Want to set an alarm reminder on your system while you work? Want to set an alarm if you take a nap in between? No worries, as we have Tkinter with us. Welcome to another page for creating Tkinter applications in Python, and in this tutorial we will learn how to create an alarm clock. Whether you know much about programming or just a little, this tutorial is for everyone. With simple steps, you’ll make your own Python alarm clock quickly.
Steps to Create an Alarm Clock Using Tkinter
We know that Tkinter is a set of tools for Python that lets you build windows and buttons on your computer screen easily. Also, the time library in Python provides functions for working with time-related operations.
We will create a program that creates a GUI alarm clock using tkinter, allowing users to set alarms with custom messages and sounds. Also, the program will display the current time, allowing users to set alarms, play alarm sounds, and trigger alarm messages when the set time matches.
Let’s see how we can build it step by step.
Step 1: Importing Libraries
In this step, we will import the necessary libraries required for the program.
import tkinter as tk
from tkinter import simpledialog, messagebox
import time
import pygame
Step 2: Creating the AlarmClock Class
Then we define a class(AlramClock) that initializes with a constructor for GUI setup. It includes methods for updating the time, setting alarms, playing alarm sounds, and triggering alarms based on set conditions.
class AlarmClock:
def __init__(self, root):
# Constructor code here
def update_time(self):
# Method to update the current time
def set_alarm(self):
# Method to set the alarm time and message
def play_alarm_sound(self):
# Method to play the alarm sound
def trigger_alarm(self):
# Method to trigger the alarm
Step 3: Initializing the GUI and Setting Up the Alarm Clock
Now, we will initialize the GUI window, set up the background image, labels for time display, and buttons for setting the alarm. The update_time() method continuously updates the current time displayed on the GUI.
def __init__(self, root):
# Constructor code here
self.root = root
self.root.title("Alarm Clock")
self.root.geometry("300x200")
self.background_image = tk.PhotoImage(file="path_to_image.png")
self.background_label = tk.Label(self.root, image=self.background_image)
self.current_time_label = tk.Label(self.root, text="", font=("Helvetica", 24, "bold"), fg="white", bg='#007FFF')
self.set_alarm_button = tk.Button(self.root, text="Set Alarm", command=self.set_alarm, bg="green", fg="white")
pygame.init()
pygame.mixer.init()
self.update_time()
Step 4: Updating the Current Time
Next, we will continuously update the current time displayed on the GUI.
def update_time(self):
current_time = time.strftime('%H:%M:%S')
self.current_time_label.config(text=current_time)
if self.alarm_time is not None and not self.alarm_sound_played:
if current_time == self.alarm_time:
self.trigger_alarm()
self.current_time_label.after(1000, self.update_time)
Step 5: Setting the Alarm
Now we create a function called “set_alarm“. This function prompts the user to input the alarm time and message using dialog boxes. Then, it displays a confirmation message using the entered details.
def set_alarm(self):
self.alarm_time = simpledialog.askstring("Set Alarm", "Enter alarm time (HH:MM:SS):")
self.alarm_message = simpledialog.askstring("Set Alarm", "Enter alarm message:")
messagebox.showinfo("Alarm Set", f"Alarm set for {self.alarm_time} with message: {self.alarm_message}")
Step 6: Playing the Alarm Sound
In this step, we create a function that loads and plays the alarm sound when the alarm is triggered.
def play_alarm_sound(self):
pygame.mixer.music.load("path_to_alarm_sound.mp3")
pygame.mixer.music.play()
self.alarm_sound_played = True
Step 7: Triggering the Alarm
Next, we define a function that triggers the alarm when the current time matches the set alarm time.
def trigger_alarm(self):
self.play_alarm_sound()
messagebox.showinfo("Alarm", self.alarm_message)
Step 8: Running the Application
Finally, we check if the script is being run directly, then create an instance of the AlarmClock class to start the application.
if __name__ == "__main__":
root = tk.Tk()
app = AlarmClock(root)
root.mainloop()
Complete Code
import tkinter as tk
from tkinter import simpledialog, messagebox
import time
import pygame
class AlarmClock:
def __init__(self, root):
self.root = root
self.root.title("Alarm Clock")
self.root.geometry("300x200")
# Set background image
self.background_image = tk.PhotoImage(
file="C:\\Users\\HP\\Desktop\\codeforgeek(python)\\alarm clock\\output.png")
self.background_label = tk.Label(self.root, image=self.background_image)
self.background_label.place(relwidth=1, relheight=1)
# Customizing date font to white and bold
self.current_time_label = tk.Label(self.root, text="", font=("Helvetica", 24, "bold"), fg="white", bg='#007FFF')
self.current_time_label.pack(pady=20)
# Customizing set alarm button
self.set_alarm_button = tk.Button(self.root, text="Set Alarm", command=self.set_alarm, bg="green", fg="white")
self.set_alarm_button.pack()
self.alarm_time = None
self.alarm_message = ""
self.alarm_sound_played = False # Flag to track if alarm sound has been played
pygame.init() # Initialize pygame audio
pygame.mixer.init() # Initialize mixer for audio playback
self.update_time()
def update_time(self):
current_time = time.strftime('%H:%M:%S')
self.current_time_label.config(text=current_time)
if self.alarm_time is not None and not self.alarm_sound_played:
if current_time == self.alarm_time:
self.trigger_alarm()
self.current_time_label.after(1000, self.update_time)
def set_alarm(self):
self.alarm_time = simpledialog.askstring("Set Alarm", "Enter alarm time (HH:MM:SS):")
self.alarm_message = simpledialog.askstring("Set Alarm", "Enter alarm message:")
messagebox.showinfo("Alarm Set", f"Alarm set for {self.alarm_time} with message: {self.alarm_message}")
def play_alarm_sound(self):
pygame.mixer.music.load("C:/Users/HP/Desktop/codeforgeek(python)/alarm clock/alarm-clock-short-6402.mp3")
pygame.mixer.music.play() # Play the MP3 audio file
self.alarm_sound_played = True # Update flag to indicate alarm sound has been played
def trigger_alarm(self):
self.play_alarm_sound()
messagebox.showinfo("Alarm", self.alarm_message)
if __name__ == "__main__":
root = tk.Tk()
app = AlarmClock(root)
root.mainloop()
Output:
When we run the code above, an application window opens, displaying the current time along with a “Set Alarm” button.
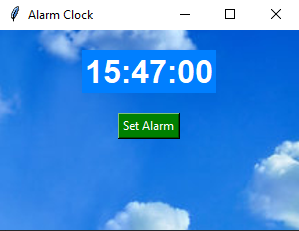
When we click on the “Set Alarm” button, a popup opens to set the time for the alarm.
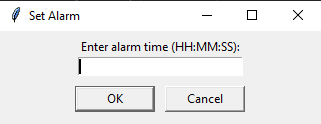
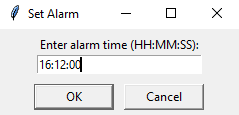
As we press “OK” another popup appears to set the message displayed at the alarm time.
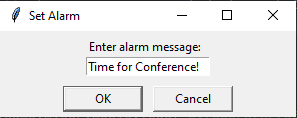
When we press “OK,” a confirmation message pops up stating that the alarm has been set.
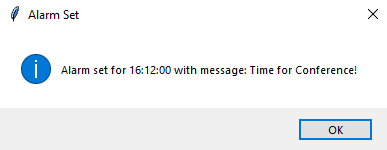
Now, as the clock strikes the alarm time, the alarm window pops up with the message and a beep sound.
Conclusion
And congratulations, as you have learned how to create an Alarm clock application for your system. Now it’s easy for you to set reminders and keep track of time while you are busy with work. We have provided you with the basic skeleton, now try experimenting with different alarm sounds and customization options. We hope the code’s functionality was clear from this content.
If you enjoy reading about Tkinter, here are some more article recommendations to explore: