Modules are the most important part of developing a Node.js application. Many beginners think of modules as they are external packages that can be installed using package managers like NPM to get some functionality but that’s not it. The modules we usually talk about are called third-party modules, besides them, there are other types of modules as well.
Even Node.js lets us create our own modules to make our coding life easy by organizing complex functionality in single or multiple files of JavaScript that can be reused. These are called local modules that consist of code that performs specific activities and can be used later so that we do not need to code that functionality every time.
Let’s dive deeper into the concept of modules, discover their types and understand how to use them properly to increase development output and skills in Node.js.
Also Read: What is Node.js?
Types of Modules in Node.js
We can divide Node modules into three types based on how they are offered or how they interact with the application.
- Core Modules
- Local Modules
- Third-Party Modules
Let’s look at each of them one by one.
Node.js Core Modules
Node.js Core Modules are the modules pre-built with Node.js. These are pre-written in the Node.js system and whenever you install Node.js they will be enclosed as part of the installation.
Some of the most popular core modules are:
- HTTP: Used for making all types of HTTP requests such as GET, POST, PATCH, DELETE, etc
- FS: A File System Module is used for working with files present in the operating system.
- Path: It can be used to work with file paths.
- Child Process: If you need to code on the operating system’s process level, you can use this module.
Using Node.js Built-in Modules
To use the core module it is not necessary to install it, you just need to import it. You can simply use the require() function and pass in that module’s name. The require() function will return the module and save it in a variable.
const path = require('path')
Example:
const path = require('path')
const name = 'Sushant'
const output = path.join('/', 'Users', name, 'test.txt') //'/Users/Sushant/test.txt'
console.log(output)
Here we have imported the “path” module, we have not installed it because it is one of the Node.js core modules. Then we used its method join() to combine multiple path segments into one and console log the output.
Output:

Node.js Local Modules
Sometimes developer needs the same functionality in different places in their software so they create their own local modules. Local Modules are those modules that are created by the developer and are not pre-built in Node.js. They are used to make development life more effortless and fast because they automatically decrease code length. Let’s create our own local module.
Creating and Using Local Modules in Node.js
To use a local module, we first have to create it. Below is an example of creating and using a local module.
Example:
Let’s create a local module “greetingsModule.js” and then try to import and use it inside the main server file.
// greetingsModule.js
// Simple Greetings Module
exports.sayGoodMorning = function(name){
console.log(`Good Morning ${name}!`)
}
exports.sayGoodAfternoon = function(name){
console.log(`Good Afternoon ${name}`)
}
exports.sayGoodNight = function(name){
console.log(`Good Night ${name}`)
}
In the above code, we have created three functions and exported them using the exports keyword. This keyword will export these functions so that they can be imported to use in other files as modules.
const greetingsModule = require('./greetingsModule')
greetingsModule.sayGoodMorning('Sushant')
greetingsModule.sayGoodAfternoon('Sushant')
greetingsModule.sayGoodNight('Sushant')
Here, we have imported the file created in the above step using the require() method. Then used the dot operator and called all three functions. The output of all three functions can be shown below.
Output:
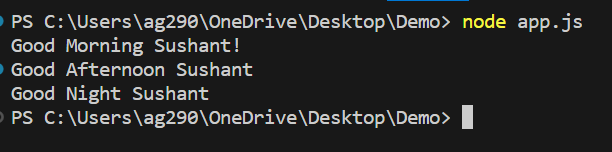
Node.js Third-Party Modules
Most of the time coding requires several kinds of functionality. Now to do this we have two methods. The first method is we can implement complete functionality on our own and the second method is we can use any third-party module which will provide us with the same functionality. We usually go for the second option. The 3rd party modules are libraries that are publicly available and developed by someone else. These are found on repositories such as npm.
Some of the most popular third-party modules are:
Installing and Using Third-Party Modules with NPM
To use third-party NodeJS modules, it is necessary to install them first. To install any third-party module we need a package manager. The most popular package manager is npm. When you install Node.js in your system, npm is also automatically installed.
Below is the command to install any package from npm directly into your project.
npm install express
The above command will install a module “express” in your project.
Also, we can install the module globally so that it can be accessed by any of your projects, you can use the same command with an additional attribute “-g” to install a module globally.
npm install -g express
Here -g means global installation.
After installing a third-party module locally or globally, you can import it into your project using require(), just like we imported the core module or a local module.
const express = require('express')
Example:
Let’s use the express module and create a simple Node.js application that sends an HTML file for the home page containing an h1 tag with the message “Home”.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
app.listen(3000);
Here we have imported the “express” module that we already installed. Then we used its “sendFile()” method to load and send an HTML file to the client when the request was received for the home(“/”) route.
Output:
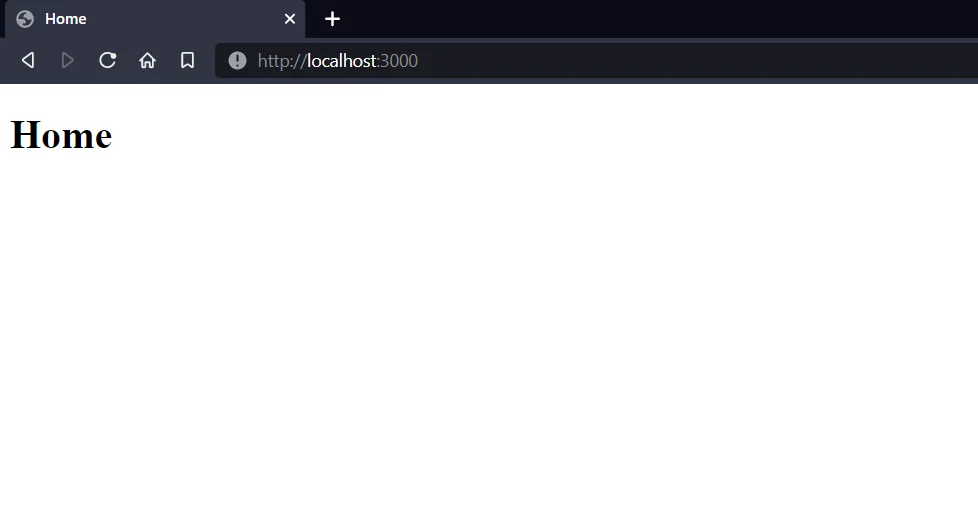
Read More: How to send an HTML File in Node.js?
Summary
Modules are an essential part of a developer’s life as they are very powerful and make things easy. There are three types of modules in Node JS, the core module which is a built-in module that comes with Node itself, a local module that can be created manually and a third-party module. These are very helpful if you want to save time. After reading this post, you are aware of modules, their types, use cases, and how you can install third-party modules. Thank you for reading this article, we hope it was helpful for you.
Reference
https://nodejs.org/api/modules.html