Welcome, coders! In our NumPy series, we have brought a useful function for you: numpy.exp2, which helps us compute the powers of two in Python. We will discuss it in detail, so keep following along.
Why Compute Powers of Two?
“Computing Powers of Two” is like figuring out how many times you can double a number. You start with 1, then double it to get 2, double that to get 4, and so on.
This is important in computers because they work with 0s and 1s, which we call binary. Let’s say you have a computer with 4 slots for 0s and 1s, called bits. Each bit can either be 0 or 1, just like flipping a coin.
Now, if you have 4 of these bits, you can make different combinations of 0s and 1s. How many? Well, each bit gives you two choices (0 or 1), and you multiply those choices together for each bit. So, it’s like 2 multiplied by itself 4 times: 2^4=16.
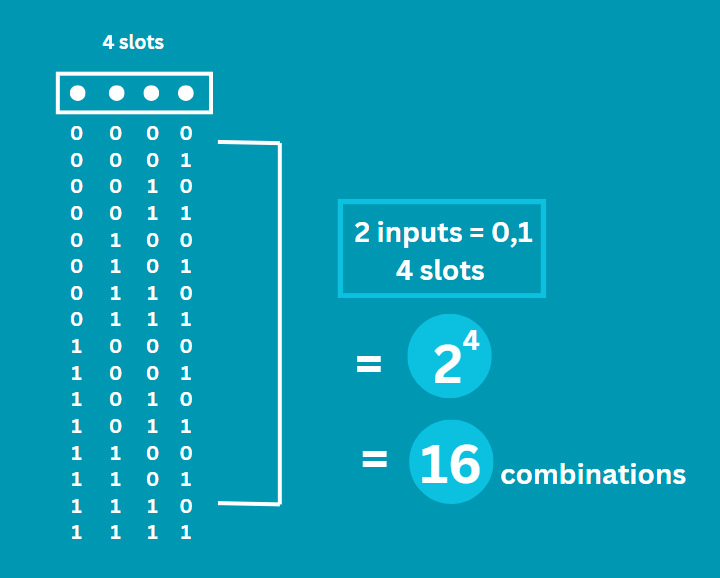
Knowing the powers of two helps us understand how much information computers can handle and how they store data efficiently. It is the foundation of computer memory and storage.
numpy.exp2() in Python
The NumPy library offers us a numpy.exp2() function, which allows us to easily compute powers of two. Let’s discuss its syntax and parameters.
Syntax:
The basic syntax of the given function is:
numpy.exp2(x, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature, extobj])
Here is what these parameters mean:
- x: It’s the input values. It can be both an array or a single value.
- out: This is where the result is stored. If you provide it, it must match the inputs. If not provided, a new array is created.
- where: It’s a condition applied to the input. Where the condition is true, the result will be set. Otherwise, the original value is kept.
- dtype: This specifies the type of the output array. If you don’t give it, the type is guessed from the input.
- casting: It controls what kind of data casting is allowed. The default is to keep it the same.
- order: This determines how the array is laid out in memory. ‘C’ means row-major (last index varies fastest), ‘F’ means column-major (first index varies fastest), and ‘A’ (default) means any order.
Working:
- The numpy.exp2() function returns 2 raised to the power of the elements of the input array.
- If the input is scalar, the result will be scalar too.
- The function supports broadcasting, meaning it can operate on arrays of different shapes and sizes.
- You can control the data type of the output array using the dtype parameter.
- The where parameter allows you to conditionally operate.
Using numpy.exp2() in Python
It will never be so clear until we see some real coding examples. Let’s see how we can use this function in Python with different inputs like scalar, 1D array, 2D array, where parameter, etc.
Example 1: Here we will compute the power of two for a scalar value.
import numpy as np
result = np.exp2(4)
print("2 raised to the power of 4:", result)
Output:
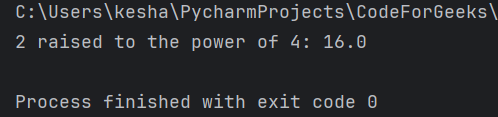
Example 2: When we work with arrays, the function returns powers of two for each element in an array.
import numpy as np
input_array = np.array([0, 3, 5, 7])
result = np.exp2(input_array)
print("Powers of two:", result)
Output:
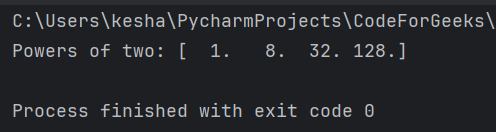
Example 3: We can apply conditions to computations using the ‘where‘ parameter.
import numpy as np
input_array = np.array([0, 1, 2, 3])
condition = input_array < 2
result_array = np.exp2(input_array, where=condition)
print("Powers of two with condition:", result_array)
Output:
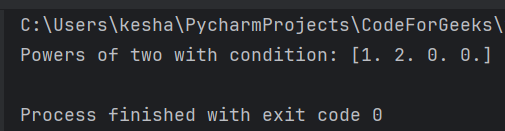
Example 4: Let’s see how we can use broadcasting with this function.
import numpy as np
scalar_value = 3
array_1d = np.array([0, 2, 4, 6])
array_2d = np.array([[1, 3], [5, 7]])
result_1d = np.exp2(scalar_value + array_1d)
print("Broadcasting scalar with 1D array:")
print(result_1d)
result_2d = np.exp2(scalar_value + array_2d)
print("\nBroadcasting scalar with 2D array:")
print(result_2d)
Output:
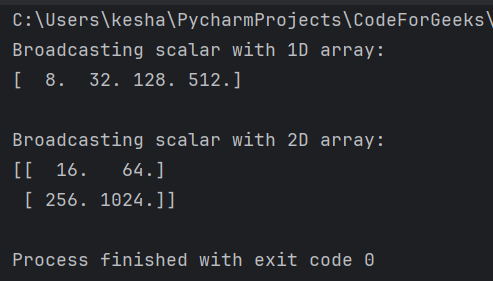
Conclusion
And that’s it for this one! We have learned about new things such as the importance of powers of two in computer memory organization. We have also seen the method in NumPy to calculate powers of two and discussed its syntax, parameters, and use cases.
If you enjoyed this one, don’t forget to check out:
Reference
https://numpy.org/doc/stable/reference/generated/numpy.exp2.html