Dropping columns from Pandas DataFrame in Python is a common operation in data analysis and data preprocessing tasks. There are several use cases where we might want to drop specific columns from DataFrame. For instance, whenever we are working with large datasets, we might get some columns that are not needed in our analysis or contain useless information so dropping these columns gives us the result in the reduction of memory usage which will also simplify the data processing. Also sometimes our data contain missing values in some columns so we need to drop those columns if they are not crucial to our analysis.
In this article, we’ll look at 5 ways to Drop One or Multiple Columns in Pandas DataFrame so that you can use the option that best suits you. Let’s get started.
Dropping Columns from Pandas DataFrame in Python
For Dropping columns from the DataFrame let’s first create a DataFrame using a CSV file.
Example:
import pandas as pd
df = pd.read_csv('Bengaluru_House_Data.csv')
print(df)
Here we have first imported pandas as pd then created the DataFrame using a CSV file Bengaluru_House_Data.csv and after that, we printed the DataFrame.
Output:
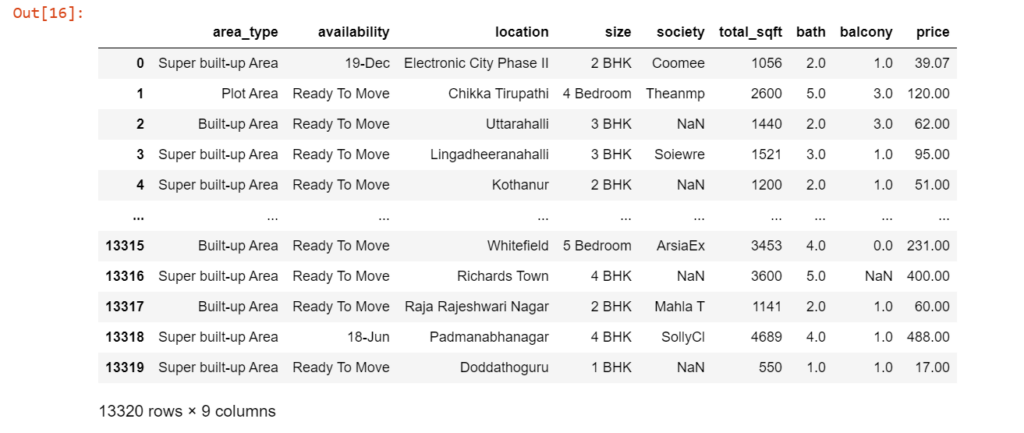
The five ways through which we can drop the columns from the Pandas DataFrame are as follows:
- Dropping Columns from a DataFrame Using the drop()
- Dropping Columns from DataFrame Using drop() based on Column Indices
- Dropping Columns from a DataFrame Using drop() with iloc[]
- Dropping Columns from a DataFrame Using drop() with loc[]
- Dropping Columns from a DataFrame in Iterative Way
1. Dropping Columns from a DataFrame Using drop()
We can drop a single column as well as multiple columns by using the drop( ) method.
Example 1:
In this example, we will drop a single column which is size column from the DataFrame.
import pandas as pd
df = df.drop(['size'], axis = 1)
print(df)
Here we have used a function df.drop and inside it, we have defined a list in which we have defined the column name and we have taken the column size to drop. Then we have defined the axis as 1, axis=1 means for columns. Then after running, the size column will be removed from the DataFrame.
Output:
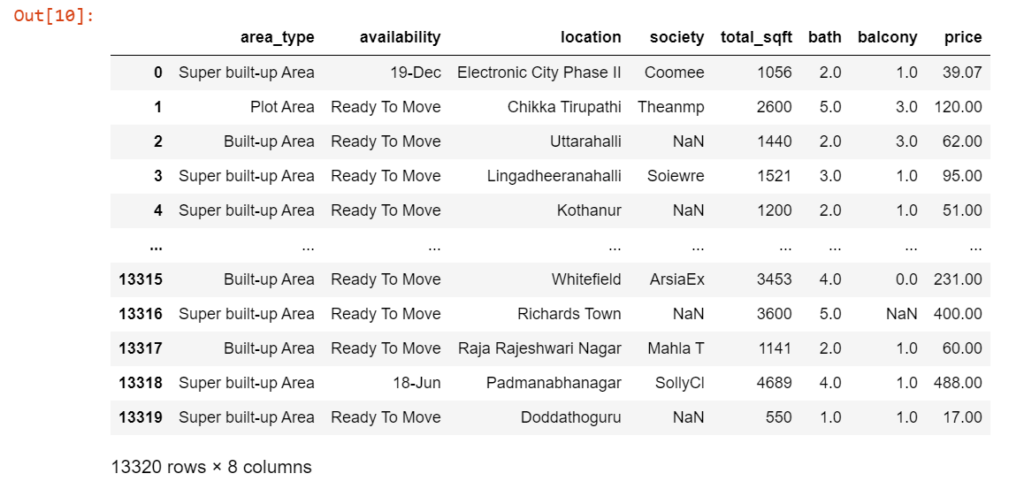
Example 2:
In this example, we will drop multiple columns from the DataFrame.
import pandas as pd
df = df.drop(['size','location'], axis = 1)
print(df)
For dropping multiple columns we have provided the column names which are location and size in the drop() function inside a list. After running the above code, the size and location column will be dropped from the DataFrame.
Output:
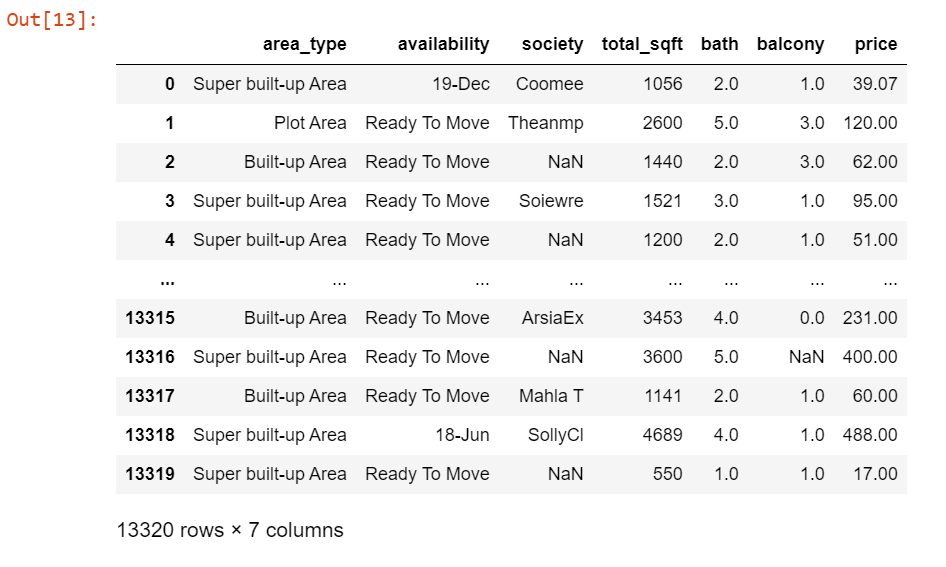
2. Dropping Columns from DataFrame Using drop() based on Column Indices
We can drop columns from DataFrame with the help drop() method and the indices of the columns.
Example:
import pandas as pd
df = df.drop(df.columns[[2,3]],axis =1)
print(df)
Here we have dropped the location and size columns having index 2 and 3. After running the above code, the size and location columns will be dropped.
Output:
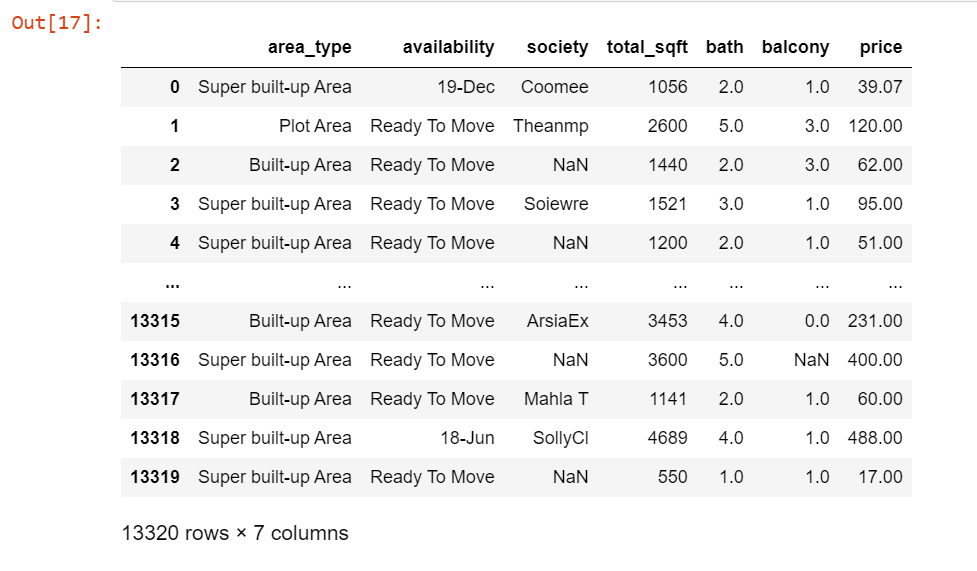
3. Dropping Columns from a DataFrame Using drop( ) with iloc[]
We can drop columns from the DataFrame using the drop( ) method and iloc[ ] together.
Example:
import pandas as pd
df = df.drop(df.iloc[:,2:4],axis =1)
print(df)
Here we have first written the function df.drop( ) and passed df.iloc[ ] as an argument inside which we have to write what we wanted to drop. We wanted to delete some columns and all the rows of those columns. In iloc[ ] first parameter is for rows and the second parameter is for columns. For rows, we wanted to delete all rows that’s why it will be a single colon (:) and we have to provide a second parameter for the column that’s why we used commas to separate it. Since we wanted to remove the location and size columns, we have to pass index 2 to 4 as 4 will not be taken. After running the above code, the size and location columns will be dropped from the DataFrame.
Output:
![Dropping Columns from a DataFrame Using drop( ) with iloc[] Output](https://codeforgeek.com/wp-content/uploads/2023/08/Screenshot-1636.png)
4. Dropping Columns from a DataFrame Using drop() with loc[]
We can also drop columns from the DataFrame using drop( ) and loc[ ] together. loc[ ] is the same as iloc[ ], the difference is we have to define the column name here rather than the index.
Example:
import pandas as pd
df = df.drop(df.loc[:,'availability':'society'].columns, axis =1)
print(df)
Here we have used df.drop( ) and passed df.loc[ ], we have defined the column name availability and society to drop. After running the above code, all the columns between these columns will be dropped.
Output:
![Dropping Columns from a DataFrame Using drop() with loc[] Output](https://codeforgeek.com/wp-content/uploads/2023/08/Screenshot-1637.png)
5. Dropping Columns from a DataFrame in Iterative Way
This is the last method for dropping columns from the DataFrame which will use del and for loop hence it is called the iterative way of deleting the columns.
Example:
import pandas as pd
for col in df.columns:
if 'size' in col:
del df[col]
print(df)
Here we ran a for loop which iterated over all the columns and then we defined the column name as size. Then we wrote if ‘size’ in col: after that del df[col] means we have iterated over all the columns and if found the size column we have to delete that column. In last we have printed the DataFrame that is df.
After running the above code, we have seen that the size column is removed and changes have come to our original DataFrame.
Output:
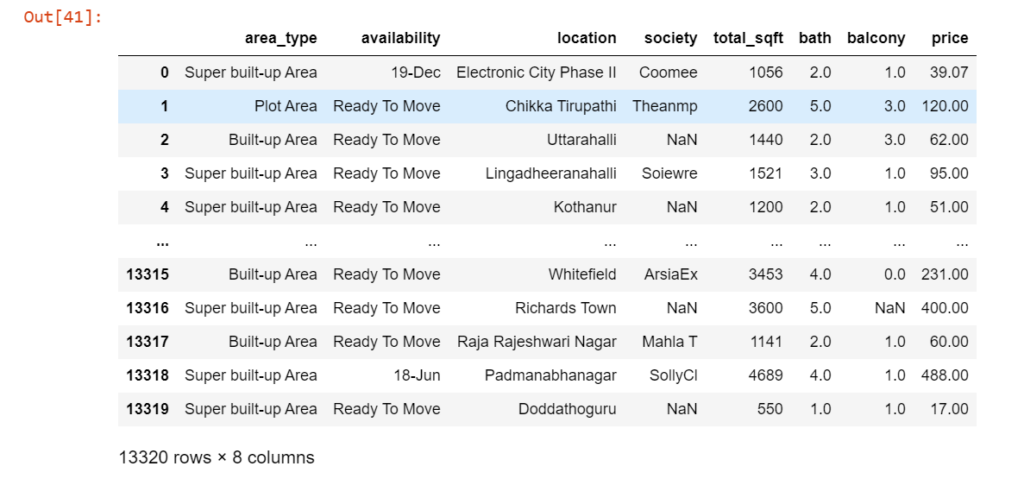
Note: In the previous methods, the size column was present in the original DataFrame after applying those methods but now in this method, we have seen that the size column is not there in the original DataFrame anymore so that means that this last method changes the original DataFrame.
Summary
Dropping columns from Pandas DataFrame is a powerful and flexible feature that allows us to manipulate DataFrame efficiently. It helps us to focus on relevant data and prepare the data for further analysis. In this article, we have discussed five ways of dropping columns with examples. After reading this tutorial, we hope you can easily drop columns from Pandas DataFrame in Python.
Reference
https://stackoverflow.com/questions/13411544/delete-a-column-from-a-pandas-dataframe