Whenever we talk about backend development there is one term that is mainly used by developers of almost every level, from beginner to expert. That term is ExpressJS. This tutorial will introduce you to the basics of ExpressJS and we are going to do several interesting things with it.
What is ExpressJS?
It is a Framework based on NodeJS that allows developers to build highly scalable and robust application programming interfaces (API). It is most popular because of its features which make it most powerful too.
Also read: NodeJS: Installation, Setup, and Creating a Hello World Application in NodeJS
ExpressJS Features
- It allows you to code middleware that can act as a bridge between HTTP requests so that you can respond to them.
- It can be used for dynamic routing between URLs. The thing that a specific URL will redirect to a specific page is called routing.
- Dynamically rendering HTML pages and manipulating them in real-time.
- It is one of the most used backend frameworks and that is because of its features which make it highly powerful.
Installing ExpressJS
For the installation process, it is required to have NodeJS installed on your computer.
- Open your terminal and run the below command:
npm init -y
A file name package.json will be added to your folder.
- Then in the same terminal run the below command:
npm i express
this will install ExpressJS as a module inside your project.
Hello World Program in ExpressJS
Let’s start our backend development journey by writing a simple hello world program.
const express = require('express')
const app = express()
app.get('/', (req, res) => {
res.send('Home Page')
})
app.listen(3000, () => {
console.log('listening on port 3000')
})
In the above code, we have imported the Express module inside our project by using the require() method.
- Then created an instance of Express and saved it into the app variable. This is the variable on which we are going to work mostly.
- After that, created a get request on “/” (home route) which will send something. After that, there is a callback function that has request and response arguments these are required and most important.
- The next line simply sent some text.
- After this, we used listen() function to start our server on port number 3000.
Output:
Go to http://localhost:3000/ and you will see the following output.
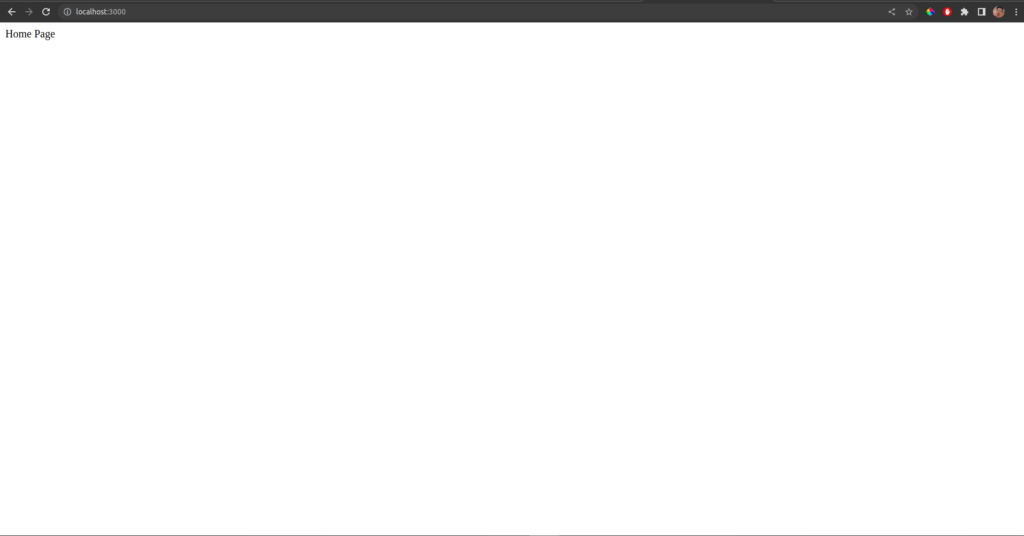
Request & Response Object
Request and Response are the two most common and most important objects for building a connection between an API and a client(i.e. user).
app.get('/', (<strong>req</strong>, <strong>res</strong>) => {
res.send('Home Page')
})
- Req: – It is the object which represents the HTTP request including its parameters, complete string, body, and headers.
- Res: – It is the object which represents the response that will be sent from API to the client.
GET and POST Method
These are the two most common methods used in backend development. Let’s discuss them.
- GET: – This method is used on those routes where nothing is expected from the user, all he or she will do is navigate to a route of API and some data will be thrown back to the user. For example in the above code when a user navigated to “/” he or she was directly getting the response of hello world and there is no way for the user to send data to API.
- POST: – This method is used when the user has to pass dynamic data to an API and the API can work on that data. The data sent through the POST request is stored in the req object.
Sending Different Files
We can also send different types of files and data from our API. In this example, we will be sending static pages and JSON data
Sending Static Pages
To demonstrate the process of sending the static file it is required to have a simple HTML file which we have already created, you can download it from the given link and place it in your project route directory.
index.html
Download the file from here and place it in your project root directory.
app.js
const path = require('path')
app.get('/front-end', (req, res) => {
res.sendFile(path.join(__dirname + '/index.html'))
})
In the above code, we first imported the file system module, then created a GET route on /front-end and called the get method on it, then used the sendFile() method and passed the path of a file we want to send.
Now Express will send that file instead of normal text. The output can be seen below.
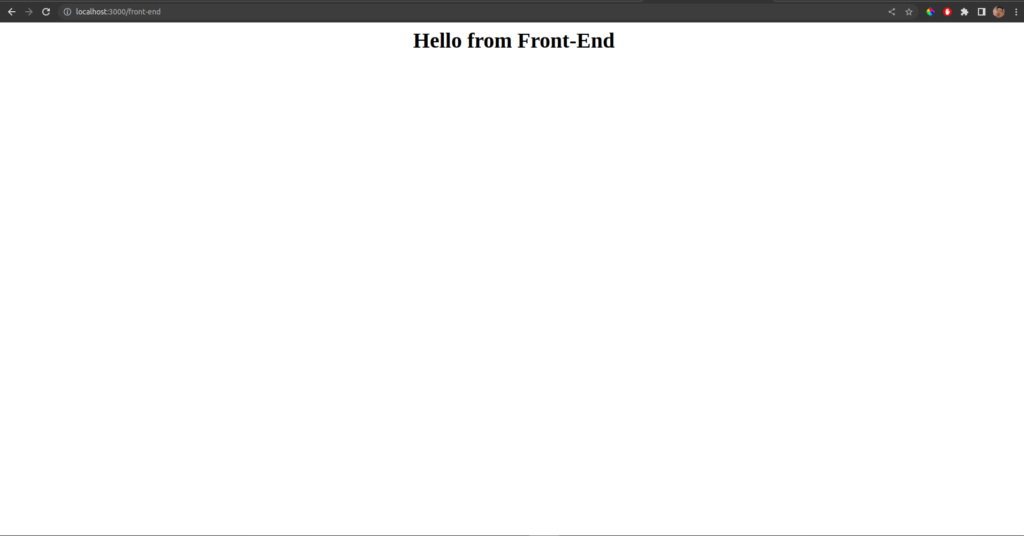
Sending JSON files
We can also send JSON data from our API as a response, which is what we will mostly do in the case of backend development. Backend development is just applying logic behind the scene and sending JSON data so that the frontend application can integrate with it.
app.get('/jsonResponse', (req, res) => {
res.json({
status: 'success',
message: 'This request was successfully processed'
})
})
In this case, I sent JSON using the JSON function and it is mandatory to pass an object instead of a simple string.
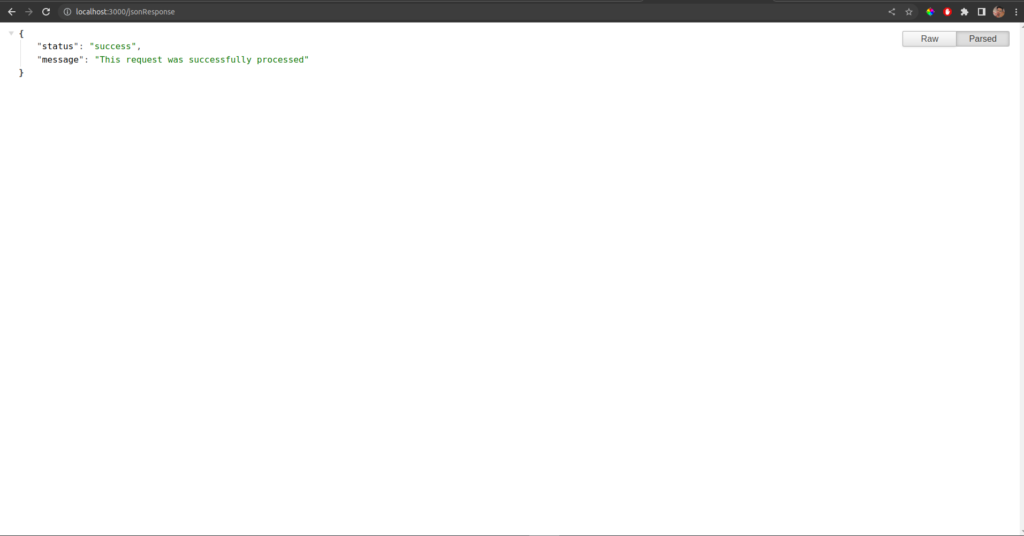
Complete Code: – https://github.com/sushant102004/ExpressJSTutorial
Summary
Express.js is a powerful and versatile framework for building APIs in Node.js. Its easy-to-use routing, middleware, and dynamic rendering capabilities make it a popular choice among developers.
The request and response objects make it simple to handle incoming HTTP requests and send responses, while the GET and POST methods provide flexibility in handling different types of requests.
The ability to send various types of files, such as HTML and JSON, further enhances the functionality of Express.js. Overall, Express.js is a great choice for any developer looking to build a scalable and robust API.