JWT stands for JSON Web Token, it is used to contain data that is compact in size and provides a way to transmit that data easily between the client and server. The JWT can contain highly confidential data since it is digitally signed using a public/private key pair, making it hard to crack. The JWT can transmit our internet via URL, HTTP header, or POST parameter. The data JWT contains is user credentials that are used to authenticate a user.
When a user login into the system, the system creates a JWT that send back to the user, and then each time the user request any resource, the JWT comes with that request used to verify whether the user who requested the resources is a valid user or not.
Node.JS JWT Structure
A JWT is a string that contains three distinct parts
- Header: It contains the algorithm used for JWT encryption.
- Payload: Payload is any data that includes in JWT, this data can be a user credential.
- Signature: It is an encrypted string generated using the header, payload with a secret key.
Node.JS JWT Module
The jsonwebtoken is a module that provides methods to create and verify the JSON Web Token. It is not a built-in module, it is required to install it before using it.
Syntax:
npm i jsonwebtoken
sign()
This method is used to create the JSON Web Token from the given data and the key pass as an argument to this method. This method generates a header by default.
Syntax:
sign(data, secrete_key, options, callback)
- data: data is used to generate the payload
- secrete_key: secrete_key is a key used to generate the signature, and is required to decode the token in order to get the data back
- options: options can be different values like expiresIn, subjects, noTimestamp, etc.
- callback: Inside the callback, we can get the error or the generated token. The callback when passes make the method asynchronous.
Node.JS verify()
This method is used to verify the JWT created by the sign() method. In order to get the data that JWT contains it is required to use the same secrete_key used to generate the token.
Syntax:
verify(token, secrete_key, options, callback)
- token: token must be a valid JWT
- secrete_key: secrete_key is the key used for creating the given token
- options: options can be different values like issuer, subject, etc
- callback: Inside the callback, we can get the error or the decoded data.
JWT Authentication API
JWT Authentication API is an API by which the JSON Web Token is generated and verified.
Let’s create the JWT Authentication API using the express and JWT modules in Node.js.
Create a folder containing a file “app.js” & open it inside the Code Editor.
Then in the terminal, type the following commands
npm init -y
this will initialize the Node Packages manager, by which we can install any Node.js framework.
npm i express jsonwebtoken
this will install express and jsonwebtoken as a module in your application.
Inside the “app.js” file, import the express and jsonwebtoken modules.
const express = require('express');
const jwt = require('jsonwebtoken');
Create a constant “app” and set it to “express()”, which means creating an instance of an express module, by which any of the express functions can be called. Then use the express middleware to parse JSON data in order to fetch data from the client.
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
Use the post method of express to handle the post request for the ‘generateJWT’ route, where we fetch the data that comes from the client and store it in the user object.
app.post("/generateJWT", (req, res) => {
const username = req.body.username;
const password = req.body.password;
if (!(username && password)) {
res.status(400).send("All input is required");
}
const user = {
username: username,
password: password,
};
});
Then use the sign() method which we explain earlier. Inside this method pass the user’s object which contains the username and password in order to generate the payloads and pass a secrete_key. Then pass an option expiresIn which is used to set the time after which the session will expire and then finally a callback function. Then in the callback, we first check for the error, if found then send it with a status code 401 otherwise send the generated JWT with a status code 200.
app.post("/generateJWT", (req, res) => {
const username = req.body.username;
const password = req.body.password;
if (!(username && password)) {
res.status(400).send("All input is required");
}
const user = {
username: username,
password: password,
};
jwt.sign({ user }, "codeforgeek", { expiresIn: "2h" }, (err, token) => {
if (err) {
res.status(401).send(err);
} else {
res.status(201).json(token);
}
})
});
Use the post method of express to handle the post request for the ‘validateJWT’ route, where we fetch the token. If the token is not fetched then send an error message with the status code 403.
app.post("/validateJWT", (req, res) => {
const token = req.body.token;
if (!token) {
res.status(403).send("A token is required for authentication");
} else {
}
});
Then use the verify() method which we explain earlier. Inside this method pass the token, secrete_key, and the callback as an argument. Then in the callback, we first check for the error, if found then send it with a status code 401 otherwise send the decoded data with a status code 200.
app.post("/validateJWT", (req, res) => {
const token = req.body.token;
if (!token) {
res.status(403).send("A token is required for authentication");
} else {
jwt.verify(token, "codeforgeek", function (err, decoded) {
if (err) {
res.status(401).send("Invalid Token");
} else {
res.status(200).send(decoded);
}
});
}
});
Create a port using listen() method to test the API, and pass the 3000 port as an argument.
app.listen(3000);
Now, inside the postman search bar and type the below URL in order to test the API.
http://localhost:3000/
Output:
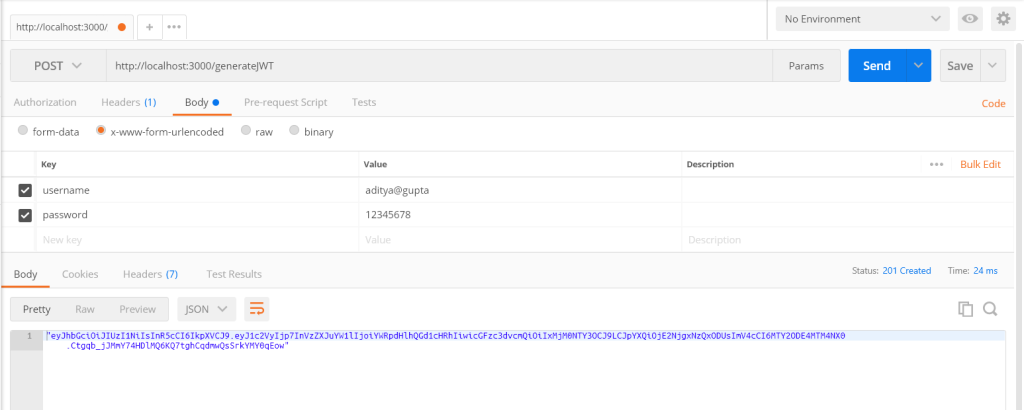
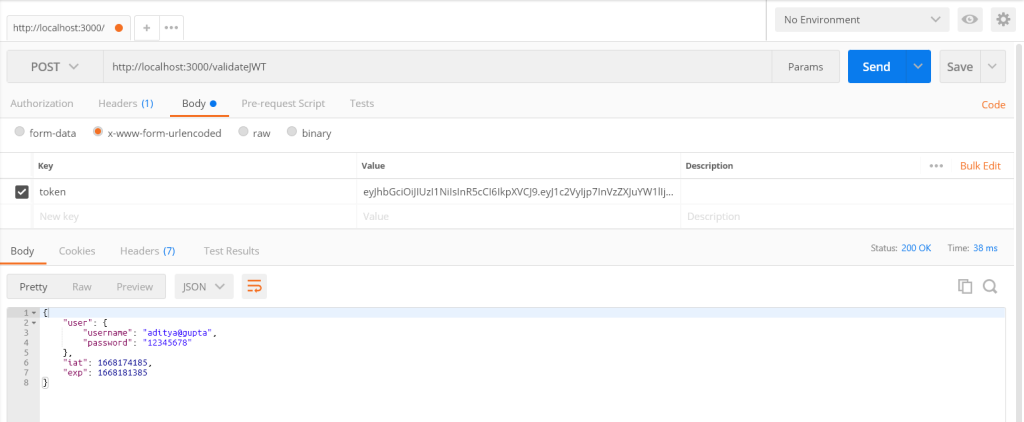
Summary
The JWT Authentication API is used to generate the JSON Web Token and to verify it. We can use jsonwebtoken module for creating this API which contains the sign() and the verify() methods that can generate the JWT and verify it respectively. We hope this tutorial helps you to create the JWT Authentication API in Node.js.
Reference
https://www.npmjs.com/package/jsonwebtoken