Who doesn’t like a KitKat break, just like us our code also needs breaks. Sometimes, you might want your program to wait a moment before doing something. This can be helpful for testing and controlling the speed of actions.
In Node.js, the traditional concept of “sleep” or blocking synchronous operations is discouraged as the beauty of Node.js lies in its simplicity and asynchronous programming model. It is purely event-driven and non-blocking.
To know more about asynchronous programming and its importance, refer to this article: Asynchronous Programming in Node.js – Callback, Promises & Async/Await
So to carry the idea of sleep and wait time smoothly in our programs, we use asynchronous methods over synchronous code. In this article, we will deep dive into sleep in Node.js, its importance and the asynchronous ways to achieve it with suitable examples.
Introduction to Sleep
A sleep function is like a pause button in programming. It allows your code to wait or take a break for a specified amount of time. In Node.js, there isn’t a native blocking sleep function to achieve a wait-like behaviour.
Why Do Programs Need Sleep?
Here are a few scenarios where you find a sleep function to be useful:
- Imagine you’re testing a game, and you want to see how it reacts after waiting for a few seconds. A sleep function can help in creating controlled delays during tests.
- If your program talks to other programs or services, some of them might say, “Hey, don’t talk to me too fast!” A sleep function helps you slow down and follow those rules.
- When you’re working on things like buttons or animations, sometimes you need to make things happen with a little break in between. That’s where a sleep function comes in handy โ it helps you control when different actions or transitions take place.
- Another scenario arises when you’re handling a bunch of API requests one after the other, it’s a good idea to add a little break between them. This way, you also avoid hitting any limits set by your API provider due to too many requests coming in too quickly.
Creating a Sleep or Delay in Node.js
Let us now see how we can achieve sleep functionality in Node.js using key methods.
1. setTimeout Function
In Node.js, you can add a delay to your code execution using the setTimeout function. All you have to do is just provide a callback function and the desired delay time in milliseconds as parameters.
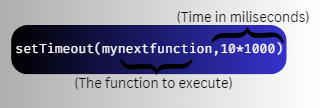
Let us understand it with an example.
Example:
function mynextfunction(){
console.log("[Let's learn about the Kitkat Break of node] "+ new Date());
}
function main(){
console.log("[Welcome to Codeforgeek!] "+ new Date());
// call mynextfunction after 10s
setTimeout(mynextfunction,10*1000);
}
main();
Here, we have created two functions: mynextfunction() and main(). Both are instructed to display a message to the console, but there will be a gap of 10s between the display of two messages.
If you look carefully we insert the mynextfunction() as a parameter to the setTimeout method and time as 10s. When the main() function is called, [Welcome to Codeforgeek!] is printed in the terminal, then after a delay of 10s [Let’s learn about the Kitkat Break of node] gets printed. Basically, we offer a 10s nap to mynextfunction() before it is called.
Output:

The exact time is also printed along with the text as we have used the “new Date()”. The first message gets printed at 19:45:45 and the other one gets printed after a delay of 10s i.e. at 19:45:55.
2. Async/Await Function
As we have seen, the setTimeout method is where you can pass a function to execute after a certain amount of time. However, with the addition of async and await in JavaScript, we can write this solution more elegantly.
It is known that async in JavaScript is used to define a function that returns a promise, and await is used within an async function to pause execution until the awaited promise is resolved or rejected.
Feel free to follow these articles if you wish to learn more about async/await:
- Node.js Promises vs Async/Await: Which one is Better
- Call async/await Functions in Parallel: Using Promise.all, Promise.allSettled, and Promise.race
Example:
Let’s consider a real-life situation, for example, we create a game with a panda as the main character. For the time when the game gets loaded or the panda gets busy, we need to invoke a sleep function to make its functionality smooth and flexible.
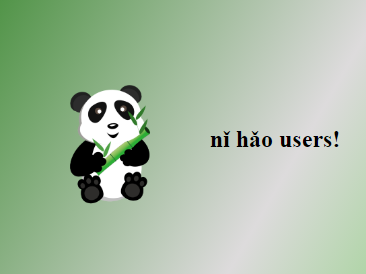
Consider the following code:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function pandagame() {
console.log('๐ผ nว hวo users! ๐ผ');
console.log('Hello from panda ' + new Date().toLocaleTimeString());
// Sleep for 1 second
await sleep(1000);
console.log('Let me go and fetch some food! ๐๐ ' + newDate().toLocaleTimeString());
console.log('๐ผ Panda is fetching data ๐');
// Sleep for 10 seconds
await sleep(10000);
console.log('Panda is ready to eat๐ผ๐! ' + new Date().toLocaleTimeString());
}
pandagame();
In this code, we have created a sleep function that returns a Promise that resolves after a specified number of milliseconds. It uses setTimeout to create a delay. Also, we have created an asynchronous function named pandagame().
The Panda program starts by logging a greeting message in Chinese and saying “Hello from panda” with the current local time. It then uses the await keyword along with a sleep function (sleep(1000)) to introduce a delay of 1000 milliseconds (1 second). This delay helps the Panda take a little break before continuing.
After the pause, the program logs a message about fetching some food, including the current local time. To mimic being busy, it logs a message indicating that the Panda is fetching data. The program uses await again, this time with sleep(10000), introducing a longer delay of 10 seconds. During this time, it’s as if the Panda is patiently waiting or working on something.
Finally, the program logs a message indicating that the Panda is ready to eat, including the current local time. The use of await and async in the program allows it to pause during the sleep functions without blocking the entire program. This helps the Panda program manage time delays in a way that aligns with the asynchronous and non-blocking nature of Node.js.
Output:

Conclusion
We hope you find this article to be fruitful and that it has increased your clarity with sleep methods in Node.js. To sum up, there may be specific scenarios where a delay or pause is necessary, such as in testing, animation, or adhering to external API rate limits. In such cases, it’s recommended to use asynchronous patterns like setTimeout with Promises or other non-blocking approaches to achieve the desired behaviour without compromising the overall performance and scalability of your Node.js application.