A MongoDB database can have multiple collections, which can contain lots of documents. To find documents based on custom queries, the MongoDB Node.js driver provides us with two useful methods: find() and findOne(). Let’s see them in detail.
How to Query MongoDB Documents in Node.js
MongoDB database is implemented in NodeJS using the MongoDB module, which provides a MongoClient which then has a method connect() for connecting to your MongoDB instance, inside the callback it returns a database object which is used to select a collection using collection() method, when done selecting a collection then the methods like find() and findOne() can use accordingly to select the document.
MongoDB findOne() Method to Find One Document
MongoDB findOne() method finds a document from a collection. If many documents match the query then it selects the first one.
This method can take an object and a callback function as an argument. Inside the object, the MongoDB query can be passed to filter the data selection. Inside the callback, it returns the error if occurs or returns the selected document if a match is found.
Syntax of findOne():
db.collection(collectionName).findOne({}, function(err, document) {
if (err) throw err;
console.log(document);
});
Here:
- db is a database connection object,
- collectionName specifies the name of a collection to look for the documents
Example of findOne():
We have multiple documents with multiple fields in a collection.
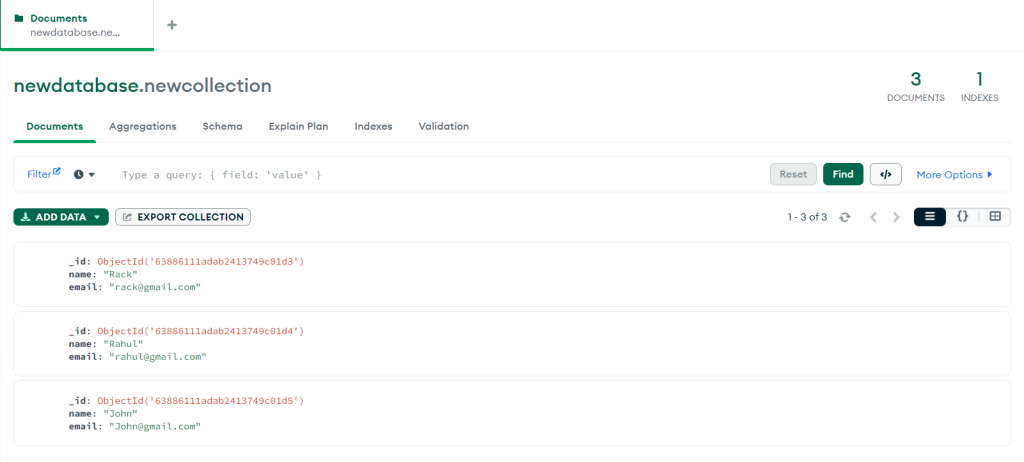
Click here if you want to learn how to insert documents.
Code to select a single record:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").findOne({}, function(err, document) {
if (err) throw err;
console.log(document);
});
});
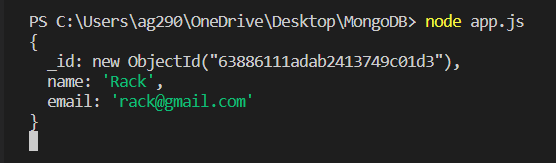
MongoDB find() Method to Find Multiple Documents
MongoDB find() method finds multiple documents from a collection. It can select all the documents from a collection. It is equivalent to SELECT * in MySQL.
This method takes a query object as an argument used to filter the documents to select the documents on the basis of some conditions. If the query is not passed then select all the documents from a collection. This method returns an object which can be converted into an array containing each document as a separate object.
Syntax of find():
db.collection(collectionName).find({}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
Here:
- db is a database connection object,
- collectionName specifies the name of a collection to look for the documents
Example of find():
If you want to select all the records from a MongoDB collection, you just need to leave the query object empty.
Code to select multiple records:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").find({}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
});
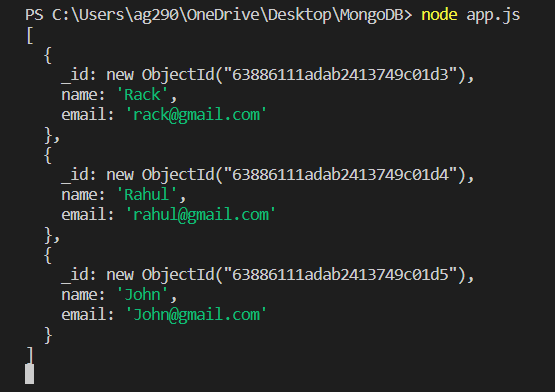
Frequently Asked Questions (FAQs)
How to find documents in MongoDB?
We have two popular methods to find documents in MongoDB: find() for multiple documents and findOne() for a single document.
How to query multiple documents in MongoDB?
We can use the find() method to query multiple documents from a MongoDB collection. For example, “db.collection(‘users’).find({ age: { $gt: 18 } })” to get all documents with an age field greater than 18.
How do I search for only one document in MongoDB?
To search for only one document in MongoDB, we can use the findOne() method. For example, “db.collection(‘users’).findOne({ age: { $gt: 18 } })” to get the first documents with an age field greater than 18.
How do I use multiple queries in MongoDB?
We can chain multiple queries using operators like $and, $or in the find() method. For example, “db.collection(‘users’).find({ $and: [{ age: { $gt: 18 } }, { age: { $lt: 30 } }] })
“ to get all documents with an age field greater than 18 but less than 30
How do I list all documents in MongoDB?
To list all documents from a MongoDB collection, we can use the find() method and leave the query criteria empty. For example, “db.collection(‘users’).find({})” to get all documents from a collection named users.
Summary
MongoDB can hold numerous documents in the collection and it can be difficult to find the required document among them. Well, we have find() and findOne() methods.
The find() method can select multiple documents whereas findOne() is limited to selecting only one of many documents. Both take a query parameter to filter the selection. If you want to know what query we are talking about and how to create that click here.