In JavaScript, Array is a variable that can store multiple types of data together, an array can have a number, a string, a boolean, object, etc. There is no fixed data type and length of an array in JavaScript which makes it super flexible and easy to use to combine data together.
Array in other Programming Languages
You often know an Array to store multiple entities of the same data type in a group. The entities of an array can be any one of the data types such as int, float, char, boolean, etc, it means if an Array is declared to store integer then it can’t store char, boolean, or any other data types. In JavaScript the array is treated differently, it is considered an object that can store any type of value.
Structure of an Array
An array contains elements inside a square bracket([]) separated by commas(,).
["element1", "element2", "element3", ...];
Declaration of a Javascript Array
An array can be declared directly or by using the built-in Array class of JavaScript.
Using Array Literals
The array can be created directly by assigning an empty square bracket to a variable.
Syntax:
const arr = [];
Here the variable ‘arr’ is considered an array.
Using Array Class
An array can be created as an instance of the Array class.
Syntax:
const arr = new Array();
Here the instance ‘arr’ is an array in JavaScript.
Directly method is preferred over the creating an instance of the Array class because if you initiate an array using the Array class with a single number it takes it as an argument to create that much number of undefined elements inside the array object.
Below is the code to declare an array using the Array class:
Example of Javascript Arrays
const arr = new Array(4);
console.log(arr);
Output:
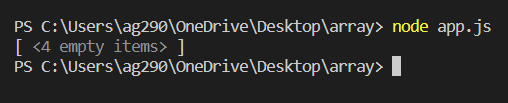
Here see we get 4 undefined elements whereas our intention is to have the number 4 as an element of the array.
Accessing Array Element
The first element of an array has the index number ‘0’, which means the array starts counting the index number from ‘0’ not ‘1’ for example if you want to access the third element of an array you have to use the index number ‘2’.
Syntax:
arrayName[i];
where i is the index number of the element to which you want to Access.
Example:
const arr = ["element1", "element2", "element3"];
const element1 = arr[0];
console.log(element1);
Output:

Assigning Array Element
Below is the syntax for assigning elements to an array.
Syntax:
arr[i] = "new element";
where i is the index location of an array to which you want to assign the element.
Example:
const arr = ["element1", "element2", "element3"];
arr[3] = "element4";
console.log(arr);
Output:

Changing Javascript Array Element
Assigning an element to a location where an element already exists will change it with the new one.
Example:
const arr = ["element1", "element2", "element3"];
arr[0] = "new element";
console.log(arr);
Output:

Javascript Array are Objects
In JavaScript, an array is an object, the difference is the array element can be accessed using its index, whereas an object element can be accessed using the key value.
We can check the data type of an array using the typeof method.
Example:
const arr = [];
console.log(typeof arr);
Output:

Looping Javascript Array Elements
All type of loops is performed on the array.
Example:
const arr = ["element1", "element2", "element3"];
for (element of arr) {
console.log(element);
};
Output:
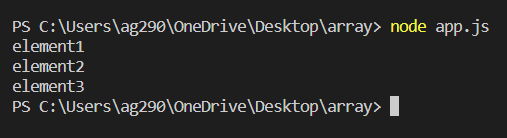
Adding Array Element
A new element can be added to an array using the push() method.
Example:
const arr = ["element1", "element2", "element3"];
arr.push("element4");
console.log(arr);
Output:
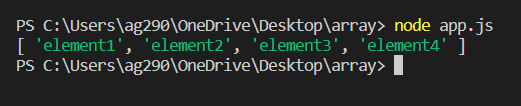
Removing Array Element
An element can be removed from an array using the pop() method.
Example:
const arr = ["element1", "element2", "element3"];
arr.pop("element3");
console.log(arr);
Output:

Length of an Array
The length of an array is the number of element present in it.
Unlike the index number, it starts counting from ‘1’ so if there is a 3 element it returns ‘3’ whereas the index number of the 3rd element is ‘2’.
Example:
const arr = ["element1", "element2", "element3"];
console.log(arr.length);
Output:

Summary
An array is a special type of object in JavaScript that can store multiple types of values, the values in an array are called its element and the elements of an array can be accessed using its index number, the index number of an array starts with ‘0’. We can add elements to an array, remove elements, change elements, find the length of the array, etc. Hope this tutorial helps you to understand the array in JavaScript.
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array