A drawer is an off-canvas menu that is initially hidden. Once the user clicks on an icon, the drawer slide in from either the left or right part of the screen. It is usually used to create a menu that is not shown to the user in the beginning.
This tutorial will teach about Flutter Drawer, one of the most basic user interface-related topics in Flutter app development. We’ll see some of the theories regarding this and then write the code to create our own drawer. So let’s dive into the interesting stuff.
Also Read: Flutter Basic Widgets
Create Flutter Drawer
In flutter, the drawer is made default via AppBar. In the top left part of the AppBar, there will be an icon that will be used to toggle the Drawer.
We have already covered the process of building AppBar from scratch in another tutorial Flutter App Bar: A Step-by-Step Guide, if you want to read it.
We believe in practical knowledge so without much more discussion let’s code drawer from scratch.
Project Structure
The project contains a Dart file which will contain all the application code. This file is the main.dart file and it is located in the lib folder.
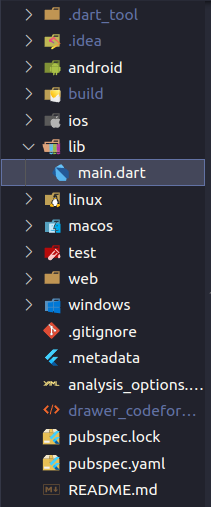
Setup Flutter App
First of all, let’s set up a basic flutter app with a main function and a stateless class to create a stateless widget.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp();
}
}
You can use the “stf” shortcut in VS Code to create a stateless widget.
Removing debug banner and adding Scaffold
Next, inside the material app, we are going to specify debugShowCheckedModeBanner to false so that we can get rid of that ugly debug sign that is shown on top of the screen which shows that app is in debug mode not in production or release mode.
Also, inside the home, we are passing the scaffold widget so that we can use all the properties such as App Bar and Drawer that are required for what we are trying to achieve.
Scaffold is a base for UI components such as AppBar, BottomNavigationBar, and FloatingActionBar. If we need to use them in our app then we will always need Scaffold.
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(),
);
Adding AppBar containing the title
Now let’s add AppBar and add a title to it. So that we can have a bit more UI ready for our project.
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: const Text('Drawer Tutorial'),
),
),
);
Now we are all set to write our drawer code.
Code for creating Flutter Drawer
Add the following code just below the AppBar. Make sure that it should be inside Scaffold.
drawer: Drawer(
child: Column(
children: [
Container(
width: double.infinity,
height: 200,
color: Colors.blueAccent,
child: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.end,
crossAxisAlignment: CrossAxisAlignment.start,
children: const [
Text(
'Hello',
style: TextStyle(
color: Colors.white,
fontSize: 36,
fontWeight: FontWeight.bold),
),
Text(
'Sushant Dhiman',
style: TextStyle(color: Colors.white, fontSize: 32),
),
],
),
),
),
Column(
children: [
const SizedBox(height: 20),
ListTile(
leading: Icon(
Icons.home,
color: Colors.grey.shade800,
),
title: const Text(
'Home',
style: TextStyle(fontSize: 18),
),
),
ListTile(
leading: Icon(
Icons.settings,
color: Colors.grey.shade800,
),
title: const Text(
'Settings',
style: TextStyle(fontSize: 18),
),
),
ListTile(
leading: Icon(
Icons.person,
color: Colors.grey.shade800,
),
title: const Text(
'Profile',
style: TextStyle(fontSize: 18),
),
),
ListTile(
leading: Icon(
Icons.help,
color: Colors.grey.shade800,
),
title: const Text(
'About Us',
style: TextStyle(fontSize: 18),
),
),
],
)
],
),
),
We know that it is a lot of code that we just showed above and it is hard to understand but don’t worry we will explain everything so that you can understand the complete code clearly.
Explanation:
- We’ve started with creating a Drawer instance inside Scaffold. If you look at the app now you will see a menu icon in the top left corner. Click that menu button, and the drawer will open but empty.
- Then we created a column because we wanted everything to align vertically.
- Then inside the column, we created the children’s argument. In this, we have a container as the first argument.
- The container’s width is “double.infinity” and the height is 200. In addition, there is a blue background.
- Inside the container, we have a column widget containing two text widgets. The first widget has “Hello” and the second has “Sushant Dhiman”. We have given them size and background colour using the “TextStyle” property.
- Then we have given padding from all the sides as the UI is glued to the sides of the screen.
- At this point, we’ve built the top portion of the drawer. Let’s focus on creating a menu.
- Those menu buttons you can see at the bottom of the output are ListTile widgets. It is nothing but a row of three widgets.
- For the first menu button, we have set the leading to an icon widget, which is basically a home icon, and colour it grey with a shadow of 800.
- Rest things are entirely the same, we have to copy and paste that list file widget and change the icon as well as the text.
- Then we wrapped all those list tile widgets inside a column because we want it to be arranged vertically again.
- To give some space at the top, we used the SizedBox widget and pasted it at a height of 20.
Complete Code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: const Text('Drawer Tutorial'),
),
drawer: Drawer(
child: Column(
children: [
Container(
width: double.infinity,
height: 200,
color: Colors.blueAccent,
child: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.end,
crossAxisAlignment: CrossAxisAlignment.start,
children: const [
Text(
'Hello',
style: TextStyle(
color: Colors.white,
fontSize: 36,
fontWeight: FontWeight.bold),
),
Text(
'Sushant Dhiman',
style: TextStyle(color: Colors.white, fontSize: 32),
),
],
),
),
),
Column(
children: [
const SizedBox(height: 20),
ListTile(
leading: Icon(
Icons.home,
color: Colors.grey.shade800,
),
title: const Text(
'Home',
style: TextStyle(fontSize: 18),
),
),
ListTile(
leading: Icon(
Icons.settings,
color: Colors.grey.shade800,
),
title: const Text(
'Settings',
style: TextStyle(fontSize: 18),
),
),
ListTile(
leading: Icon(
Icons.person,
color: Colors.grey.shade800,
),
title: const Text(
'Profile',
style: TextStyle(fontSize: 18),
),
),
ListTile(
leading: Icon(
Icons.help,
color: Colors.grey.shade800,
),
title: const Text(
'About Us',
style: TextStyle(fontSize: 18),
),
),
],
)
],
),
),
),
);
}
}
Output:
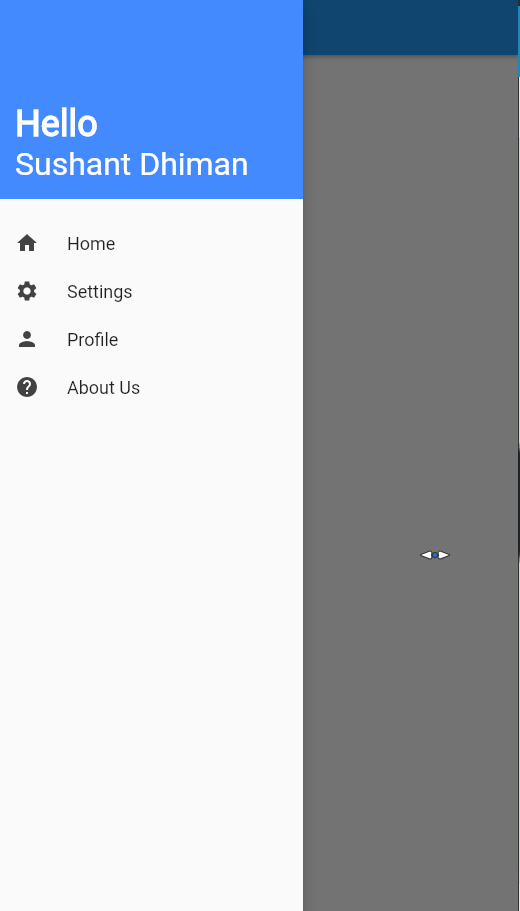
Summary
In this tutorial, we discussed some of the theoretical parts and the full implementation of Drawer in Flutter. After reading this tutorial, we hope you have a basic understanding of drawers. We just told you how to make things work and implement functionality, rest of depart is about creativity which you have to show on your own. You can be as creative as you want with the drawers. Thanks for reading.
Reference
https://docs.flutter.dev/cookbook/design/drawer