Tic-Tac-Toe is a fun two-player game played on a 3×3 grid. Players take turns marking either ‘X’ or ‘O’ in one of the nine spaces in the grid. The objective is to be the first player to align three of their marks horizontally, vertically, or diagonally. Did you know you can create one using JavaScript? Let me show you how!
Understanding the Rules of Tic-Tac-Toe
Before we start creating the game, it’s important to understand the rules. This will guide our approach and make it easier to develop the game’s logic. Here are the rules:
- The game is played on a 3×3 grid.
- Two players take turns, with one using ‘X’ and the other ‘O’.
- A player wins by placing three of their marks in a row (horizontally, vertically, or diagonally).
- The game ends in a draw if all spaces are filled and no one has won.
Building a Tic-Tac-Toe Game Using JavaScript
We will walk through building a basic version of the Tic-Tac-Toe game using HTML, CSS, and JavaScript.
Step 1: Setting Up HTML Structure
Firstly we create the basic structure with a 3×3 grid for the game.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tic-Tac-Toe</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Tic-Tac-Toe Game</h1>
<div id="game-board">
<div class="cell" id="cell-0"></div>
<div class="cell" id="cell-1"></div>
<div class="cell" id="cell-2"></div>
<div class="cell" id="cell-3"></div>
<div class="cell" id="cell-4"></div>
<div class="cell" id="cell-5"></div>
<div class="cell" id="cell-6"></div>
<div class="cell" id="cell-7"></div>
<div class="cell" id="cell-8"></div>
</div>
<script src="index.js"></script>
</body>
</html>
This HTML structure creates a 3×3 grid using div elements. Each cell is given a unique ID for easy reference in JavaScript.
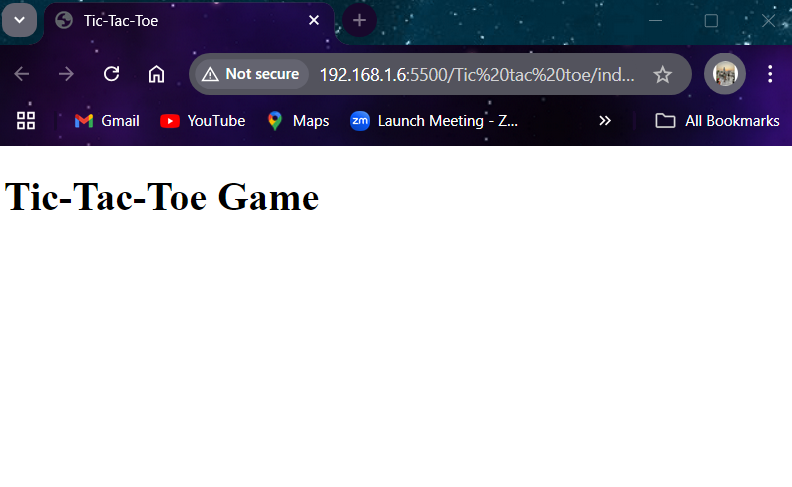
Enjoy making games with JavaScript? Be sure to check out how to create a Snake game using JavaScript!
Step 2: Styling Grid with CSS
Next, we will add basic styling to make the game visually appealing.
body {
font-family: Arial, sans-serif;
text-align: center;
background-image: url('image.png');
background-size: cover;
background-position: center;
background-repeat: no-repeat;
}
h1 {
font-style: italic;
color: white;
background-color: rgb(84, 219, 91);
padding: 10px;
display: inline-block;
}
#game-board {
display: grid;
grid-template-columns: repeat(3, 100px);
grid-gap: 8px;
justify-content: center;
margin-top: 50px;
}
.cell {
width: 100px;
height: 100px;
background-color: rgb(84, 219, 91);
font-size: 5em;
color: aliceblue;
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
}
This CSS code creates a grid for the game board and styles the cells. Each cell is given a fixed size and is aligned in the centre. We have also added some styling to the board and given a background image.
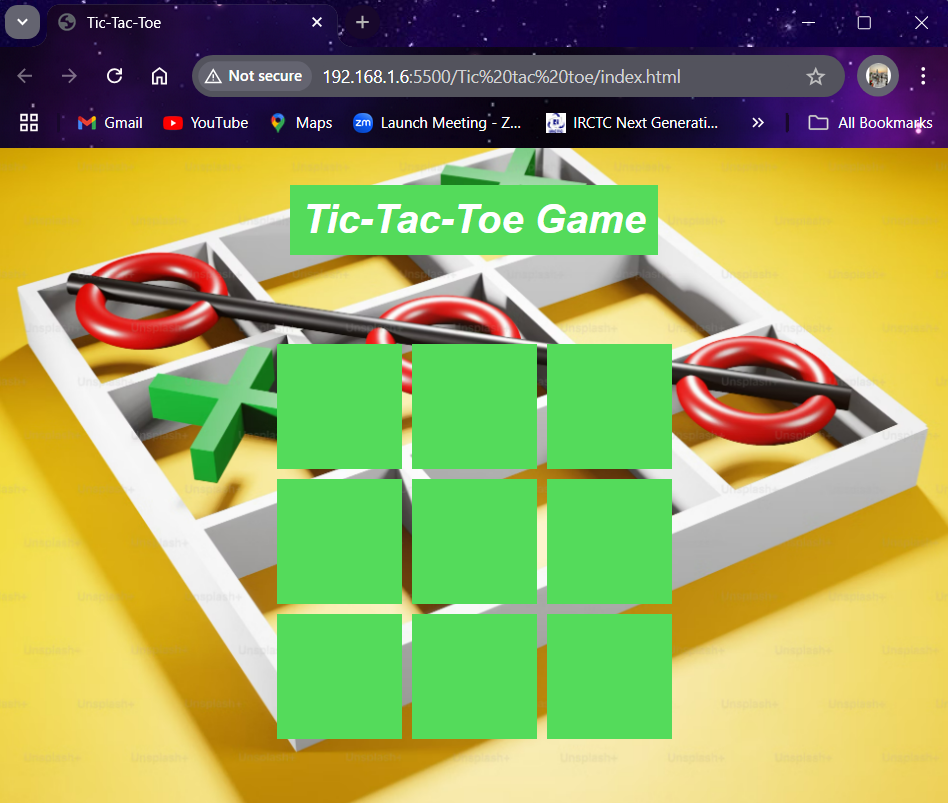
Step 3: Adding Logic with JavaScript
Now, we will add the game functionality using JavaScript.
let currentPlayer = "X";
let board = ["", "", "", "", "", "", "", "", ""];
const cells = document.querySelectorAll(".cell");
cells.forEach((cell, index) => {
cell.addEventListener("click", () => {
if (!board[index]) {
board[index] = currentPlayer;
cell.innerText = currentPlayer;
if (checkWinner()) {
setTimeout(() => {
alert(`${currentPlayer} wins!`);
resetGame();
}, 100);
} else if (board.every(cell => cell !== "")) {
setTimeout(() => {
alert("It's a draw!");
resetGame();
}, 100);
} else {
currentPlayer = currentPlayer === "X" ? "O" : "X";
}
}
});
});
function checkWinner() {
const winningCombinations = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6]
];
return winningCombinations.some(combination => {
return combination.every(index => board[index] === currentPlayer);
});
}
function resetGame() {
board = ["", "", "", "", "", "", "", "", ""];
cells.forEach(cell => cell.innerText = "");
}
JS Explanation:
- Variables: currentPlayer tracks whose turn it is (‘X’ or ‘O’). board is an array representing the 3×3 grid.
- Event Listeners: Each cell listens for a click event. When clicked, it marks the cell with the current player’s symbol (‘X’ or ‘O’).
- Check for Winner: The checkWinner function checks if any of the winning combinations are satisfied by the current player. If so, the game declares the winner.
- Reset: The resetGame function clears the board for a new round after a win or a draw.
Output:
Now it’s time to test our game, bring your co-gamer and let the game begin. Here is how our game will work:
Conclusion
HTML creates the basic structure of the Tic-Tac-Toe game, like the grid. CSS makes the game look nice with colours and layout. JavaScript adds the game’s logic, allowing players to click, mark Xs and Os, and check for winners. All three work together to make the game function. You can further modify it according to your customisations and add features like score tracking or an AI opponent.
Python is also a great tool for building such projects and it is very easy to learn. Here are some cool projects we made using Python:
- Creating an Alarm Clock with Tkinter
- Creating a Savings Calculator in Python
- Building a Simple Calculator using Streamlit
- Creating QR Codes with Python
- Creating an Age Calculator in Python
Reference
https://stackoverflow.com/questions/68796049/tictactoe-game-in-javascript