Welcome to the next chapter of our NumPy logarithmic series. I hope you’ve gone through the first one, i.e., Log10. Here we will discuss another important log function, which is log2. It helps us to calculate the logarithm base 2 of the input. We will delve into its concept, syntax, parameters, and real-life use cases, so let’s continue further.
What is log2?
This mathematical function tells us how many times we have to multiply the base (2) by itself to get the given number.
Let’s say you have a number, like 8. Now, you want to know how many times you need to multiply 2 by itself to get that number. That’s where log2 (pronounced “log base 2”) comes in.
For example, with 8, you write it like log2(8) = x. The ‘x’ tells you how many times you need to multiply 2 to get 8. Here, x is 3 because 2 multiplied by itself 3 times equals 8.
Here’s how it goes:
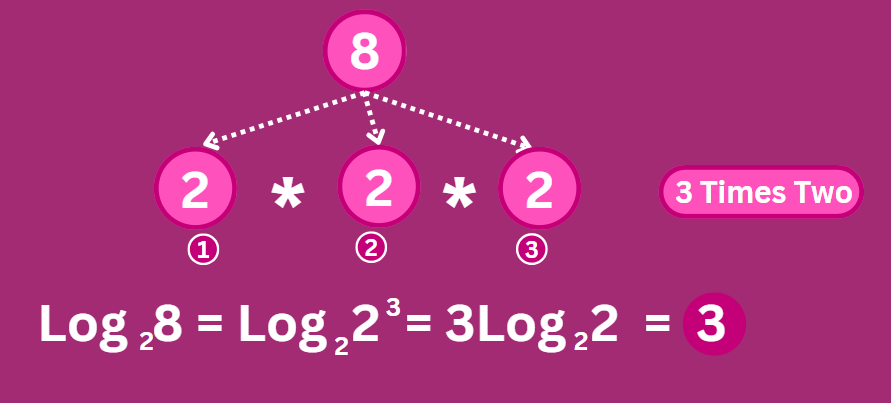
This simple idea of log2 is used in many fields, especially in computer science. It helps figure out things like how fast a computer algorithm works. For example, in binary search, log2 is used to know how many steps it takes to find something in a sorted list.
So, log2 helps us understand how things grow exponentially and is very helpful in many situations.
numpy.log2() in Python
This Numpy function finds the log of numbers, but only to the base 2. We can use it on lists or arrays of numbers, and it gives us back a new list or array where each number has been turned into its base-2 log. So, if we have a list of numbers like [2, 4, 8], numpy.log2() will give us [1, 2, 3].
Syntax:
Let’s take a glimpse at its syntax:
numpy.log2(x, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature, extobj])
The parameters used here are:
- x: Input array or object.
- out: Output array where the result is placed.
- dtype: Data-type of the output. If not given, infer the data type from x.
- where: This parameter is used to select elements based on conditions.
Working:
Here’s an explanation of how numpy.log2() works:
- Input: We give it a bunch of numbers, like [1, 2, 4, 8, 16].
- Operation: It calculates the base-2 logarithm for each number. For example, log2(1) is 0, log2(2) is 1, and so on.
- Output: It gives us back a new list with the results, like [0, 1, 2, 3, 4].
It’s fast because it can handle lots of numbers at once, making it quicker than doing each calculation one by one. And it’s versatile too, able to handle different types of data for all sorts of tasks in Python.
Using numpy.log2() in Python
Now we will see how we can implement the Numpy log2() function in Python. Observe the given examples:
Example 1:
Let’s start by testing it on a single number.
import numpy as np
number = int(input("Please enter an integer: "))
result = np.log2(number)
print("2 will multiply itself", result, "times to get the given number.")
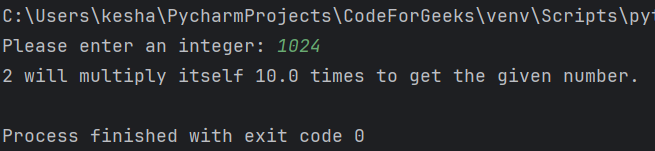
Example 2:
Now we will calculate log2 for each element in a 1-D array.
import numpy as np
arr = np.array([64, 32, 4, 128, 16])
result = np.log2(arr)
print("Here is the array containing power of two of each element in array: ", result)

Example 3:
The log2() function is able to perform broadcasting. Here, let’s test it by broadcasting a scalar quantity.
import numpy as np
scalar = 4
arr = np.array([0, 4, 16, 8, 64])
result = np.log2(scalar * arr)
print(result)
In the line result = np.log2(scalar * arr), we do two things:
- First, we quadruple each number in the array arr by multiplying it by the scalar value (which is 4).
- Then, we find the base-2 logarithm of each of these quadrupled numbers. This tells us how many times we need to multiply 2 by itself to get each of the quadrupled numbers.
- Also for 0, the logarithm of 0 is undefined, so it will be represented as −∞.
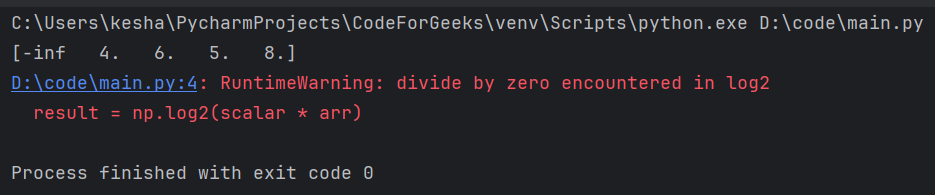
Example 4:
Let’s try to use the where parameter in this function.
import numpy as np
arr = np.array([0, 8, 2, 64, 4])
condition = (arr > 4)
result = np.log2(arr, where=condition)
print(result)
In this example, since the condition (arr > 4) is true only for elements 8, and 64, logarithms are calculated only for these elements, resulting in nan or garbage value for the rest of the elements.

Summary
And that’s all you need to know about the log2() function in NumPy. We have gone through the idea of logarithm base two, its use, and working. Now you are geared up with the knowledge of its syntax, parameters, and visualization through examples and implementations.
Do you like the explanation of this logarithmic function? If yes, then do read about the next function, i.e., log1p().
Reference
https://numpy.org/doc/stable/reference/generated/numpy.log2.html