We are all aware of the growing popularity of QR code generators. Did you know that you can create your own QR code generator? With Python, it is possible to perform this magic. In this article, we will teach you about the amazing qrcode module in Python and show you step-by-step how you can create your own QR code generator.
Introduction to qrcode in Python
The qrcode module in Python helps you make QR codes quickly and easily. You can use it to create QR codes for different things like sharing web links or contact details. It’s handy because you can customize your QR codes, change colors or add logos. This tool is great for businesses, marketers, and anyone who needs to share information. It makes creating and using QR codes simple and effective, whether you’re online or offline.
Installation
To install the qrcode module in Python, you can use pip. Just open your command line interface and type the following command:
pip install qrcode
Hit Enter, and pip will download and install the qrcode module along with any dependencies it needs. Once the installation is complete, we can start using it in our Python projects.
Generating QR code using qrcode
A QR code is a square-shaped pattern made of black modules arranged on a white background. It stores information that can be read by a smartphone or a QR code scanner. When scanned, it can lead you to websites, display text, or perform other tasks. Let’s see how we can build an app that can generate a QR.
Step 1: Importing qrcode
Firstly, we will import the qrcode library, which contains functions to generate QR codes.
import qrcode
Step 2: Defining Data
Then we define the data that we want to encode into the QR code. In this case, it’s a URL to YouTube.
data = "https://www.youtube.com/"
Step 3: Generating QR Code
Now we will create a QRCode object named qr. We set parameters such as version (size), error correction level, box size, and border for the QR code. We add the data (URL) to the QR code. Finally, we generate the QR code with the make() function.
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
Step 4: Creating an Image from QR
Next, we will create an image representation of the QR code using the make_image() function. We specify the fill color (black) for the QR code modules and the background color (white).
img = qr.make_image(fill_color="black", back_color="white")
Step 5: Saving the Image
Then we will save the generated QR code image as “example.png” in the current directory.
img.save("example.png")
Step 6: Printing Success Message
Finally, we will print a success message to indicate that the QR code generation process is completed.
print("QR Code generated successfully.")
That’s it, you have created your own QR code. Now, let’s take a glimpse over the complete code, and then we will see the working of our application.
Complete Code
import qrcode
# Define the data to be encoded
data = "https://www.youtube.com/"
# Generate QR code
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
# Create an image from the QR Code instance
img = qr.make_image(fill_color="black", back_color="white")
# Save the image
img.save("example.png")
print("QR Code generated successfully.")
Output:
When we run the given code, we’ll get a QR code image file named “example.png” saved in the same place where we ran the code.

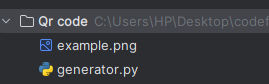
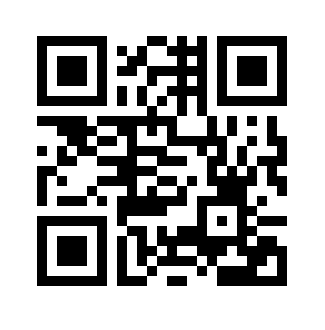
Let’s play along with the created QR code. Scan the given image with your scanner and see if you can enter the magical world of creative videos on YouTube.
Conclusion
We hope you now understand how to create your own QR code generator using Python. With the qrcode module, it’s easy to generate customized QR codes for various purposes. By following the steps outlined above, you can quickly create QR codes that link to your desired URLs. Try it out yourself and explore the possibilities of QR technology.
If you like this one, do check out –
- Create a Basic Calendar Application with Python Tkinter
- Creating a Temperature Converter in Python Using Tkinter
Reference
https://stackoverflow.com/questions/55269996/python-how-to-generate-qrcode