While building web applications we may face various types of errors that can be caused due to some reasons. The kinds of errors that we may come across in Node.js are Syntax errors, Run time errors, Module errors, Network errors, I/O errors, Asynchronous errors, Environment and Configuration Errors, and Memory and Performance Issues. To run our application without any errors we should follow some error handling techniques for the particular type of error that occurred.
In this article, we will focus on solving a Module error “Cannot find module” by observing some basic mistakes that we often make accidentally while coding. But first, let’s get started with some context.
Overview of Node.js & NPM
Node.js is an open-source, cross-platform, server-side JavaScript runtime environment that allows users to run JavaScript code outside of a web browser. It is built on the V8 JavaScript engine, which is the same engine used by Google Chrome. It is used to build many web applications and it provides many inbuilt modules.
Besides Node.js inbuilt modules we can also download external frameworks and libraries to make our applications more efficient. One of the ways to download these third-party libraries is using NPM. Which is a package manager for Node.js having millions of packages to be used in your Node.js project. To use the NPM modules, you first have to install them and if it is not successfully installed this causes the “Cannot find module” errors, let see this in depth.
Read More – What is Node.js?
“Cannot find module” Error in Node.js
The “Cannot find module” mainly throws when the module we are trying to import or require cannot be found or accessed by Node.js. So generally, we are advised to install the module in the directory before importing it into our application. The reasons for this error might be the Module not being installed, Incorrect module path, Missing or incorrect file extension, Incorrect module name or case sensitivity, or the Module not located in the correct directory.
One of the examples is here, in the below script we tried to import the module, but the module name is case-sensitive so the error occurs.
const http = require('htTp');
const server = http.createServer((req,res)=>{
res.end("Hello World");
})
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
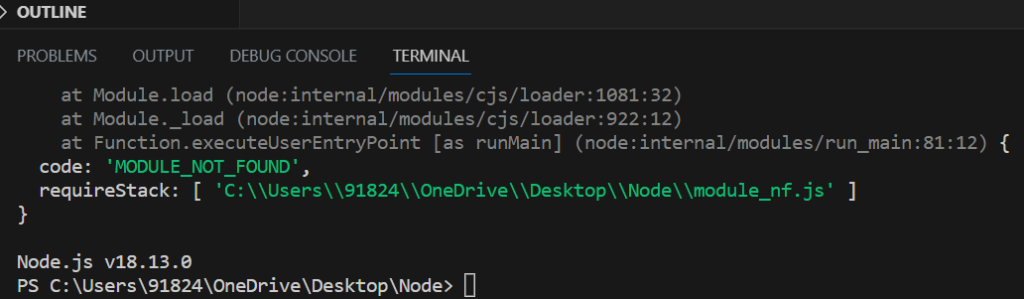
Troubleshooting “Cannot find module” Error
Let’s now look at the causes of the occurrence of this error and ways to fix it.
1. Module not installed
We face this error if the module that we are trying to access may not be installed in your project’s dependencies. To fix this error we need to install the module using Node Package Manager.
For this go to the terminal, move to your project directory and run the following command:
npm install <module_name>
This will download and install the module in your project, and you should be able to import it without the “Cannot find module” error.
2. Missing or incorrect file extension
Ensure that you specify the correct file extension for the module you are importing or trying to access. Node.js requires you to include the file extension (e.g., .js, .json) while importing the modules. If the file extension is missing or incorrect, Node.js won’t be able to locate the module that you are trying to access.
3. Incorrect module name
Ensure that you are accessing the module with the correct name. If the module name is misspelt or doesn’t match the actual module name, Node.js won’t be able to find it. Additionally, Node.js is case-sensitive, so make sure the case of the module name matches the actual file. The example that we have discussed above comes under this case.
4. Module not located in the current directory
If the module you are trying to access is not located in the current directory or the node_modules directory, Node.js won’t be able to find it or locate it. Make sure the module is located in a directory that Node.js can access, or install it as a dependency as mentioned in point 1 using the given command.
5. Incorrect module path
If you want to specify a relative path while importing a module, make sure the path is correct. Check twice that the path you are providing matches the actual file location. If the module is located in a different directory, you may need to adjust the path accordingly or install the module in the current directory.
Frequently Asked Questions (FAQs)
What is npm install?
The npm install is used to install modules from the npm registry. It installs modules specified in the package.json file and downloads them in the node_modules folder of the current directory.
What does cannot find module mean?
Cannot find module means that the module you are trying to access is not located or installed in your project’s dependencies.
How to fix module not found in JavaScript?
One of the most common ways to fix this error is to install the missing module again. For installing the module move to your project directory and run the command npm install <module_name>.
How do you check module is installed or not?
You can check if a module is installed or not by using two methods, first by looking at the node_modules directory in the project folder for the module, and second by running npm list <module_name>.
How do I fix no module found error in Python?
The solution for this error in Python is quite similar to NodeJS, you just have to use a package manager like pip to install the missing module. To install the module using pip, open the terminal and run the command pip install <module_name> globally or locally.
Continue Reading –
- React & TypeScript: What Is ReactNode and When to Use It?
- Set Up an Nginx Reverse Proxy on Linux with Node.js Application
Conclusion
By addressing these common causes, you should be able to resolve the “Cannot find module” error and successfully import the required modules into your Node.js application. Remember to consult the specific error message, stack trace, and additional information to aid in troubleshooting and debugging the issue effectively.
Reference
https://stackoverflow.com/questions/9023672/how-do-i-resolve-cannot-find-module-error-using-node-js