JSON stands for JavaScript Object Notation. It is used to structure data based on JavaScript object syntax, which is easy for humans to read/write and machines to parse.
JSON is lightweight, making it perfect for fast data transmission over HTTP. It is compatible with almost any modern programming language such as C, PHP, Python, Java, etc, and hence widely used for cross-platform data exchanges. In this tutorial, we will learn how to send JSON responses using the express framework.
Express res.send() Method
In an Express application, when handling routes we generally use res.send() to send a response back to the client making an API call to our Node server.
Example of res.send():
const express = require('express');
const app = express();
app.get('/api', (req, res) => {
res.send('Content to send');
});
app.listen(3000);
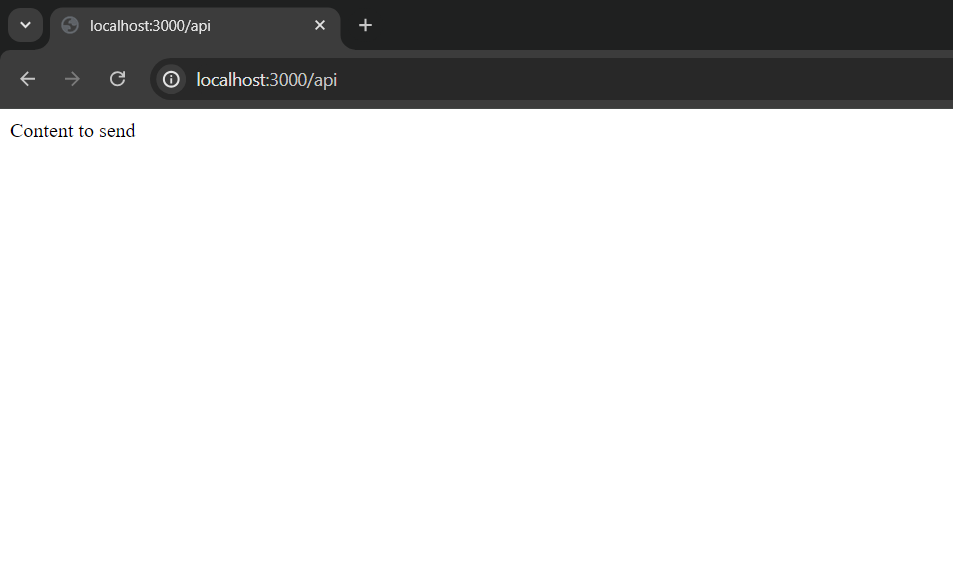
But in many cases, our res.send() is not proven to be the best method for sending JSON. With res.send() method, for sending a JavaScript object as JSON we manually need to parse it into JSON, we also have to set the Content-Type header to application/json and it also does not support JSONP (JSON with Padding). For all these problems, express provides a dedicated method res.json().
Express res.json() Method for Sending JSON Response
We can send a JSON response by using res.json() method. It accepts an object, converts it into JSON, sets the Content-Type header to application/json and then sends it.
Syntax:
res.json(data);
data can be an object or a value you want to convert into JSON and send as a response to the clients.
Return:
The res.json() method returns a promise to get the resolved JSON data.
Example of res.json():
const express = require('express');
const app = express();
app.get('/api', (req, res) => {
res.json({
message: 'Content to send'
});
});
app.listen(3000);
If you are new to express and can’t understand the above code then refer – Express Tutorial for Beginners
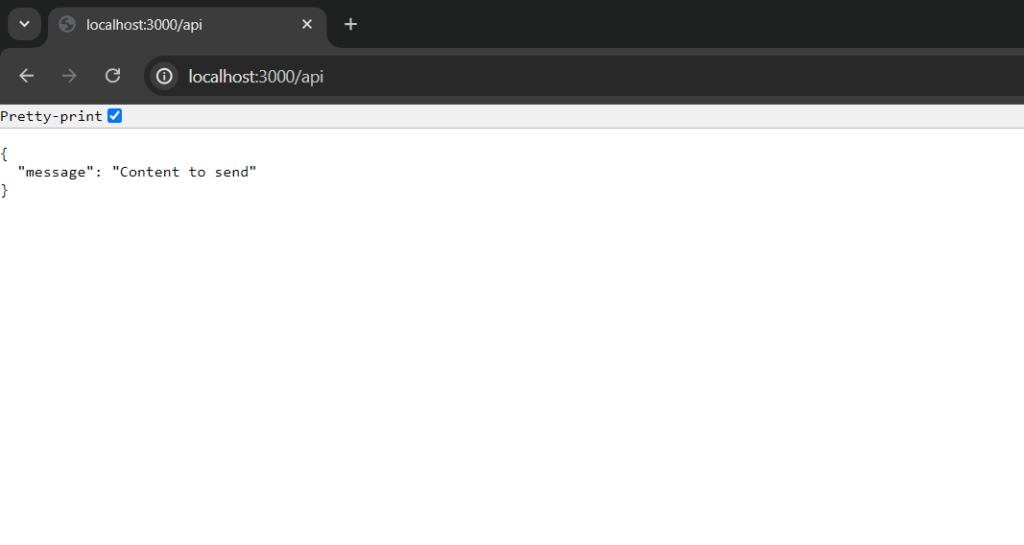
Frequently Asked Questions (FAQs)
What is JSON full form?
JSON stands for JavaScript Object Notation, used to structure data based on JavaScript object syntax.
How to send JSON data using Express?
You can use the Express res.json() method to send JSON data in Node.js. You just have to call the res.json() method and pass an object or a value, this method will automatically transform it into JSON, set the Content-Type header and send it.
What is the difference between send() and json() in Express?
The main difference between these two is that the send() method sets the Content-Type as “text/html” whereas the json() method sets it as “application/json” and can transform the data into a JSON string before sending it as a response object.
How to send a variable in JSON?
To send a variable in JSON, encapsulate it as a value within a JS object and pass this object as an argument to the res.json() method.
How to send array data in JSON?
To send array data using the res.json() method, you should wrap the array within a JavaScript object using a key that holds the array as its value.
How to write a JSON string?
You can create a JavaScript object and pass it as an argument to JSON.stringify() function to convert it into JSON string.
Conclusion
To send data as a response to the client, we use res.send() method and to send static files we use the res.sendFile() method. In the same way, to send JSON, Express.js has a method res.json() that takes an object or a value, converts it into JSON and sends it as a response. With this method, you don’t even have to set the Content-Type header, it will automatically set it to “application/json”.
If you are new to Node.js:
- What is Node.js?
- Asynchronous Programming in Node.js – Callback, Promises & Async/Await
- How to send an HTML File in Node.js?
Reference
https://stackoverflow.com/questions/19696240/proper-way-to-return-json-using-node-or-express