A NodeList is indeed a reliable friend. But wait, they’re not quite as flexible as your regular array buddies. Sometimes, while coding, there are specific methods and tricks you may want to apply to your project. However, NodeList doesn’t always make it easy to perform these tasks, whereas ArrayList is more accommodating. In such cases, you might need the skill to convert them and align them with the standards of your projects. In this article, we will learn how to sort this problem. So let’s get started!
Introduction to NodeList
A NodeList is like an ordered collection of elements, similar to an array, containing things like pictures or text in a specific sequence. While it’s usually immutable, meaning you can’t directly modify its content, changes in the document are reflected.
NodeList is commonly encountered in various scenarios in web development:
- Elements are chosen using methods like querySelectorAll() or getElementsByTagName(), which gives back a NodeList which is a collection of selected DOM elements.
- DOM traversal involves iterating through child nodes or using methods like childNodes, resulting in another NodeList.
- For event handling, NodeList is used when collecting elements affected by an event, like those from getElementsByClassName().
Examples of DOM Queries That Return NodeList Objects
Using querySelectorAll():
const animals = document.querySelectorAll('.Animal-class');
// 'animals' is a NodeList containing all elements with the class 'Animal-class'
Accessing Child Nodes:
const animal1 = document.getElementById('Dog');
const childNodes = animal1.childNodes;
// 'childNodes' is a NodeList containing all child nodes of 'animal1'
Getting Elements by Tag Name:
const paragraphs = document.getElementsByTagName('p');
// 'paragraphs' is a NodeList containing all 'p' elements in the document
Why We Need for Conversion of JavaScript NodeList to Array?
Now that you know what a NodeList is, let’s have some fun and create one! Imagine we’re making an HTML file and adding a list of the 10 most loved cakes and confectionery items.
Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sweet Time!</title>
</head>
<body>
<ul><li class="Sweet Cake">Chocolate Cake</li>
<li class="Sweet Cake">Cheesecake</li>
<li class="Sweet Cake">Red Velvet Cake</li>
<li class="Sweet Cake">Ice Cream Cake</li>
<li class="Sweet Cake">Cupcakes</li>
<li class="Sweet confectionery">Macarons</li>
<li class="Sweet confectionery">Éclairs</li>
<li class="Sweet confectionery">Brownies</li>
<li class="Sweet confectionery">Donuts</li>
<li class="Sweet confectionery">Fruit pie</li></ul>
</body>
<script>
//Let's grab all the sweet items first!!
const allsweets = document.querySelectorAll('.Sweet');
console.log(allsweets)// Nodelist
</script>
</html>
Output :
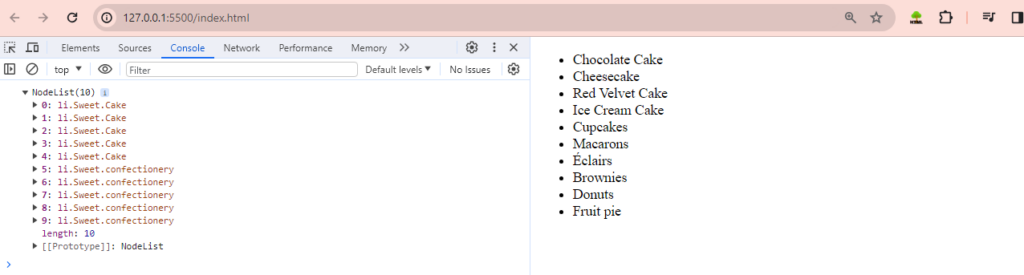
So, as you can see, this logs out all our sweet items as a NodeList. The fact that a NodeList is not an array can be problematic because node lists only support a selected few methods: .items, .keys, and .values. While these are useful, we could use .filter on them. Let’s try using .filter and see what happens. We will try to filter out Cake items.
<script>
//Let's grab all the sweet item first!!
const allsweets = document.querySelectorAll('.Sweet');
console.log(allsweets)// Nodelist
const Cakesweet = allsweets.filter((node) => {
if(node.classList[1] === 'Cake'){
return true;
}
return false;
});
</script>
Output :
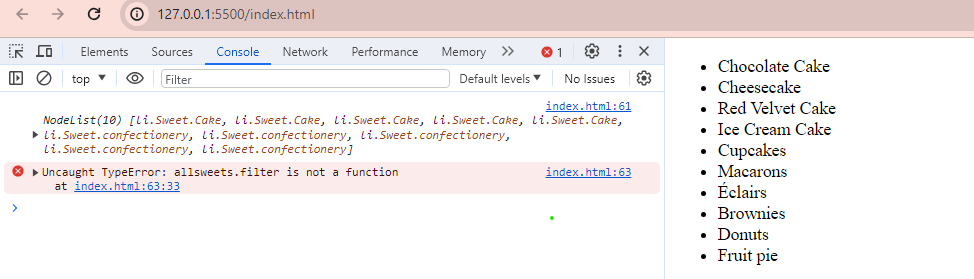
Oops, we got an error that pooped up because allsweets is a NodeList, not an array. To use functions like .filter, we need to convert the NodeList to an array.
NodeList lacks essential array methods and properties, such as map(), filter(), forEach(), and length. It lacks some of the convenient features found in arrays, making it less flexible for handling groups of DOM elements. To work more efficiently with DOM elements, developers often convert NodeList to an array. This conversion allows them to use a variety of helpful array methods, making tasks like filtering, mapping, and iterating through elements easier and more versatile.
Now that we know the need for conversion let’s explore some of the useful methods of conversion using the same example.
Methods of Conversion of JavaScript NodeList to Array
1. Array.from
Array.from in JavaScript is a method that turns NodeList or strings into easy-to-use arrays, which allows you to use array methods and properties on them. It is the most convenient method for this conversion. Let’s see how.
Syntax:
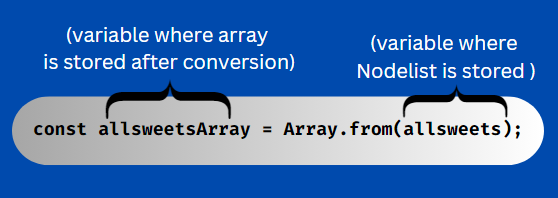
Code:
<script>
//Let's grab all the sweet item first!!
const allsweets = document.querySelectorAll('.Sweet');
console.log(allsweets)// Nodelist
const allsweetsArray = Array.from(allsweets);
const Cakesweet = allsweetsArray.filter((node) => {
if(node.classList[1] === 'Cake'){
return true;
}
return false;
})
console.log(Cakesweet);
</script>
Output:
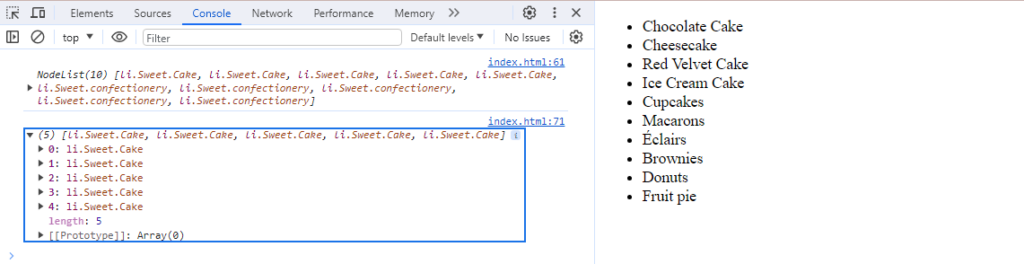
The .filter method works now as the data is successfully converted to an array as we use the Array.form.
2. Spread Operator
The spread operator (
Syntax:
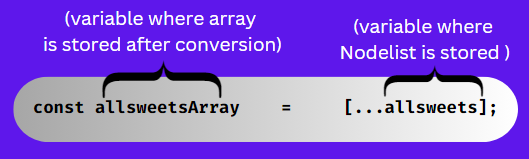
Code:
<script>
//Let's grab all the sweet item first!!
const allsweets = document.querySelectorAll('.Sweet');
console.log(allsweets)// Nodelist
// Convert NodeList to an array using the spread operator
const allsweetsArray = [...allsweets];
const Confectionerysweet = allsweetsArray.filter((node) => {
if(node.classList[1] === 'confectionery'){
return true;
}
return false;
})
console.log(Confectionerysweet);
</script>
Output:
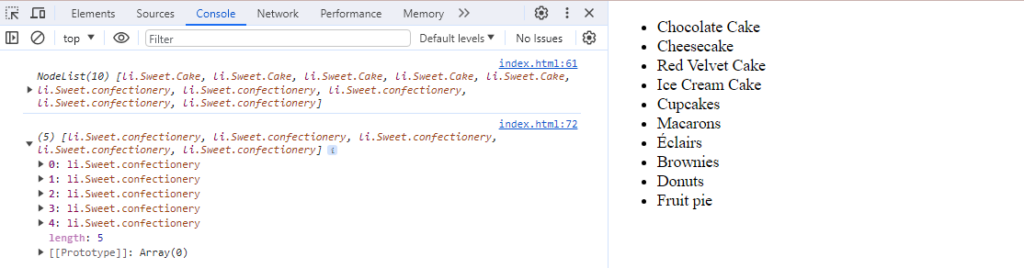
3. Array.prototype.slice.call()
Array.prototype.slice.call is an older method that turns array-like things, like NodeList, into real arrays by borrowing tricks from arrays. It’s not used much now because newer methods like the spread operator or Array.from are simpler and cooler. But hey, it’s good to know about the old tricks too!
Syntax:
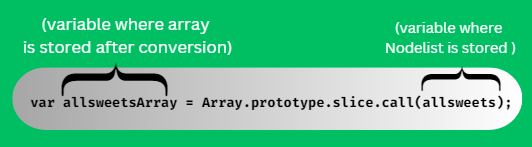
Let’s add Dark Chocolate to the list and try to filter it out as a chocolate category.
Code:
<script>
//Let's grab all the sweet item first!!
const allsweets = document.querySelectorAll('.Sweet');
console.log(allsweets)// Nodelist
// Convert NodeList to an array using the spread operator
var allsweetsArray = Array.prototype.slice.call(allsweets);
const Chocolatesweet = allsweetsArray.filter((node) => {
if(node.classList[1] === 'chocolate'){
return true;
}
return false;
})
console.log(Chocolatesweet);
</script>
Output:
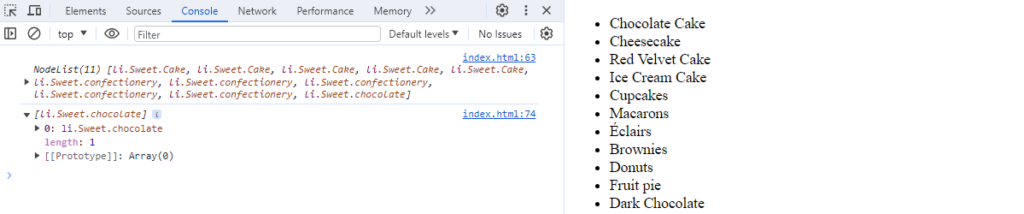
Summary
Okay, so that’s it! Now you know three ways to convert a NodeList to an array. You might have also understood the concepts of a NodeList and why it’s important to change it into an array. If you enjoyed reading this article, you might also like some of the ones listed below.
Further Reading:
Reference
https://stackoverflow.com/questions/3199588/fastest-way-to-convert-javascript-nodelist-to-array