Have you ever wondered how to turn those cool SVG images into regular PNGs using Node.js? Well, buckle up! In this article, we will learn about the Sharp library and how to use it to convert an SVG to PNG. Are you ready to dive into the world of pixels? Let’s get started with the Introduction!
What is Scalable Vector Graphics?
Scalable vector graphics or SVG is a special kind of image format. Unlike regular pictures, SVG images are like flexible drawings that can change size without losing their quality. They use a special code called XML to describe the shapes and colours. SVG images are great for websites that need different-sized images. They can move, change colours, and even respond to clicks.
Need for SVG to PNG Conversion
Sometimes, we need to change SVG pictures into PNG format:
- Some internet browsers from the past don’t understand SVG well. So, turning SVG into PNG helps our pictures show up nicely everywhere.
- When we want to share our pictures with others, turning them into PNG files can be handy because many people are familiar with PNG images.
- PNG images load fast on websites, making sure people don’t have to wait too long to see cool pictures.
Moving on, we’ll show you the easy-peasy way with Sharp. Just simple steps, clear explanations, and a bit of Node.js magic.
Node.js Sharp Library
Sharp in Node.js is a speedy image-processing wizard. This library provides a range of functionalities for tasks such as resizing, cropping, rotating, and compressing images. It is beginner-friendly which makes it perfect for websites and apps. It is a trusted tool, always getting better with regular updates.
Installation:
To use Sharp in your Node.js project, you need to first install it. Run the given command in your terminal:
npm install sharp
Output:
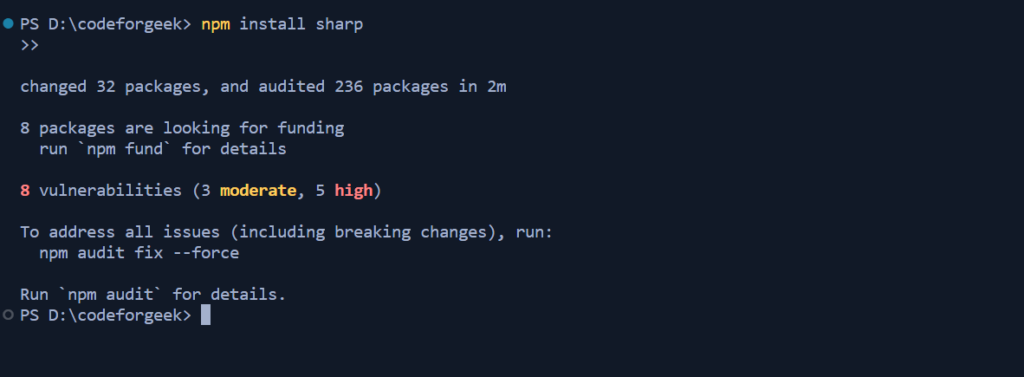
If you have the same display as the above then the installation is successful and ready for image magic in your Node.js project.
Convert SVG to PNG Using Node.js & Sharp Library
To learn the basic steps of using Sharp to convert an SVG to PNG, let’s go through an example.
Imagine you have a project, and in that project, you want to create drawings of trees in SVG format. Later, you also want to convert these SVG drawings into PNG images. Let’s walk Stepwise.
Step 1:
Create a file named index.js and write the following code in it.
const fs = require('fs');
const sharp = require('sharp');
// Create a simple SVG content
const svgContent = `
<svg width="200" height="300" xmlns="http://www.w3.org/2000/svg">
<rect width="100%" height="100%" fill="skyblue" />
<!-- Tree trunk -->
<rect x="80" y="150" width="40" height="100" fill="saddlebrown" />
<!-- Tree leaves -->
<circle cx="100" cy="100" r="60" fill="forestgreen" />
<circle cx="140" cy="140" r="50" fill="forestgreen" />
<circle cx="60" cy="140" r="50" fill="forestgreen" />
</svg>
`;
// Save the SVG content to a file
fs.writeFileSync('example.svg', svgContent, 'utf-8');
// Convert SVG to PNG using sharp
sharp('example.svg')
.png()
.toFile('example.png')
.then(() => {
console.log('SVG successfully converted to PNG');
})
.catch(error => {
console.error('Error converting SVG to PNG:', error);
});
Explanation:
- We use the fs library to help with reading and writing files and the sharp library for converting images.
- Then we write the XML SVG code, This is like giving instructions to draw a sky-blue background, a brown tree trunk, and green leaves. And we are wrapping these instructions in a variable named svgContent.
- It is then saved in a file called example.svg.
- Then, the drawing that was saved as example.svg is to be taken and transformed into a picture file named example.png. If the process goes smoothly, you get the “SVG successfully converted to PNG” message displayed. However, if any issues arise, the error is shown.
Step 2:
Run the following command in the terminal to run your node file.
node index.js
Output :

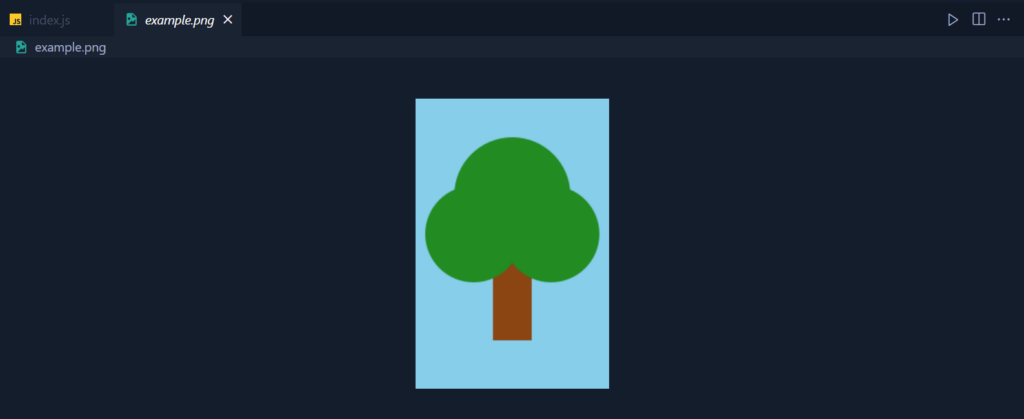
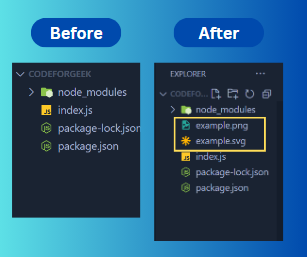
And that’s it! We have just created a project where we have a tree SVG and then we turn it into a PNG image.
Performance Benefits of Using Sharp for SVG to PNG Conversion
Sharp is fast as it uses native bindings to low-level libraries like libvips for quicker performance compared to pure JavaScript solutions. It is ideal for handling multiple conversions simultaneously. It is also recognized for its low memory usage, making it efficient for processing large images. Sharp supports streaming, enabling image processing without loading the entire image into memory.
Sharp is faster than PhantomJS for SVG to PNG conversion, especially in server-side applications. It also surpasses Inkscape and CairoSVG as it excels in large-scale batch processing.
Conclusion
This article is your guide to using the Sharp library in Node.js to turn cool, flexible SVG drawings into regular PNG images. It explains why you might want to do this and walks you through the process with a simple example. Sharp is highlighted for its speed and efficiency, making the conversion a breeze. Hope this makes it clear and boosts your confidence with Node.js image magic!
Further Reading:
- Create a Simple Static File Server with Node.js: A Beginner’s Guide
- Node-gyp Explained: Integrating Native Modules in Node.js
- What Is the Purpose of ‘node_modules’ Folder?
Reference
https://www.npmjs.com/package/sharp