Creating a Pandas DataFrame using lists in Python is one of the most common and straightforward ways to construct a DataFrame.
Whenever we have data in the form of lists, we can arrange and refine it by creating a DataFrame. We can also apply filters, transforms, or aggregation operations on lists to create a structured DataFrame that can be easily analyzed. Often, data is extracted as lists from various sources like APIs, databases, or CSV files and then imported into Python. We can convert these imported data into a DataFrame for further exploration and manipulation.
In this article, we’ll look at 5 ways to create a pandas DataFrame from lists so that you can use the option that best suits you. Let’s get started.
Creating a Pandas DataFrame from List in Python
There are five ways of creating DataFrame from lists which are as follows:
- Creating a Pandas DataFrame Using a Simple List
- Creating a Pandas DataFrame Using List with Index and Column Names
- Creating a Pandas DataFrame Using the zip( ) Function
- Creating a Pandas DataFrame Using Multi-Dimensional List
- Creating a Pandas DataFrame Using Dictionary of Lists
Let’s see them one by one with an example for each.
1. Creating a Pandas DataFrame Using a Simple List
Let’s look at the first topic which is using a simple list, in this, we will just take a list and try to create a DataFrame from it.
Example:
import pandas as pd
lst = [10,30,56,78,90]
df = pd.DataFrame(lst)
df
Here we have first imported the pandas as pd. Then created a list lst contains a few numbers. For creating a DataFrame, we have used the pd.DataFrame() function and passed the list lst as an argument, we have defined a variable df which will store the DataFrame and can be directly called to print it.
Output:
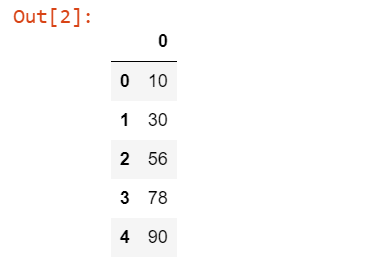
We can see that in the DataFrame, the numbers 10.30,56,78,90 which are our list elements have taken the form of rows. So we can say that if we create a DataFrame using a list then those list items will take the form of a single column.
2. Creating a Pandas DataFrame Using List with Index and Column Names
Let’s now try to give me a unique index for the DataFrame instead of a random number that is automatically generated in the previous example, plus we’ll change the name of the column from 0 to something more relevant.
Example:
import pandas as pd
lst = [10,30,56,78,90]
df = pd.DataFrame(lst,index =['a','b','c','d','e'], columns=['Marks'])
df
Here, inside the pd.DataFrame() function we have passed a list and index as arguments. We also wanted to change the name of the column, for that we have passed an additional argument columns=[‘Marks’].
Output:
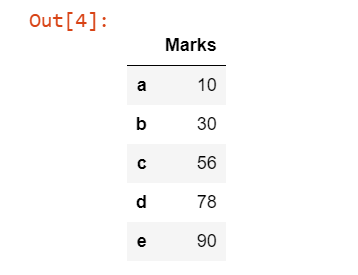
After printing df we can see that the column name is changed and indexes are also changed.
3. Creating a Pandas DataFrame Using zip() Function
In this example, we’ll use the zip() function with pd.DataFrame() function to create a DataFrame. For the zip() function, we need two or more lists so we can join them together to form a DataFrame.
Example:
import pandas as pd
lst1= ['Raj', 'Rajat', 'Aasif', 'Karan']
lst2=[56,89,90,34]
df = pd.DataFrame(list(zip(lst1,lst2)))
df
Here we have created the first list with the name lst1 which stored the names of students, and in the second list which is lst2 we have marks. Now we have used the zip() function and provided list1 and list 2 then passed it into the list() function which will create a list of tuples that are mapped according to their indexes. Now we wanted to create a DataFrame, so we passed all of this into pd.DataFrame() and printed the DataFrame.
Output:
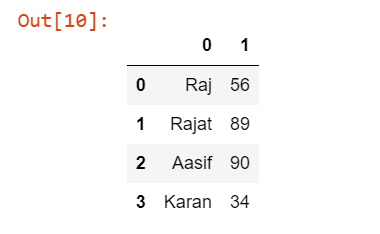
4. Creating a Pandas DataFrame Using Multi-Dimensional List
In this example, we will create some lists inside a list and then convert that multidimensional list to a DataFrame.
Example:
import pandas as pd
lst = [['a',2],
['b',6],
['ç',10],
['d',90]]
df=pd.DataFrame(lst, columns=['Alphabets','Integers'])
df
Here we have created a list of lists and named it lst, then we have created a DataFrame df using pd.DataFrame() while providing the list lst as an argument. We have also provided the column names.
Output:
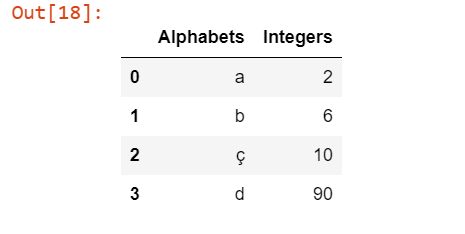
Seeing the output we can say that the first element of every list will form the first column which is Alphabets, and the second element of every list will form the second column which is Integers.
5. Creating a Pandas DataFrame Using Dictionary of Lists
In this example, we’ll create a dictionary and then try to convert it to a DataFrame.
Example:
import pandas as pd
dictionary = {'Name':['Raj','Rajat','Karan'],
'Marks': [50,90,80]}
df = pd.DataFrame(dictionary)
df
Here, we have created a dictionary. The key of the dictionary will act as a column name and the values will act as the cells. Then passed it as an argument to pd.DataFrame() function and printed the DataFrame.
Output:
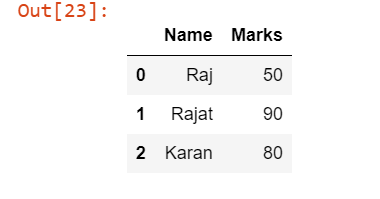
In the above output, we can see that the Name and Marks which were the keys of the dictionary become columns with corresponding values.
Summary
Creating Pandas DataFrame using lists is a fundamental and versatile method for handling data in tabular format. It allows us to work efficiently with structured data, making it an essential technique in data cleaning, preprocessing and data importing from external sources. We have discussed five ways of creating a DataFrame from lists with examples. After reading this tutorial, we hope you can easily create a pandas DataFrame using lists in Python.
Also Read: Convert Pandas DataFrame To NumPy Array
Reference
https://stackoverflow.com/questions/43175382/python-create-a-pandas-data-frame-from-a-list