Checking if a DataFrame is empty using Pandas in Python can be beneficial in various use cases such as before processing or analyzing a DataFrame. This checking for the emptiness of the DataFrame will avoid unexpected errors. Also, sometimes when we iterate through multiple DataFrame we need to handle the empty DataFrame and non-empty DataFrame separately so for that we first have to check whether the DataFrame is empty or not so that we can distinguish them.
In this article, we will look at three ways to check if a Pandas DataFrame is empty so that you can use the one that best suits you. Let’s get started.
Checking if a Pandas DataFrame is Empty in Python
In Pandas, a DataFrame is a two-dimensional tabular data structure that consists of rows and columns. In this article, we want to determine if a DataFrame is empty, so first let’s create an empty DataFrame.
Example:
import pandas as pd
df = pd.DataFrame()
print(df)
Here we used the pd.DataFrame() function to create an empty DataFrame. We have not passed any data to pd.DataFrame() function and thus we get an empty DataFrame, we can see that on printing the DataFrame itself we get that df is an empty DataFrame and does not have any columns or any rows.
Output:

Also Read: Create a Pandas DataFrame from Lists
Following are the methods to check if the DataFrame is empty in Python:
- Using df.empty property
- Using df.shape property
- Using len() function
1. Using df.empty Property
Whether a DataFrame is empty or not, it has a straightforward way to use the empty property. It will return True if the DataFrame is empty else it will return False if the DataFrame is not empty.
Example:
import pandas as pd
df = pd.DataFrame()
print(df.empty)
Here df.empty gives us a Boolean value which essentially tells us whether the DataFrame is empty or not. We have used this property on the DataFrame df which we created above and we got True which is the correct answer as the DataFrame df was an empty DataFrame.
Output:

2. Using df.shape Property
We can check whether the DataFrame is empty or not with the help of df.shape property which will return the tuple of rows and columns of the DataFrame. Hence, df.shape[0] gives us the count of rows and if the count of rows is 0 then DataFrame is empty else the DataFrame is not empty.
Example:
import pandas as pd
df = pd.DataFrame()
print(df.shape[0]==0)
By using the df.shape property of the DataFrame we got a tuple of rows and columns and then compared the value at the 0th index in the Tuple which is the number of rows in the DataFrame with 0. After printing out df.shape[0]==0 statement we got True because the above DataFrame is empty.
Output:

3. Using len() Function
We can check whether the DataFrame is empty or not using the Python built-in len( ) function that gives the length of the Python object. If the length of the DataFrame is 0 then we can say that DataFrame is empty otherwise it may not be an empty DataFrame.
Example:
import pandas as pd
df = pd.DataFrame()
print(len(df)==0)
Here we passed a DataFrame df to the len( ) function it gave us the number of rows in the DataFrame so after that when we compared the number of rows with zero, we again got True because the DataFrame is an empty DataFrame.
Output:

What if the DataFrame Has NaN Values?
Let’s say we have a DataFrame that does have some rows or one row and all the values in the DataFrame are NaN. Will this DataFrame be considered an empty DataFrame or not? Let’s see it with an example.
Example:
import numpy as np
df_nan = pd.DataFrame({'Col1': [np.nan]})
print(df_nan)
Here we created a DataFrame df_nan that has one row and one column and the value in that particular row is NaN.
Output:

Now should DataFrame df_nan be considered an empty DataFrame or not? Fundamentally based on the definition that we set for an empty DataFrame this df_nan DataFrame should not be an empty DataFrame because it does have a row.
Let’s apply the three methods that we saw above to check whether DataFrame df_nan is empty or not.
print(df_nan.empty)
print(df_nan.shape[0]==0)
print(len(df_nan)==0)
Here we applied the df.empty property first then we compared the count of rows with 0 using the df.shape property and then we used the len( ) function to calculate the length of the DataFrame and compared it with 0.
After running, we got False from all three ways which is the correct answer that is this df_nan DataFrame will not be considered as an empty DataFrame.
Output:
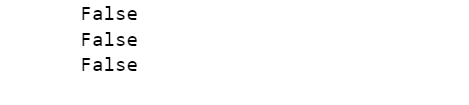
Summary
Checking if a DataFrame is empty with pandas is a valuable practice for handling different scenarios when working with data. It can help ensure that our code behaves as expected, especially when dealing with real-world datasets that may contain missing or empty data. We have used empty, shape, and len() methods in this tutorial with some examples. After reading this we hope you can easily check whether the Pandas DataFrame is empty or not in Python.
Reference
https://stackoverflow.com/questions/19828822/how-to-check-whether-a-pandas-dataframe-is-empty