Node.js is used to create projects such as APIs, microservices, servers, and even web applications. In a web application, we have to send the HTML file so that the user can interact with the application. We can send different HTML files on different routing requests such as sending a file when the user requests the home route “/”, or sending a contact form when the user requests the “/contact” route.
There are many modules that can be used for sending HTML files, like HTTP, etc. But we will use the express module which is beginner friendly and we already covered an article on NodeJS Installation, Setup, and Creating a Hello World Application in NodeJS where we use express to send a message as a response to the client.
Express Module
Express module has a method sendFile() which can be used to send HTML files directly from the Node.js server to the browser.
For using the express module it is required to install it using NPM.
Syntax:
npm i express
For more about NPM check: A Beginner’s Guide to Node Package Manager
Create an application to send an HTML file using Node.js
Functionality: A simple Node.js Web App that sends an HTML file for the home page containing an h1 tag with the message “Home” in response to the client.
Approach: We use the “sendFile()” method of Express to send an HTML file to the client when the request receive for the home(“/”) route.
Implementation: Below is the step-by-step implementation for sending an HTML file in Node.js.
Step 1: Create a new folder & open it on code Editor.
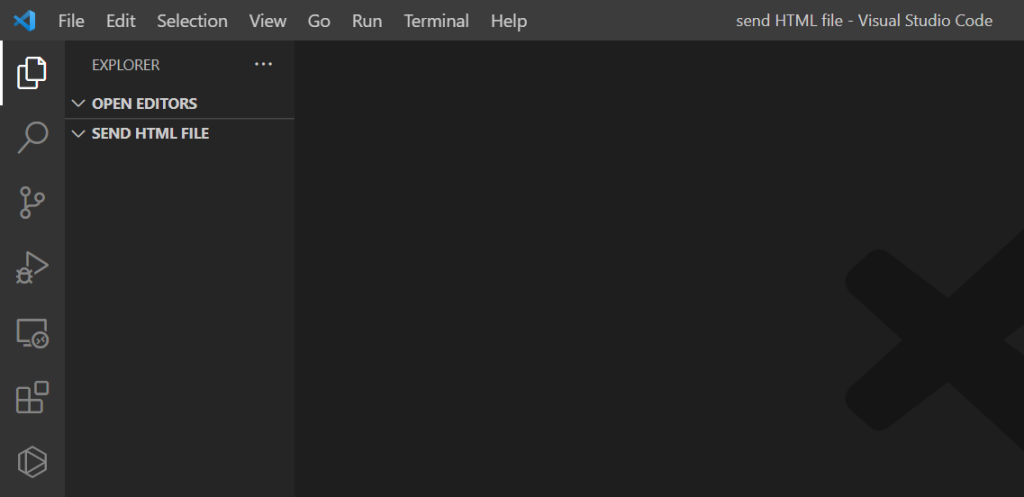
Step 2: Create a file “aap.js” inside that folder.
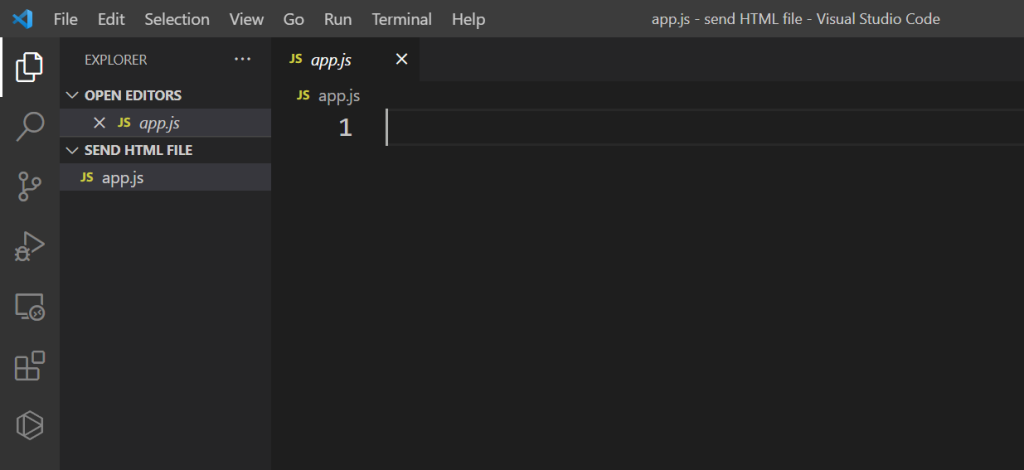
Step 3: Create another file “index.html” which will send as a response to the client.
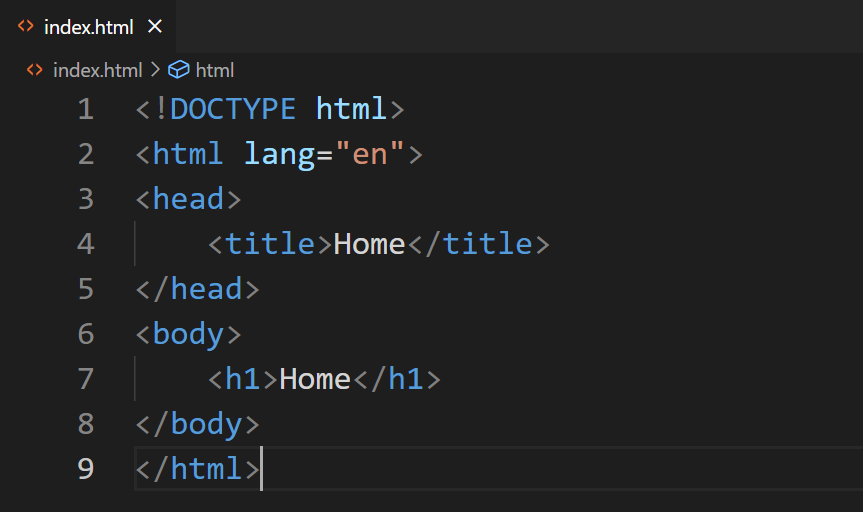
Step 4: Open the terminal & type the below command. This initializes the Node Packages Manager, by which we can install any Node.js framework.
npm init -y
Output:
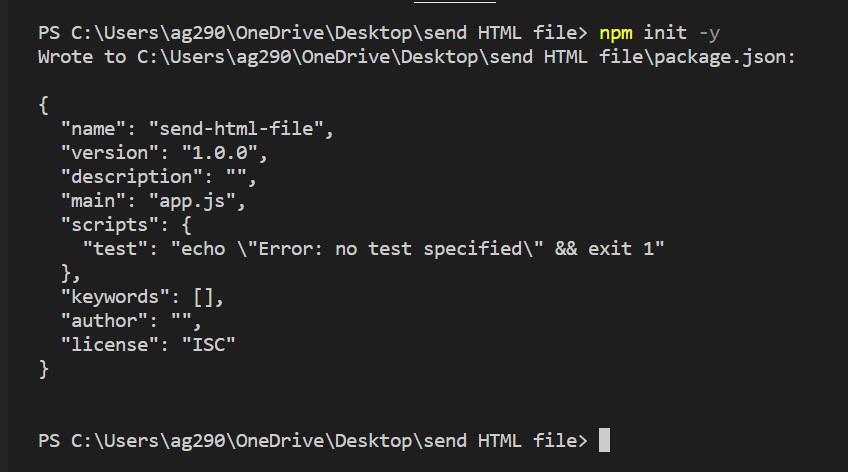
Step 5: Inside the terminal, type the below command to install express as a module in the project.
npm i express
Output:
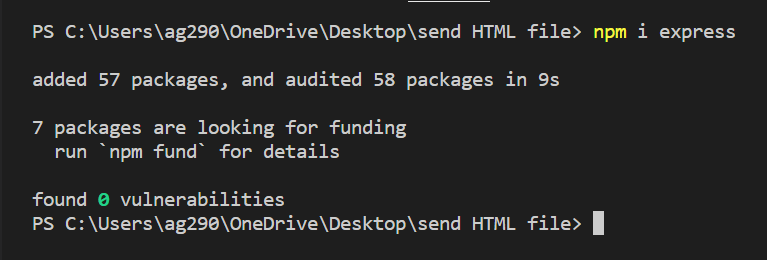
Step 6: Open the “app.js” file and import the express module using the below statement.
const express = require('express');
Step 7: Create a constant “app” and set it to “express()”, which means we are creating an instance of an express module, by which we can call any of the express functions.
const app = express();
Step 8: Then write the following code
app.get('/', (req, res) => {
});
Here, we use the express get method, which defines what happens when the client requests for the “/” route, after that we separate it using a comma and pass a callback function.
Inside the callback function, write res.sendFile(), res means response, the response object is used to send different types of data to the client, and the sendFile() is a method of response object by which we send the HTML file as a response.
app.get('/', (req, res) => {
res.sendFile();
});
If you want to send JSON instead of HTML content, you can use the express’s json() method.
Inside the sendFile method, type the file location that you want to send as a response.
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
If you are wondering why we use __dirname, you can check out NodeJs Globals where we have explained many global objects including __dirname.
Step 9: After that, create a port in order to test our application on browsers, for this, we will use the express method “listen”, by using our “app” instance and pass a port as an argument which is used to open the application in the browser.
app.listen(3000);
Step 10: Now, open any web browser and type the following, inside the search bar, this opens the Node.js application we set to port 3000.
http://localhost:3000/
Complete Code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
app.listen(3000);
Output:
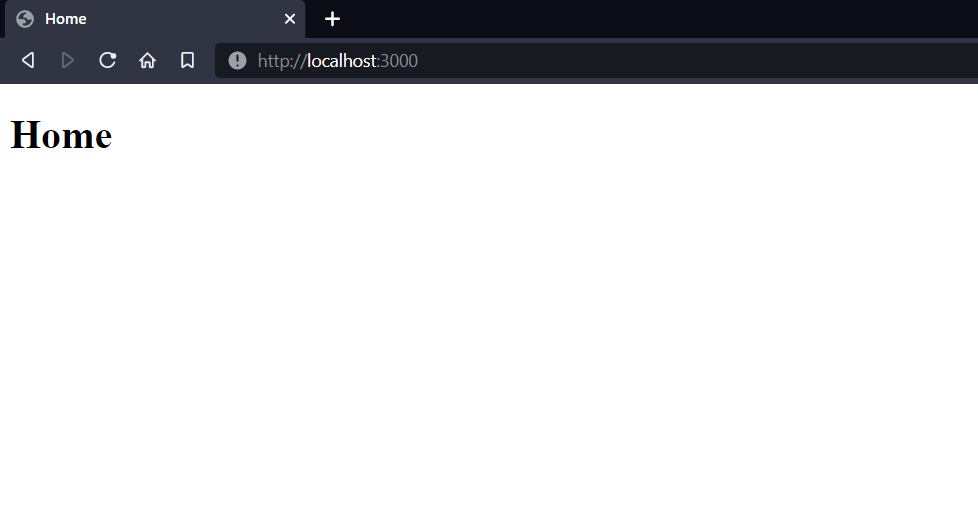
Frequently Asked Questions (FAQs)
How to use Node.js as the backend?
To use Node.js as the backend, you first have to install it on your system, then use its HTTP module to create a server, write JavaScript code for functionality and then execute it using the node <filename> command.
Is Node.js good for the backend?
Yes, Node.js provided all the essential tools and frameworks necessary for developing the backend system. Its event-driven, non-blocking architecture makes it super fast in handling multiple requests simultaneously.
Why not use Node.js for the backend?
While Node.js is faster than other technologies, it has a limitation. It is single-threaded it can’t be used for high computation tasks that need intense CPU processing.
Is npm a web server?
No, NPM stands for Node Package Manager, used to install different Node.js packages. NPM is the core of Node.js, it is an online repository of thousands of Node.js packages used for different functions. These packages are free to install and can be used in the creation of different types of applications.
Why Node.js is fast?
Node.js is faster due to its asynchronous nature. It doesn’t block the execution of other programs while executing one, hence executing multiple blocks of code simultaneously will increase overall speed and performance.
What is the best database for Node.js?
There is no particular database that fits Node.js, but it does work well with NoSQL databases like MongoDB by offering flexibility in schema design and data types.
Do I need HTML for Node.js?
If you just want to create servers, APIs or microservices with Node.js, it doesn’t require an HTML file. But if you want to create a fully fledge web application rendering and displaying web pages in browsers, HTML becomes essential.
How to send HTML from Node.js?
You can send HTML from Node.js by using the sendFile() function from the Express module. Additionally, if you want to send content from Node.js to an HTML page you can use a templating engine like EJS as well.
Summary
We can send an HTML page while creating a Node.js application, for this, we have to use the express.js module method sendFile(). You can also attach CSS in HTML for custom design. We can send multiple files for different routing requests. You can also read HTML form data using Node.js. We hope this tutorial helps you to render HTML using Node.js.
Reference
https://stackoverflow.com/questions/20345936/nodejs-send-html-file-to-client