The find() method returns the first element that passes the given test function. This function does not execute for null elements and returns undefined if elements fail to pass the test case. In this tutorial, along with the find() method, we will also learn about many variants of this function that help us find the index, last index, etc.
Syntax of find() Method
array.find(function(element, index, array), thisArg)
Here is the meaning of each parameter in the above syntax:
- array: An array whose elements are to be used.
- function: A test function.
- element: The current element that is being processed.
- index: The index of the current element
- array: The array find was called upon.
- thisArg (optional): A value to use as this when executing the callback.
Return:
It returns the value of the first element to pass the specified test function and if elements are unable to pass the test case, it returns undefined.
Working of find() Method
The find() method is iterative. It calls the provided test function once for each element in the array, in ascending order, until it returns a true value. The find() returns that element and stops iterating. If it does not return a true value, it returns undefined.
To use the given method, we need to first define a test function, then the find() method would take every element in the array and check it against that test function, returning the first element that fulfils the test. The search always takes place from left to right.
Example of find() Method
Let’s better understand this with code. Let us provide the test case as a number greater than 5 and then the code will look like:
let array = [5, 2, 7, 4, 8];
function check(num) {
return num > 5;
}
console.log(array.find(check));
Here we created an array and a test function, then passed that function into the array.find() method. Since the first element on iteration within the array that satisfies the test is 7, it returns 7.

Click here to understand the concept and usage of Array in JavaScript.
Other Array Find Methods
Now we will discuss different types of array find methods. These are not subtypes but work similarly and lie in the same category.
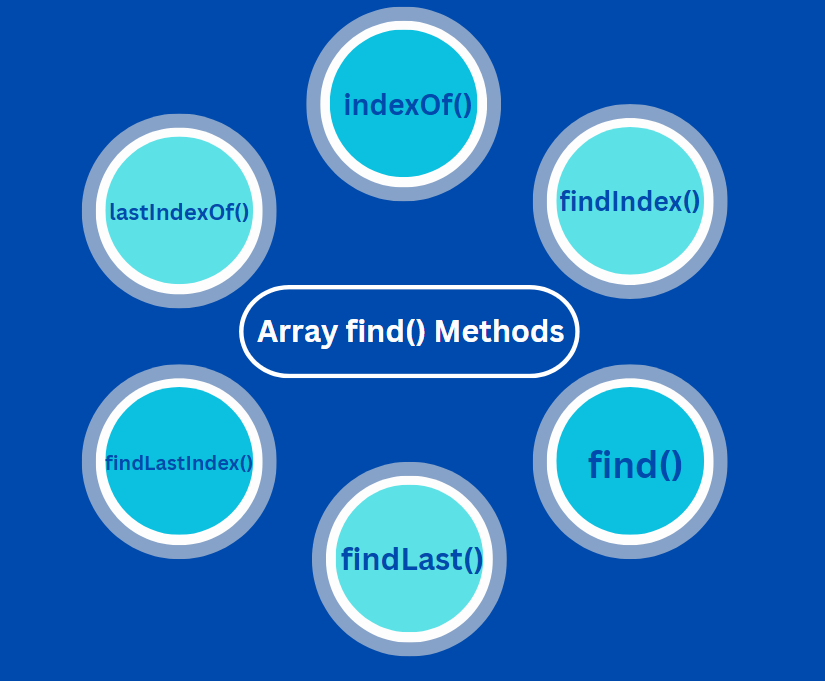
1. findLast() Method
This method works similarly to the find() method but this returns the value of the last element in the array that passes the test.
let array = [5, 2, 7, 4, 8];
function check(num) {
return num > 5;
}
console.log(array.findLast(check));
Here the element seen from last which passes the condition of our function is 8.

2. findIndex() Method
This method returns the index of the first element in an array that passes the test function. It works similarly to the find() method, the only difference is that this returns the index of that element.
let array = [5, 2, 7, 4, 8];
function check(num) {
return num > 5;
}
console.log(array.findIndex(check));
Here we know that the first element that passes the test case is 7, so its index is printed, which is 2.

3. findLastIndex() Method
This method returns the index of the last element in an array that passes the given test function.
let array = [5, 2, 7, 4, 8];
function check(num) {
return num > 5;
}
console.log(array.findLastIndex(check));
Here we know that the last element that passes the test case is 8, so its index is printed, which is 4.

4. indexOf() Method
This method returns the value of the index or position of the specified element.
const planets = ["Jupiter", "Uranus", "Mercury", "Pluto" , "Earth"];
let index = planets.indexOf("Pluto");
let index2 = planets.indexOf("Venus");
console.log("The position of Pluto starting from index 0 is ", index);
console.log("The position of Venus ", index2);
In the given code, we have 5 elements in the array. Now, if we find the index of “Pluto,” it will turn out to be 3, as it is the 4th element, and index counting starts from 0. As for Venus, since it’s not an element in the array, the method returns -1.

5. lastIndexOf() Method
This method returns the index of the element which ranks at the last.
const planets = ["Jupiter", "Pluto", "Mercury", "Pluto" , "Earth"];
let index = planets.indexOf("Pluto");
let index_last = planets.lastIndexOf("Pluto");
console.log("The position first Pluto is ",index);
console.log("The position last Pluto is ",index_last);
If we see in the given code, there are two elements named Pluto, the index of the first coming element is printed by indexOf() which is 1 and the index of the element at the last is printed by lastIndexOf() which is 3.

Conclusion
All of these methods that we have used are very important, as they make us find elements and their positions in an array. The element or the index of the element can be searched from both front and back with a test function to get elements based on our needs.
If you want to know how to remove duplicate values from an array in JavaScript then click here.