In this article, we are going to see how we can get the full URL in Express.js. Express.js is a popular framework for building web applications in Node.js. It provides a straightforward way to handle HTTP requests and responses.
In web development, there are many situations where you might need to access and work with the full URL of a request. Whether you’re building a RESTful API, a web server, or just want to log the URLs your users are visiting, understanding how to get the full URL in Express can be quite handy.
Understanding Full URL
Before we dive into the technical details, let’s clarify what we mean by the “Full URL”. URL stands for Uniform Resource Locator. The full URL is a combination of several components:
1. Protocol: This can be either HTTP or HTTPS. The protocol defines the rules for how data is exchanged between your browser and the web server. HTTP is unencrypted, while HTTPS is secure and encrypted.
2. Host: The host is the server’s name and port. For example, in the URL “https://www.example.com”, “www.example.com” is the host, and “8080” is the port. The port is usually omitted for HTTP (80) and HTTPS (443) because they are the default ports.
3. Path: The path represents the specific resource or endpoint on the server. For example, in the URL “https://www.example.com/products/trees“, “/products/trees” is the path.
4. Query Parameters: These are additional data sent along with the URL, often used for filtering or customizing requests. They appear after a question mark, like this: “?color=red&size=10”.
In Express, you can access all these components to construct the full URL for the current request.
Using Express.js to Get the Full URL
Below is a step-by-step guide to get the full URL using Express in Node.js.
Step 1: Install Express
First of all, you need to install the Express framework. You can do this by running the following command in your project directory (assuming you have Node.js and npm installed):
npm install express
This command installs the Express package and adds it to your project’s dependencies.
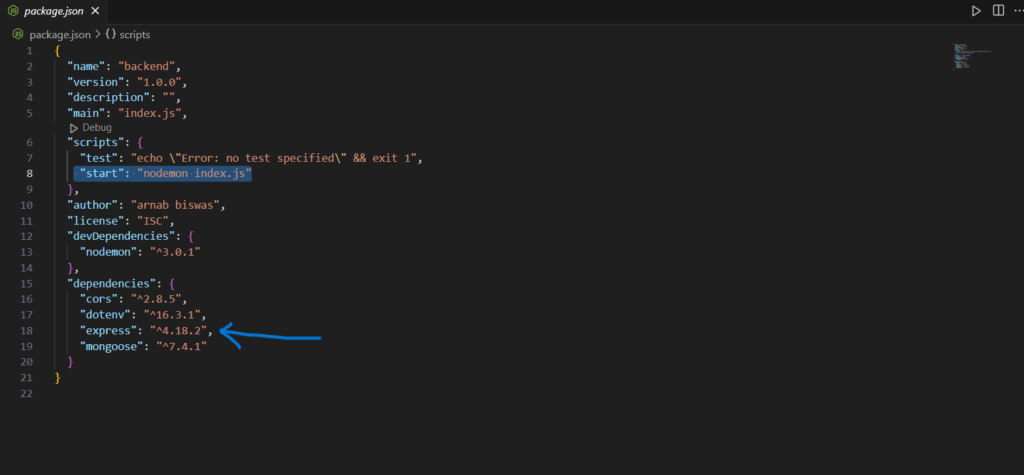
Step 2: Import Express
After installing Express, you need to import it into your JavaScript file.
const express = require('express');
This line imports the Express module and makes it available for use in your application.
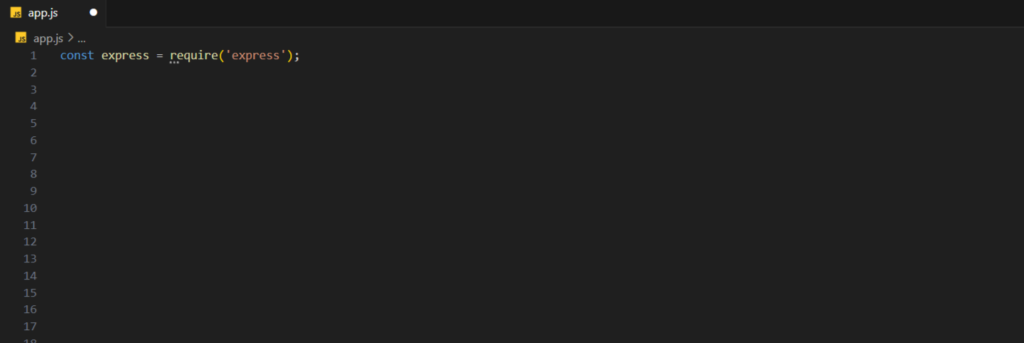
Step 3: Create an Express Application
Next, you need to create an instance of the Express application:
const app = express();
This line initializes your Express application and allows you to define routes and handle HTTP requests.
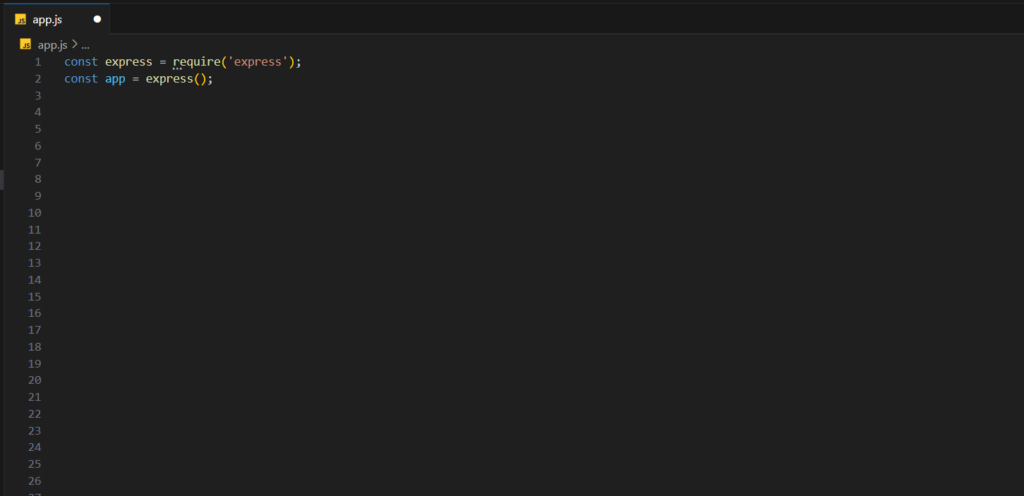
Step 4: Define a Route
In your code, you have to define a route that handles HTTP GET requests to the root URL (“/”). You can do this using the app.get method:
app.get('/', (req, res) => {
// Route handling code goes here
});
In the callback function, (req,res) are the request and response objects, respectively.
Step 5: Retrieve the Full URL
Inside the route handler, you want to retrieve the full URL of the incoming request. You can do this by using various properties of the req object. Here’s how you construct the full URL:
const fullUrl = `${req.protocol}`+'://'+`${req.get('host')}${req.originalUrl}`;
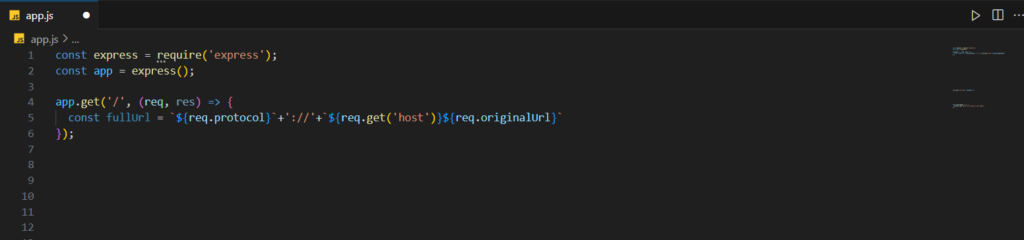
Here,
- req.protocol: This property holds the protocol used for the request, either “http” or “https”.
- req.get(‘host’): It returns the host, which includes the hostname and, if applicable, the port number.
- req.originalUrl: This property contains the original URL requested, including the path and query parameters.
Step 6: Send the Response
Now, you have to send a response back to the client with the full URL:
res.send(`Full URL: ${fullUrl}`);
This line sends a simple text response to the client, displaying the full URL.
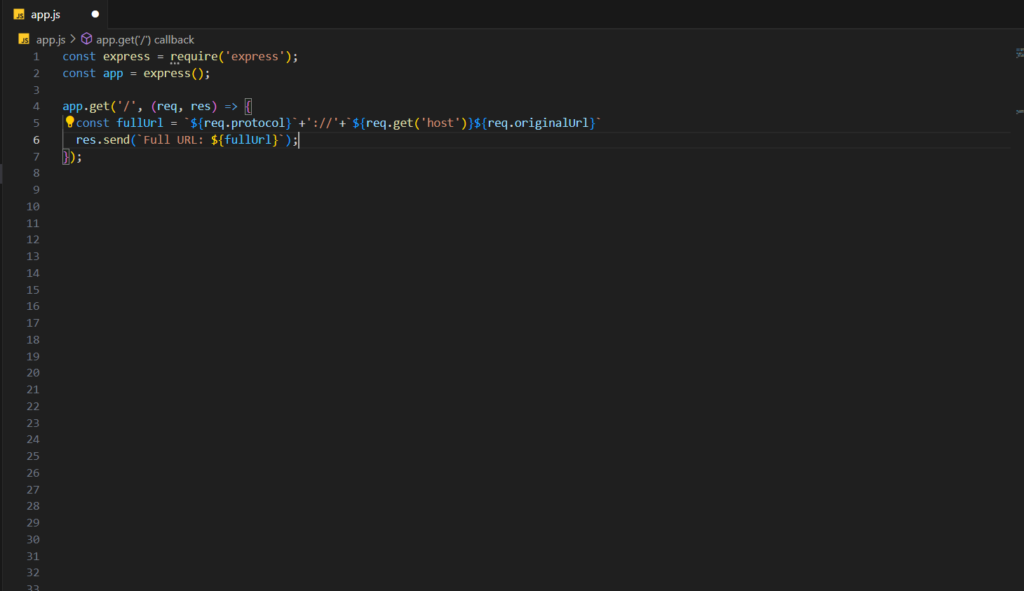
Step 7: Start the Server
Lastly, you have to write the below code to start the Express application and listen for incoming requests on a specified port (in this case, port 5000):
app.listen(5000, () => {
console.log('Server is running on port 5000');
});
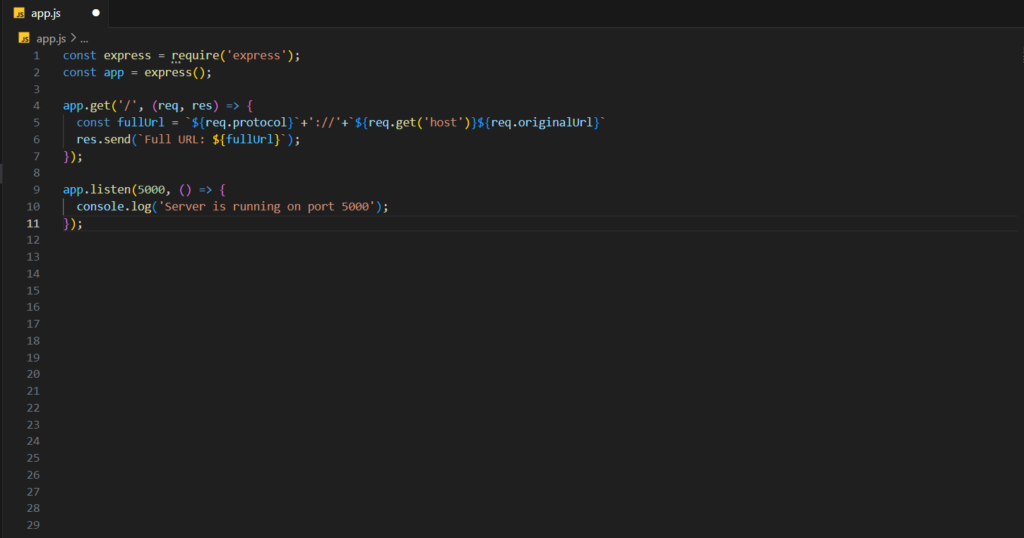
Complete Code
const express = require('express');
const app = express();
app.get('/', (req, res) => {
const fullUrl = `${req.protocol}`+'://'+`${req.get('host')}${req.originalUrl}`;
res.send(`Full URL: ${fullUrl}`);
});
app.listen(5000, () => {
console.log('Server is running on port 5000');
});
Output:
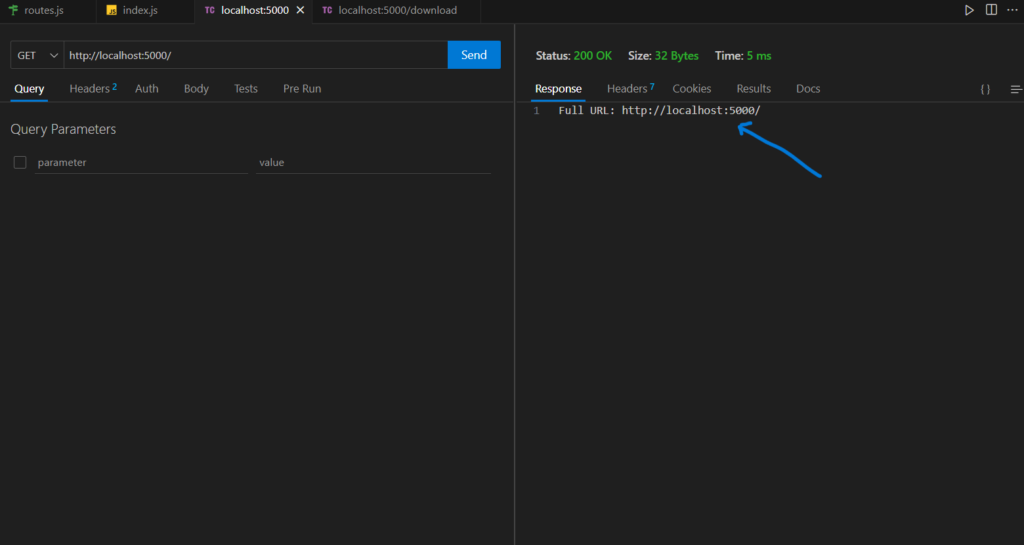
Conclusion
Now we have reached to the end of this article. Hope it has elaborated on Getting the Full URL in Express.js. In Express.js, you can very easily get the full URL of an incoming request by using the req object’s properties. The full URL is a combination of the protocol, host, path, and query parameters. For more such articles on Node and Express follow https://codeforgeek.com/.
Reference
https://stackoverflow.com/questions/10183291/how-to-get-the-full-url-in-express