Readline Module is a built-in module in Node.js used for handling user inputs and providing resultant output to the command line. This module offers various methods to help interact with CLI. Using this tool we can create a fully functional CLI-based application. Let’s learn it in detail.
Introduction to Node.js Readline
The Readline Module is used to interact with the command-line interface (CLI) using which we can read user inputs and write our responses. This module implements the process input stream and output stream to give the functionality of reading input and writing output.
Example Usage:
Whenever we run “npm init” to initialize NPM, it prompts for multiple inputs to create a configuration file package-log.json. The same type of functionality can be achieved using this module.
Get Started with Node.js Readline
The Readline is a built-in package so no need to install it manually. All we have to do is import it into the script we want to use it in.
const readline = require('readline');
Let’s check it with real data.
Reading and Writing to the Console:
Let’s write some simple JavaScript code to create a program using the Readline interface to prompt the user for an input name and then log the message “Hello” concatenated with the entered value.
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.question('What is your name? ', (name) => {
console.log('Hello ' + name);
rl.close();
});
The first line is the importing statement. The next line creates a readline interface (rl) using the createInterface method having process stdin and stdout as parameters to read and write to the console. Then we invoke the rl.question() method to emit a message and receive the user input. The callback prints the results to the console and exits rl interface.
Output:
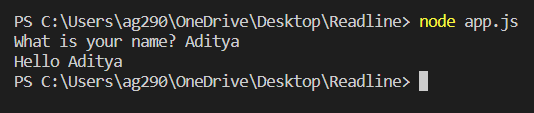
You can find more information about process.stdin and process.stdout in the “Node Process Object” tutorial.
Method of Node.js Readline Module
Let’s now explore all the methods that the readline module provides to interact with the terminal.
1. createInterface()
This method takes process.stdin and process.stdout as arguments to create the instance of the InterfaceConstructor class. This instance is associated with a single input stream for reading and an output stream for writing.
Syntax:
readline.createInterface(input, output);
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
2. rl.question()
This method is used to accept input from the user. This method takes two arguments, a question to ask from the user and a callback function. This callback is used to get the entered value.
Syntax:
rl.question(message, callback);
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.question('What is your name? ', (name) => {
console.log('Hello ' + name);
});
Output:
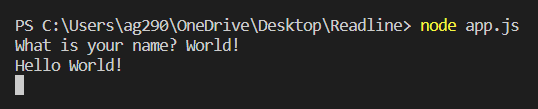
In the output, you can notice that the application keeps asking for input even after entering an input, for getting rid of this we have another method rl.close().
3. rl.close()
This method is used to close the Interface i.e. take control of the input and output stream from the instance of InterfaceConstructor.
Syntax:
rl.close()
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.question('What is your name? ', (name) => {
console.log('Hello ' + name);
rl.close();
});
Output:
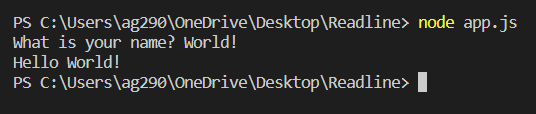
This method does not immediately stop the other events from happening like ‘line’, ‘close’, ‘resume’, etc.
4. rl.resume()
This method resumes the input streams if it is paused but it doesn’t prompt anything.
Syntax:
rl.resume()
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.question('What is your name? ', (name) => {
console.log('Hello ' + name);
rl.close();
rl.resume();
});
Output:
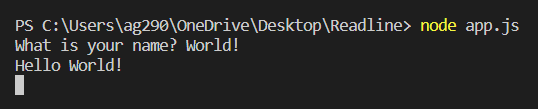
5. rl.setPrompt()
This method is used to set prompts. It takes a string containing any kind of message as an argument.
Syntax:
rl.setPrompt(message)
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.setPrompt('Enter your name: ');
6. rl.prompt()
This method is used to output the value set by rl.setPrompt() in the new line in the console. It resumes the input stream if it is paused and provides a new location for the user to enter the input.
Syntax:
rl.prompt()
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.setPrompt('Enter your name: ');
rl.prompt();
Output:

To get the entered input, we have to use another method rl.on().
7. rl.on()
This method takes an event and a callback. The event can be “line” which will activate when the enter key is pressed, “pause” which is active when input steam is paused, “resume” when it resumes, “SIGINT” when the user presses ctrl + c, and “SIGTSTP” when the user presses ctrl + z.
Syntax:
rl.on(event, callback)
Example:
const readline = require('readline');
const rl = readline.createInterface(process.stdin, process.stdout);
rl.setPrompt('Enter your name: ');
rl.prompt();
rl.on('line', (line) => {
console.log(`Hello ${line}`);
rl.close();
});
Output:
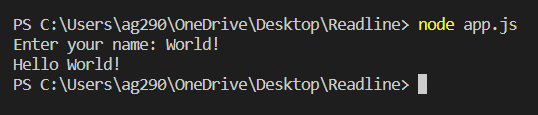
Creating a CLI-Based Calculator App Using Node.js Readline Module
Let’s create a calculator app using the methods we just learned.
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
const calculate = (num1, num2, operator) => {
switch (operator) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
return num1 / num2;
default:
return null;
}
};
rl.question('Enter the first number: ', (firstNumber) => {
const num1 = parseFloat(firstNumber);
rl.question('Enter the second number: ', (secondNumber) => {
const num2 = parseFloat(secondNumber);
rl.question('Enter the operator (+, -, *, /): ', (operator) => {
const result = calculate(num1, num2, operator);
if (result !== null) {
console.log(`${num1} ${operator} ${num2} = ${result}`);
} else {
console.log('Invalid operation!');
}
rl.close();
});
});
});
Here we have created a function that takes two numbers and one operator, then used a switch case to perform the operation based on the operator and returned the result.
Then we used the methods that we learned to prompt the user for the number input and then pass it to our function. Additionally, we use a conditional statement to make sure that an error message is displayed if the result is null.
Output:

Summary
In short, the nodejs readline module is used for reading input and writing output to the console. Using it one can prompt different types of messages in the command line while giving the option to add the input, making it ideal for interacting with the CLI.
Read More: Asynchronous Programming in Node.js – Callback, Promises & Async Await
Reference
https://nodejs.org/api/readline.html