The forEach() method in Node.js is a basic tool for looping across arrays and performing an action on each element. This technique provides a clear and legible syntax while streamlining the process of applying actions to each element in an array.
Node.js forEach() Method
The forEach() method iterates over each element in an array and executes a provided callback function once for each element. This callback function can perform various operations on each element.
Syntax:
array.forEach(function(currentValue, index, arr), thisValue)
Parameters:
- function: The function to execute for each element in the array.
- currentValue: The value of the current element.
- index (Optional): The index of the current element.
- arr (Optional): The array “forEach” was called upon.
- thisValue: The value that should be used as the “this” value in the callback function.
Let’s now see the examples and use cases of the forEach() method in detail to understand its working.
Logging Array Elements Using forEach() Method
The forEach() method can be used to iterate through each element of the numbers array and log each element to the console.
Example:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
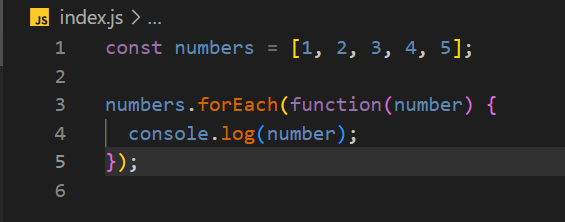
The forEach() method is used to execute a provided function once for each array element. The function that is being supplied in this instance is anonymous and accepts a single parameter: number, which represents the element that is being iterated over at the moment. The function body calls console.log(number), which outputs each array element to the console.
Output:
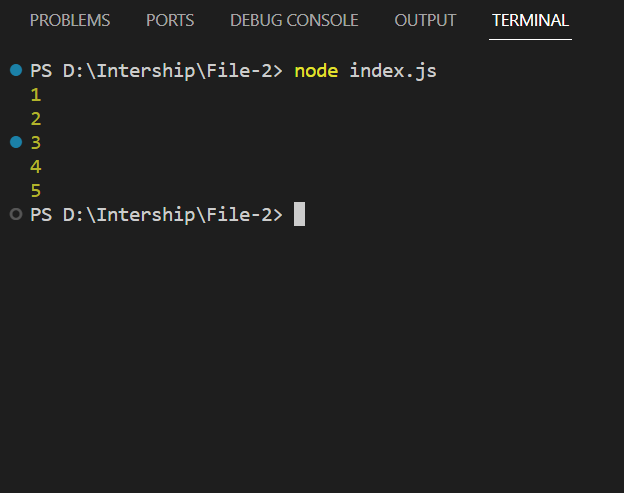
Accessing Array Index Using forEach() Method
We can access the index of each element along with the element itself and print both to the console using the forEach() method.
Example:
const fruits = ['apple', 'banana', 'orange'];
fruits.forEach(function(fruit, index) {
console.log(`Fruit at index ${index} is: ${fruit}`);
});
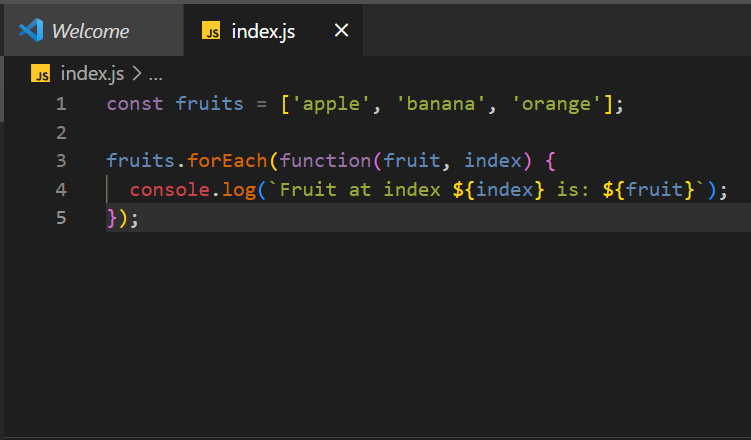
In this case, the callback function is an anonymous function that takes two parameters: fruit, representing the current element being iterated over, and index, representing the index of the current element in the array. Inside the function body, a string template is used to log a message to the console, indicating the fruit at the current index.
Output:
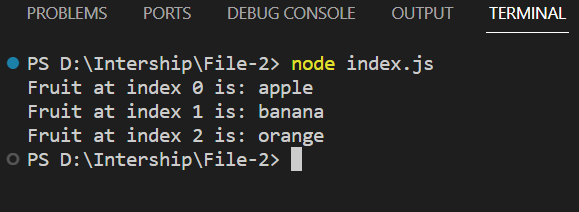
Modifying Array Elements Using forEach() Method
The forEach() method can be used to modify the array. In this example, we have doubled each element of the numbers array.
Example:
const numbers = [10, 20, 30];
numbers.forEach(function(number, index, array) {
array[index] = number * 2;
});
console.log(numbers);
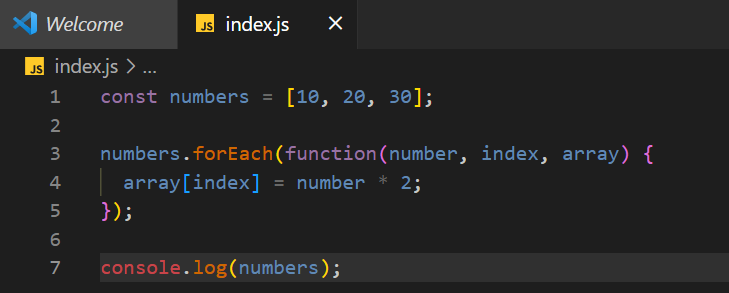
In this case, the function passed takes three parameters: number, representing the current element being iterated over, index, representing the index of the current element in the array, and array, representing the array itself. Inside the function body, each element of the numbers array is multiplied by 2 (number * 2), and the result is assigned back to the corresponding index in the numbers array.
Output:
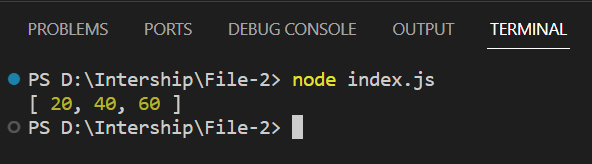
Chaining forEach() with Other Methods
We can also use the forEach() with other array methods. By using other array methods such as map, filter, and reduce, the forEach() method opens a world of possibilities for creating sophisticated data processing workflows.
Example:
const numbers = [1, 2, 3, 4, 5];
numbers
.map(number => number * 2)
.filter(number => number % 3 === 0)
.forEach(number => {
console.log(number);
});
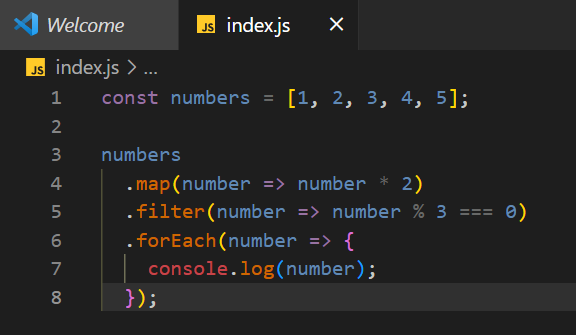
In the above script, the method chaining is performed on the numbers array as the map() method is called on the numbers array. It iterates over each element of the array and doubles its value. After the operation, the array will be modified as [2, 4, 6, 8, 10].
The filter() method is then called on the mapped array. It iterates over each element and filters out those elements that don’t satisfy the condition specified in the callback function (number % 3 === 0). So, after this operation, the array becomes [6], as only the number 6 is divisible by 3.
Finally, the forEach() method is called on the filtered array. It iterates over each element and executes a provided function once for each array element. Therefore, when this code is executed, it will log the number 6 to the console.
Output:
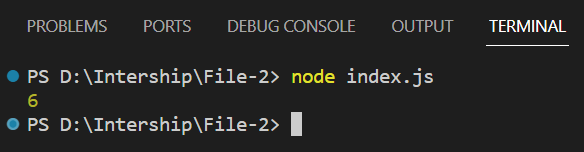
Error Handling in forEach() Method
It’s important to handle errors gracefully. Ensure error handling within the callback function to prevent unexpected failures. Use try-catch blocks or conditionals to handle errors appropriately.
Example:
array.forEach(item => {
try {
// Some operation
} catch (error) {
console.error('Error processing item:', error);
}
});
Within the body of the function is a try-catch block. The primary operation on each item should take place in the try block. The catch block will capture any errors that arise when the action inside the try block is being executed. The catch block will use console.error() to record an error message stating that there was a problem processing the current item.
Benefits of Using forEach() Method
The forEach() method in JavaScript provides several benefits:
- Simplicity and Readability: The forEach method has more concise and legible syntax than the traditional loops making code readability and maintenance easier.
- Functional Programming Paradigm: By encouraging the usage of immutability and higher-order functions, this method conforms to the fundamentals of functional programming.
- No Loop Counter Variable Is Needed: Unlike conventional loops, forEach eliminates the option of an unintentional change occurring inside the loop body by not requiring a loop counter variable.
- Support for Method Chaining: We can perform complex operations more effectively by using the other array methods in combination with this method.
It’s important to keep in mind that the JavaScript forEach() function has certain restrictions despite its many advantages. An important limitation is that it cannot provide early loop termination like conventional loops do with the “break” statement, or early return with “return”.
Conclusion
In conclusion, the forEach() method, while a powerful and versatile tool in JavaScript, should be used judiciously in comparison to other array iteration methods. It provides a clear and clean way to iterate across arrays and run custom logic for each element. It is popular among programmers due to its readability and simplicity. It’s crucial to recognize its restrictions, such as the inability to return a value or break out of the loop. On the other hand, more intricate processes like reduce, filter, and map frequently provide simpler, more understandable answers. By being aware of the advantages and disadvantages of each technique, developers may select the best one for the particular use case at hand, increasing the readability, maintainability, and general effectiveness of their code.
Continue Reading:
- NodeJS Loops: A Beginner’s Guide to Loops in NodeJS
- Remove Duplicate Values From Array In JavaScript
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach