Updating the value of a row in a Pandas DataFrame is a fundamental data manipulation task that allows us to modify specific elements within a DataFrame’s cells. Whether we need to correct errors, incorporate new information, or adjust data for analysis, this operation is essential for maintaining data accuracy and relevance. By identifying the row that requires an update and applying the necessary changes, we can ensure that our DataFrame reflects the most up-to-date and accurate information for informed decision-making and meaningful insights. Understanding how to efficiently update row values empowers us to keep our data aligned with our analytical objectives.
In this article, we’ll look at 4 methods to update the value of a row in Pandas DataFrame in Python. Let’s get started.
Methods for Updating the Value of a Row in a Pandas DataFrame
For Updating the value of a row in a Pandas DataFrame let’s first create a DataFrame using a CSV file.
Example:
import pandas as pd
data = pd.read_csv("NYC_Jobs.csv")
data.head()
Here we first imported pandas as pd, then created the DataFrame using a CSV file, the name of the CSV file is NYC_Jobs.csv and after that, we printed the head of the DataFrame df.
Output:
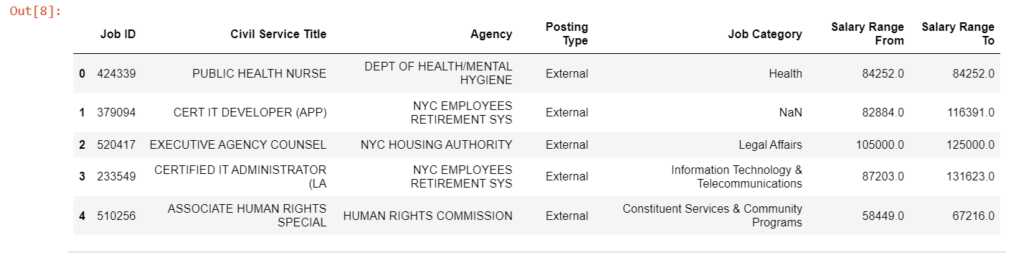
There are four methods through which we can update the value of a row of the above Pandas DataFrame which are as follows:
- Using the fillna() method
- Using the replace() method
- Using the loc() method
- Using the at() method
1. Updating the Value of a Row Using the fillna() Method
The fillna() is a very simple method for updating the value of a row in Pandas DataFrame. It’s all about filling the null values or missing values with a specified value.
Example:
data.fillna('Legal Affairs')
Here we have written data.fillna(‘Legal Affairs’) means we have filled the null values of the DataFrame with the Legal Affairs keyword.
Output:
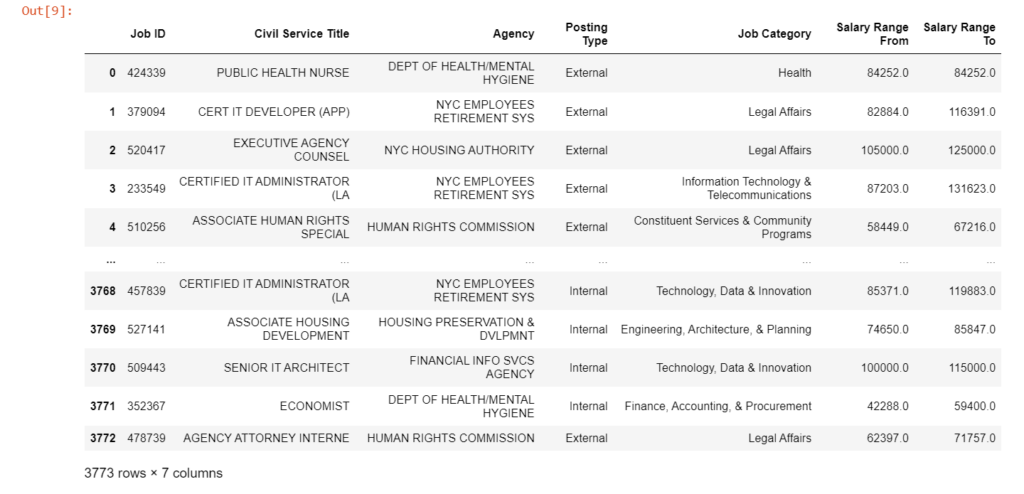
2. Updating the Value of a Row Using the replace() Method
We can use the replace() method for updating the value of a row in the DataFrame. It’s a very straightforward method that is used to replace some value with another value and it only works with exact matches within the DataFrame. Here we need not provide any index or label value to it.
Example:
data.replace('NYC HOUSING AUTHORITY','NYC AUTHORITY FOR HOUSING')
Here we have written data.replace(‘NYC HOUSING AUTHORITY’,’ NYC AUTHORITY FOR HOUSING’) means wherever in the DataFrame there is a keyword NYC HOUSING AUTHORITY, it will get replaced with NYC AUTHORITY FOR HOUSING.
Output:
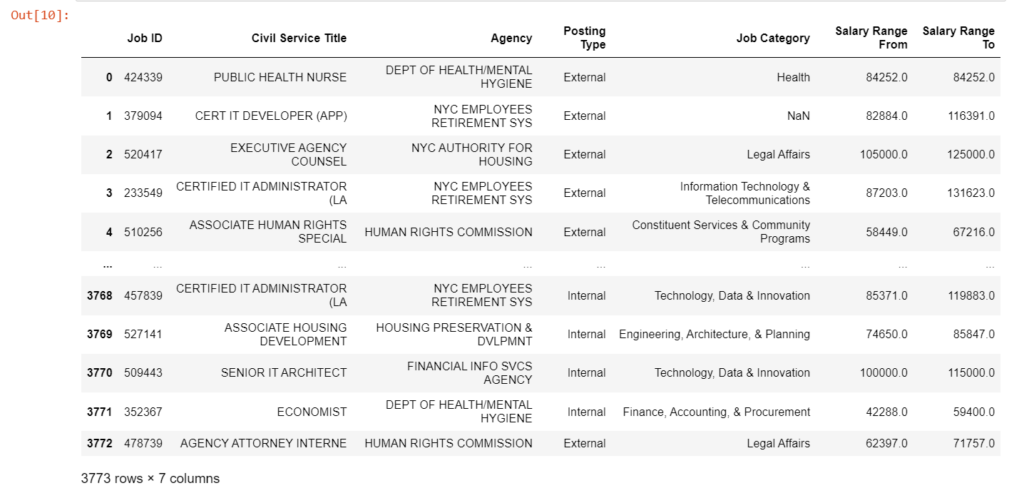
3. Updating the Value of a Row Using the loc() Method
We can use the loc() method for updating the row with respect to columns. Here we will provide the labels of the columns.
Example 1:
data.loc[data['Posting Type'] =='External', "Posting Type"]=1
Here we have written data.loc[data[‘Posting Type’] ==’External’, “Posting Type”]=1 means that wherever in the Posting Type column there is a keyword External it will get updated to 1.
Output:
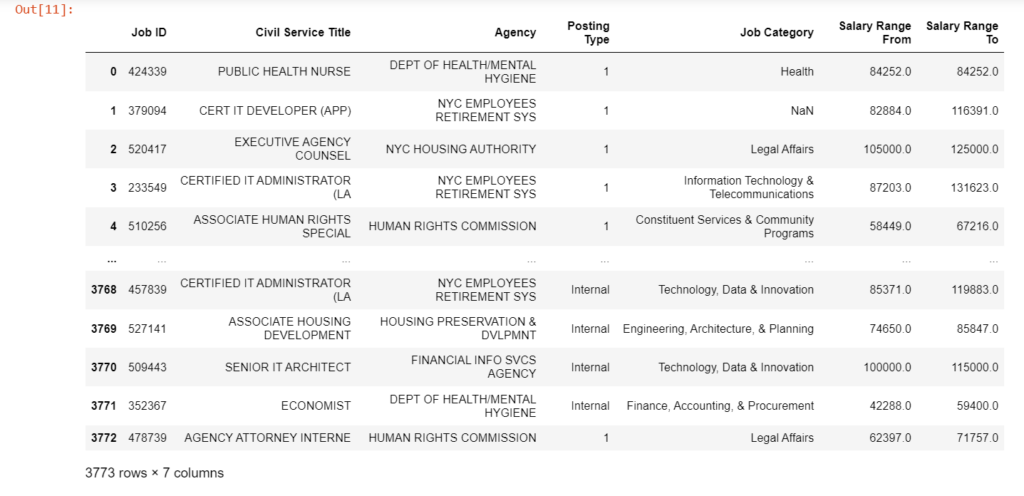
Example 2:
data.loc[3, 'Job Category']= "ITT"
Here we have written data.loc[3, ‘Job Category’]= “ITT” means that at index 3 in the Job Category column, the previous keyword will get updated to ITT.
Output:
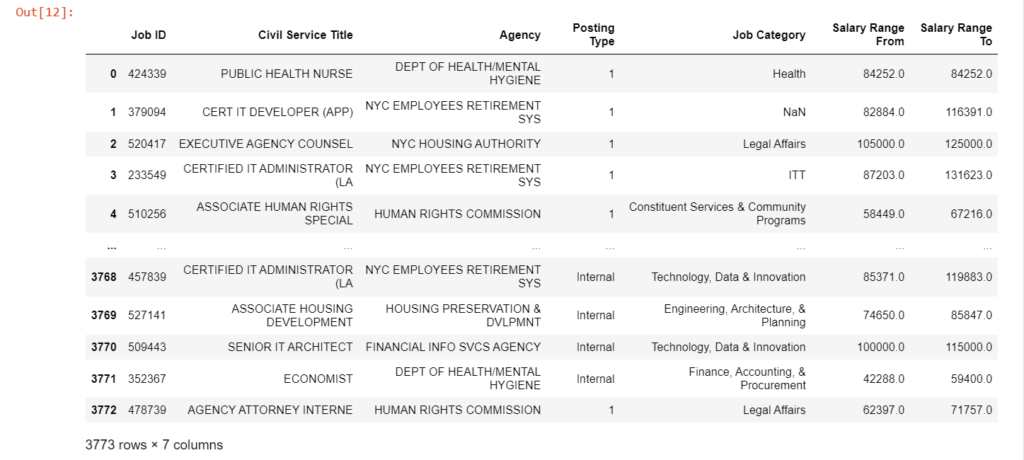
4. Updating the Value of a Row Using the at() Method
We can also use the at() method for updating the value of a row in the DataFrame. Here with respect to the column, we can update the value of one row at a time.
Example:
data.at[data['Job Category'] =='Health', "Job Category"]='Health Dept.'
Here we have written data.at[data[‘Job Category’] ==’Health’, “Job Category”]=’Health Dept.’ means where the value in the Job Category column is equal to “Health” will get changed with a new value “Health Dept.”.
Output:
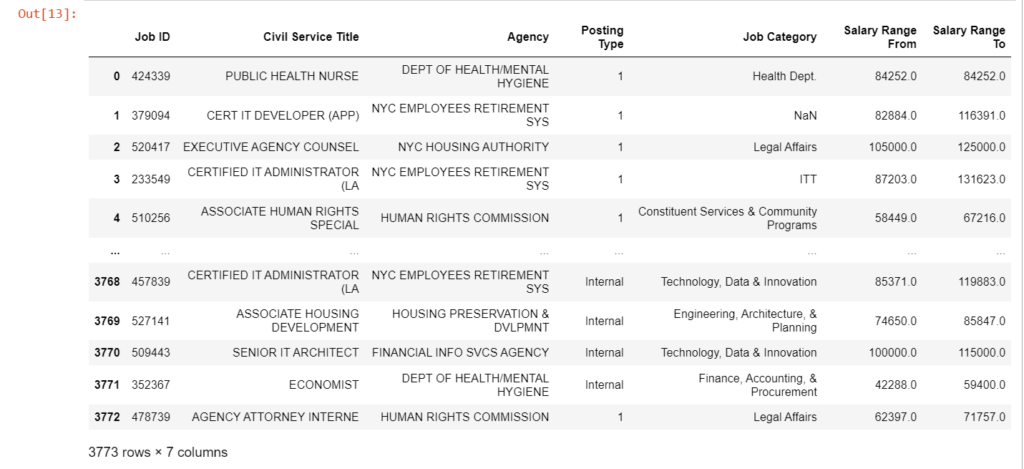
Summary
In this tutorial, we have discussed four methods which are fillna(), replace(), loc(), and at() to update the value of a row in Pandas DataFrame with examples. After reading this tutorial, we hope you can easily update the value of a row in Pandas DataFrame in Python.