Web Module is used to create a web application in Node.js. A web application in Node.js is a server containing various resources that clients can request, these resources include any type of video/audio files, document files even HTML, CSS, and JS files that combined to form a web page.
Using the web module we can create servers in order to send the web page on different routing requests such as sending the home page when the client requests the “/” route or sending a contact form when the client requests the “/contact” route.
We can also fetch data from clients using web modules such as fetching login credentials i.e username and password. Then we can define logic for the validation where if it’s a valid user, we send the resource they request, if not then simply send an unauthorized message.
Working With the NodeJS Web Module
The HTTP module in NodeJS is used to create a web server that can handle various HTTP requests sent by the HTTP client like web browsers and return web pages in response. The HTTP module can start a server locally on a given port which helps in accessing the web application.
HTTP is a built-in module so it is not required to install it. We can simply import it using the below syntax.
Syntax:
const http = require('http');
Creating Server using the NodeJS web module
We can create servers using one of the method createServer() of the HTTP module which can start a server on a given port, takes request from the client side, and send a response.
Syntax:
http.createServer(callback)
In the callback, we can define the functionality of our web application. We have covered the NodeJS Callbacks in a separate article if you want to learn about them.
Create a web server using the HTTP module
Let’s create a very simple Node.js Web Application using an HTTP module, we have already covered an article in which we define how to create a web application using the Express Module.
Create a folder, open it inside any code editor and create a file name app.js.
Inside the app.js file, import the HTTP module.
const http = require('http');
Then use createServer() of the HTTP module and pass an arrow function as a callback containing two arguments request and response. The response is used to send data to the client and the request is used to fetch data from the client. The client in our case is a web browser.
http.createServer((request, response) => {
})
We have covered the NodeJS Arrow functions in another article if you want to learn about them.
Inside the callback use response.send() method to send a message to the client, let’s type “Hello world!” and then end the response to send the message.
http.createServer((request, response) => {
response.write('Hello World!');
response.end();
})
Then at the end of the createServer() method use dot notation and call the listen method and pass a port 3000 so that we can able to run our application on the defined port.
http.createServer((request, response) => {
response.write('Hello World!');
response.end();
}).listen(3000);
Then open the terminal and type the following command to run the application
node filename
where the filename is the name of the file you are writing the code, in our case the filename is app.js.
Then open the browser and type the following URL to see the output of the application.
http://localhost:3000/
Complete Code:
const http = require('http');
http.createServer((request, response) => {
response.write('Hello World!');
response.end();
}).listen(3000);
Output:
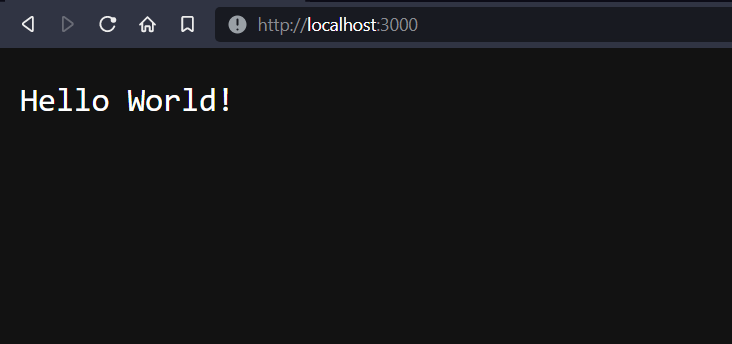
Summary
Node.js is used in creating different types of projects such as APIs, microservice, etc but creating a web application requires a web module. We hope this tutorial helps you to understand the concept of web modules in Node.js.
Reference
https://nodejs.org/api/http.html