NodeJs Child Process is used to create child processes that can run simultaneously proving a multicore functionality to a Node.js application.
Node.js is a single-threaded, it can perform one operation at a time. This limits the scalability of a Node.js Application since we are not able to utilize the full power of the CPU. For running multiple operations at a time parallelly, Node.js Provide a module Child Process which contains many methods used to initiate processes that can run individually without affecting other processes. The child processes then terminate once complete their execution.
To understand the Child Process it is important to have prior knowledge of Events and Streams in Node.js. We have covered Node.js Events and Node.js Streams in another article if you want to learn about them.
NodeJS Child Process Module
For creating child processes, it is required to import the child process module using the below statement.
Syntax:
const childProcess = require('child_process');
Methods to Create Child Process
Let’s now look at some of the methods that can be used to create child processes in NodeJs.
1. spawn()
This method takes commands, arguments, and options to start a new process. The options can contain shell as a boolean value, and “true” runs the command inside the shell. By default, the value is false which will not execute the command on the shell, so it is important to pass the shell as true.
Syntax:
spawn(command[,arguments][,options])
Example:
Here we are using the spawn method to create a child process that will execute the command pass as an argument. The result of this method is equivalent to the result that comes when passing the command mkdir new-folder in the shell.
const { spawn } = require('child_process');
const child = spawn('mkdir', ['new-folder'], {shell: true});
child.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
child.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
child.on('close', () => {
console.log('child process closed');
});
Output:
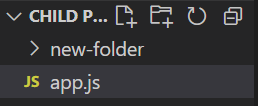
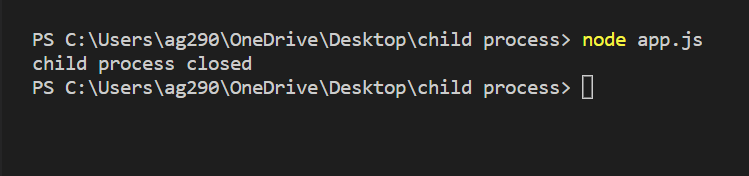
2. exec()
This method takes a command, options, and a callback function in which we can write the code to log the error or success message.
Syntax:
exec(command[, options][, callback])
Example:
Here we are executing the same command as the previous example by using the exec() method. We are passing the command and argument together instead of passing them separately like in the case of the spawn method.
const { exec } = require('child_process');
exec('mkdir new-folder', (err) => {
if (err) {
console.error(err);
return;
}
console.log("New folder Created");
});
Output:
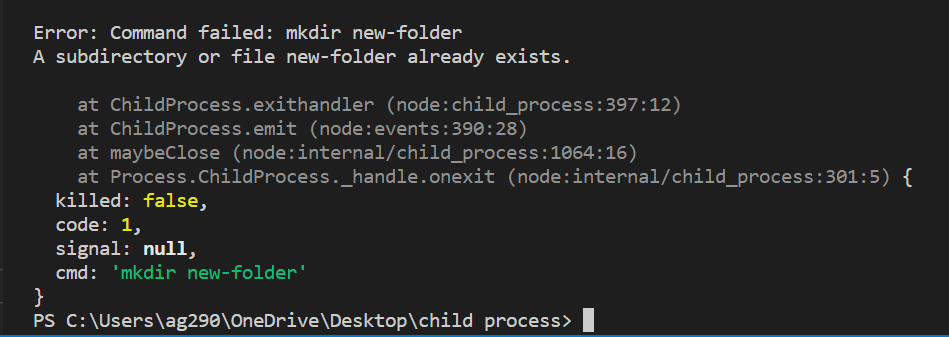
3. fork()
This method is similar to spawn but it provides a way of communication between the parent and child process via the IPC communication channel by using the send method.
Syntax:
fork(modulePath[, args][, options])
Example:
Here we have established a channel between the parent file parent.js and the child file child.js by using the fork module. Then send a message from child.js to parent.js to see that they are able to communicate, once received the message at both ends, we close the child process because it is no longer needed.
parent.js
const { fork } = require('child_process');
const child = fork(__dirname + '/child.js');
child.on('message', function(message) {
console.log("Message from child: " + message.msg);
});
child.send({ msg: 'This is parent process' });
child.js
process.on('message', function(message) {
console.log("Message from parent: " + message.msg);
});
process.send({ msg: 'This is child process' });
Output:

Summary
Child Process is helpful for running various processes simultaneously, this helps in utilizing multitasking functionality in Node.js since by default Node.js runs every process on a single thread. This is very useful when creating a large-scale Node.js Application running many tasks at a time, without blocking the other part of the code.
Child processes run independently from each other so does not interrupt other processes. There are various methods to start a child process providing different implementations and features. Hope this article helps you to understand the concept of the child process in Node.js.
Reference
https://nodejs.org/api/child_process.html#child-process