Hello Coders! We are all aware of the power of the NumPy library. It is great for our mathematical calculations. One such mathematical calculation includes logarithmic computation, and yes, NumPy has a logarithm function that helps us perform such complex calculations. In this article, let’s learn about the log10 function, its format, parameters, and example use cases. So, let’s start.
What is log10?
The logarithm base 10 of a number, written as “log(x)”, tells you what power of 10 equals that number “x”.
Suppose you have a number, say 100. Now, to find out how many times you have to multiply 10 by itself to get 100, you use log base 10. In this case, log base 10 of 100 tells you that you need to multiply 10 by itself twice (because 10 * 10 = 100). So, log base 10 of 100 is 2.
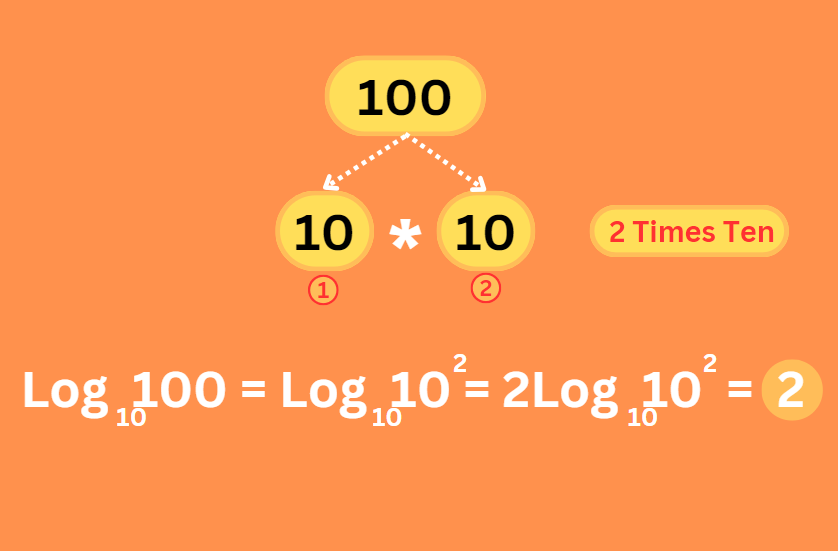
For example:
- log₁₀(100) = 2 because 10² = 100
- log₁₀(1000) = 3 because 10³ = 1000
- log₁₀(10) = 1 because 10¹ = 10
Logarithms are important because they:
- Condense big numbers.
- Solve complex equations.
- Help understand scales.
- Analyze data efficiently.
- Measure algorithm complexity.
- Assist in probability and statistics.
numpy.log10() in Python
Now that we understand what logarithmic base ten is and why we use it, let’s see how we can implement it in programming. We achieve this using NumPy’s log10() function.
Syntax:
In Python, we write it like this:
numpy.log10(x, out=None, where=True, casting='same_kind', order='K', dtype=None, ufunc 'log10')
Here is what each of the parameters used here means:
- x: This is the input. It can be a single number, a list of numbers, or a sequence of numbers.
- out: This is where the result will go. It needs to be the same size and shape as what you expect to get.
- where: This tells us where the data in ‘x’ is valid. It can be a true/false array or condition.
- casting: This tells us how to convert data types if needed.
- order: This decides how the output’s memory is organized.
- dtype: This tells us what type of data the output array should have.
- ufunc ‘log10’: This is the universal function used for the operation.
Working:
For each number in the input array ‘x’, numpy.log10() finds its base 10 logarithm. It gives back an array with the same shape as the input, where each number is the base 10 logarithm of the corresponding number in the input. If you pick the ‘out‘ option, the result goes into that array; if not, it makes a new one.
Using numpy.log10() in Python
I assume you’re clear with the function provided so far, but for better understanding, let me guide you through some examples.
Example 1:
Let’s start with a simple example using a scalar input.
import numpy as np
user_in = float(input("Enter a number: "))
result = np.log10(user_in)
print("Log base 10 of", user_in, "is:", result)
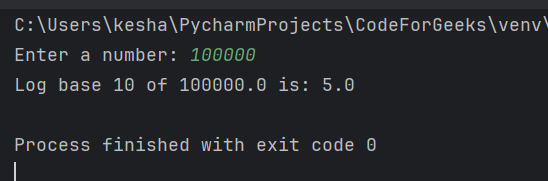
Example 2:
Now let’s see how it works with 1-D array input.
import numpy as np
x = np.array([100, 1000, 100, 1000])
result = np.log10(x)
print("The array containing the log base 10 value of each element is ",result)

Example 3:
This function performs similarly with a 2-D array.
import numpy as np
x = np.array([[2, 5, 8], [15, 20, 25]])
result = np.log10(x)
print("The array containing the log base 10 value of each element is ", result)

Example 4:
Now we will see how we can use the where parameter.
import numpy as np
x = np.array([5, 10, 15, 20])
result = np.log10(x, where=x > 10)
print("The array containing the log base 10 value of each element is ",result)
This example calculates the base-10 logarithm only for elements in the array x where the value is greater than 10. For the rest, it will either show ‘nan’ or throw garbage.

Example 5:
This function returns the NaN value if we provide a negative input value.
import numpy as np
x = np.array([-100, 1000, -100, 1000])
result = np.log10(x)
print("The array containing the log base 10 value of each element is ", result)

Summary
And yes, that’s it for the first logarithmic function we discussed in NumPy. Log10 is a very useful function. Here, we have discussed its core concept, working, and syntax. We have not only gone through theory but also seen practical examples and studied its behaviour for different kinds of inputs.
We have more in the NumPy logarithm series, such as NumPy log2 and NumPy log1p, so be sure to check them out!
Reference
https://numpy.org/doc/stable/reference/generated/numpy.log10.html