Maps in JavaScript store key-value pairs where keys can be of any type. It works well for adding and removing key-value pairs, making them suitable for applications in which performance is important. It also remembers the insertion order, ideal for sequence-dependent operations.
How to Create a Map?
There are two ways in which we can create a map.
1. The new Map() Method
We can pass an array to the new map() constructor to create a map.
const fruits = new Map([
['India', 'New Delhi'],
['Australia', 'Canberra'],
['France', 'Paris']
]);
Elements in the array become key-value pairs, such as ‘India’ as the key and ‘New Delhi’ as its corresponding value.
2. The set() Method
We can add elements to a map using this method.
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
Here, an empty map is created using the new map() constructor, and then using the set method, we add the key-value pairs to it. With every new line of Country.set, a new key-value pair is pushed into the map.
Now if we print the given map, we see that all these key-value pairs are added to a single map, and all the keys are displayed along with their value pairs.
console.log(Country);
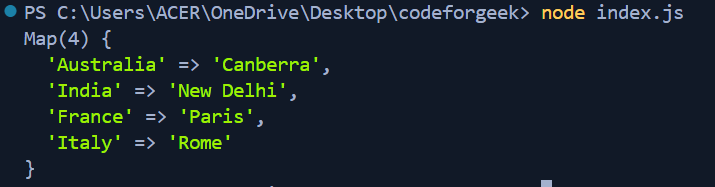
Essential Map Methods in JavaScript
The map object provides several methods to work with key-value pairs. Let’s look at them one by one.
1. The get() Method
This method allows us to access the value of a specific key. It returns the value pair corresponding to the provided key.
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
console.log(Country.get('France'));
Here it will return the value of ‘France’ which is ‘Paris’.

2. The has() Method
This method enables us to check for a key’s existence. It returns true if the key is present in the map and false if the key is absent in the map.
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
console.log(Country.has('France'));
console.log(Country.has('Britain'));
In this case, our map has ‘France’, hence it will return true, and for “Britain,” it returns false as it’s absent.

3. The delete() Method
This method removes a particular key along with its paired value.
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
Country.delete('Australia');
console.log(Country);
Here, this method will delete ‘Australia’ from the map and then the updated map will be displayed.
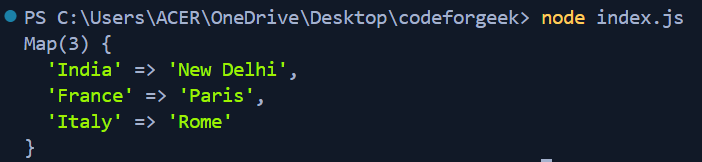
4. The clear() Method
This method deletes all the keys and their values at once. The map gets empty on deletion.
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
Country.clear();
console.log(Country);
In this case, all the keys get cleared from the map, and a null map is displayed.

5. The size() Method
This method helps us to know the size of our map. It returns the size of the map, i.e., the count of keys present in the map.
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
console.log(Country.size);
An integer value (4) is displayed.

Alternative:
const Country = new Map();
Country.set('India', 'New Delhi');
Country.set('Australia', 'Canberra');
Country.set('France', 'Paris');
Country.set('Italy', 'Rome');
console.log(Country);
If you have noticed, when we print our map using the ‘console.log(Country)’, there is a ‘Map(4)’ before elements. The 4 here is nothing but the size of the map.
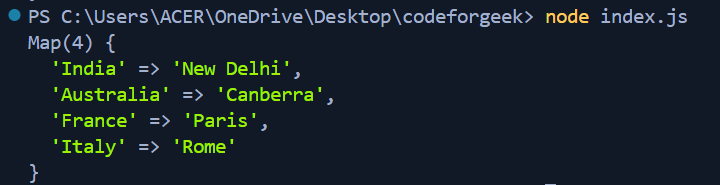
Iterating through a Map in JavaScript
Iteration helps us to display the key-pair values in our map on the console or perform any element-wise operation. Let’s see how to iterate through it.
1. Using for…of
This method allows you to iterate over the entries of a Map object directly.
Syntax:
for (let [key, value] of map) {
console.log(key, value);
}
Example:
const map = new Map();
map.set('Google', 'Sundar Pichai ');
map.set('Meta', 'Mark Zuckerberg');
map.set('Microsoft', 'Satya Nadella');
for (let [key, value] of map) {
console.log(`${key}: ${value}`);
}

2. Using forEach
This method uses a callback function where the first parameter is the value and the second is the key.
Syntax:
map.forEach((value, key) => {
console.log(key, value);
});
Example:
const map = new Map();
map.set('Jupiter', 'Giant Among Giants');
map.set('Neptune', 'Blue Giant');
map.set('Saturn', 'Ringed Planet');
map.forEach((value, key) => {
console.log(key, value);
});

Using Functions as Keys in JavaScript Map
When we assign a key to a map, it doesn’t need to be an object. The maps provide us with the facility to use functions as keys. The function that needs to be added as a key should be defined first. Then we can assign values to it.
const square = function(num) {
return num * num;
};
const cube = function(num) {
return num * num * num;
};
const map = new Map();
map.set(square, 5);
map.set(cube, 3);
console.log(`Square of 5 is: ${square(map.get(square))}`);
console.log(`Cube of 3 is: ${cube(map.get(cube))}`);
If you carefully look at the line: ${square(map.get(square))}, here firstly we get 5 from map.get(square), then square(5) results in 25.

Conclusion
Using maps is very useful as it supports the key of any datatype making it best for different use cases. Also, elements are iterated in the order in which they were inserted hence the order remains preserved. This works well to store and retrieve data frequently. Feel free to experiment with key-value management in your code.
And readers, you may have heard about Next.js. It is a popular React framework in web development. If you are interested click here to go to our exclusive Next.js series!
Reference
https://stackoverflow.com/questions/47778935/creating-a-javascript-map