
sum_columns = np.cumsum(array_2d, axis=1)
print("Original 2D array:")
print(array_2d)
print("\nCumulative sum along rows (axis=0):")
print(sum_rows)
print("\nCumulative sum along columns (axis=1):")
print(sum_columns)
Output:
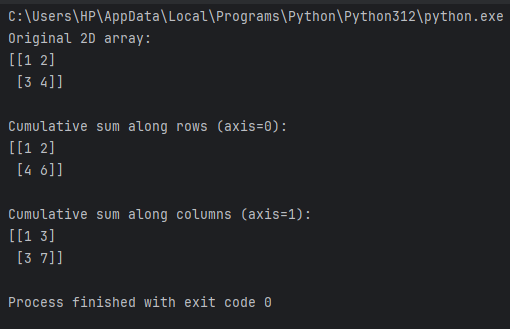
Example 3:
In this example, we use the dtype parameter to specify the data type of the output array as float, and the out parameter to store the cumulative sum result in a pre-allocated array named result.
import numpy as np
numbers = np.array([1, 2, 3, 4, 5])
result = np.zeros_like(numbers, dtype=float)
np.cumsum(numbers, out=result)
print("Original array:", numbers)
print("Cumulative sum with dtype=float and out parameter:", result)
Output:
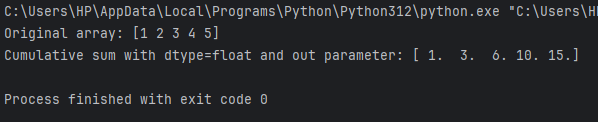
Summary
So we have reached the bottom line. I hope that you have found answers to all your questions about cumulative sums and the cumsum library in Python. Here, we have covered in detail the definition of cumulative sums, the need to calculate them, and how we can calculate them. We have also seen code implementations, which enhance the learning even more. This function will surely help you while you work with graphs and data in the future.
Continue Reading:
- Calculating the Mean of Pandas DataFrame in Python
- Create a Pandas DataFrame from Lists: 5 Easy Approaches
Reference
https://numpy.org/doc/stable/reference/generated/numpy.cumsum.html