What if we wish to integrate languages like C, and C++ into our JavaScript code? Well, this magic is made possible by the Node-gyp! In this article let’s explore more about this awesome complex tool and learn to use it in Node.js.
Introduction to Node-gyp
It is a command line tool to build and configure native add-on modules for node.js. The gyp stands for “Generate your project“. While it’s a powerful tool, beginners might find it a bit complex so let’s break it into easy fragments for better understanding:
What are Native Modules or Add-ons?
These are special extensions for Node.js that use languages like C++ or C instead of just JavaScript. Think of them like power-ups for Node.js that let it do extra cool things beyond what regular JavaScript can handle.
Why do We Need Native Modules?
Sometimes, certain tasks need super-fast performance or involve talking to your computer’s brain (the operating system) in a way that’s easier with languages like C++. Native modules help when we want to do things that regular JavaScript can’t do as efficiently.
Role of Node-gyp
Imagine you’ve got this special power-up (the native module), but it’s like a LEGO piece that needs to be put together for your computer to understand. Node-gyp is like your builder tool. It reads a set of instructions (a file called binding.gyp) and puts together the LEGO pieces, so your special power-up works perfectly with Node.js.
In a Nutshell, it’s like having a regular superhero (Node.js) with some extra gadgets (native modules) and a trusty sidekick (Node-gyp) to make everything work seamlessly!
Installing Node-gyp Using NPM
In the terminal or command prompt, type the following command and run it:
npm install -g node-gyp
This command uses npm (Node Package Manager) to install node-gyp globally on your system. After the installation completes, you can check if node-gyp is installed correctly by typing:
node-gyp --version
If everything is set up properly, this command will display the version number of node-gyp. Something like this:

That’s it! You’ve successfully installed
Commands for Native Module Integration
Here are some important Node-gyp commands and their descriptions:
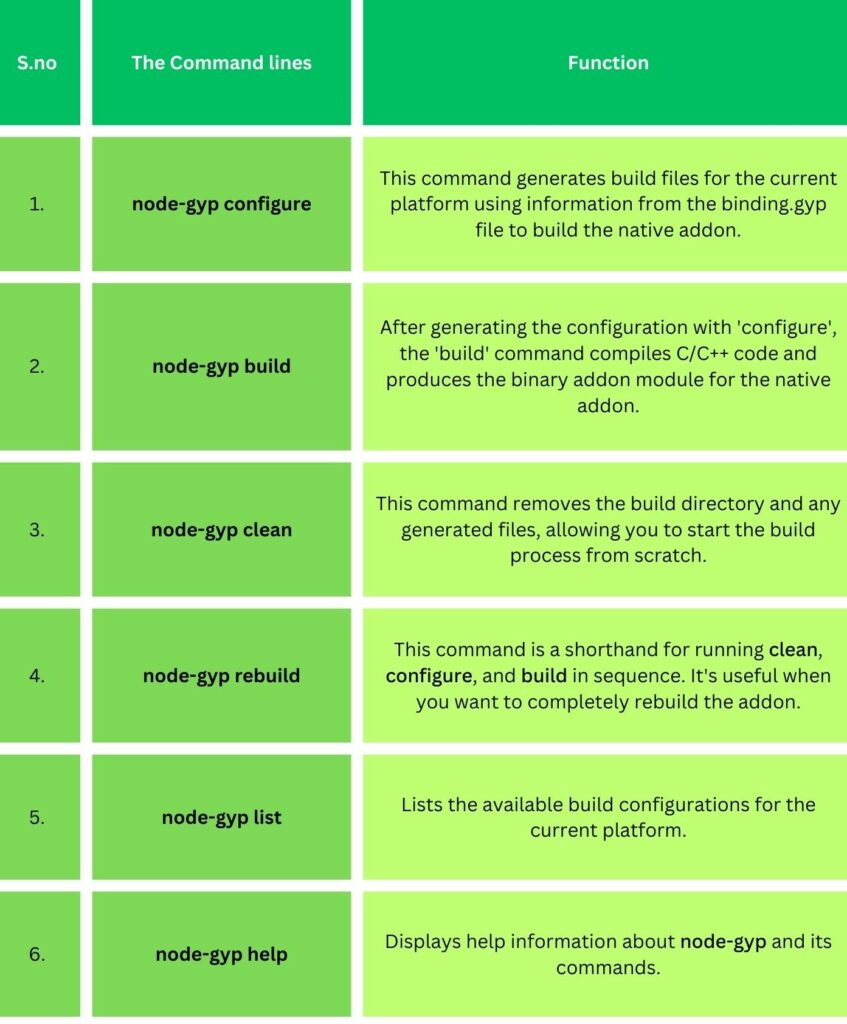
Configuration: An Example of Using Node-gyp
Let’s walk through a simple example where we create a native module that adds two numbers together. We’ll use C++ as the native language and Node-gyp to build and integrate it into a Node.js application.
1. Create a C++ File (addon.cpp)
Create a file named addon.cpp in your project folder with the following code:
#include <node.h>
using namespace v8;
void Add(const FunctionCallbackInfo<Value>& args) {
Isolate* isolate = args.GetIsolate();
// Check the number of arguments
if (args.Length() < 2) {
isolate->ThrowException(Exception::Error(
String::NewFromUtf8(isolate, "You need to pass two numbers").ToLocalChecked()));
return;
}
// Convert arguments to numbers
v8::Local<v8::Context> context = isolate->GetCurrentContext();
double value = args[0]->ToNumber(context).ToLocalChecked()->Value() + args[1]->ToNumber(context).ToLocalChecked()->Value();
// Create a new JavaScript Number with the result
Local<Number> result = Number::New(isolate, value);
args.GetReturnValue().Set(result);
}
void Initialize(Local<Object> exports) {
NODE_SET_METHOD(exports, "add", Add);
}
NODE_MODULE(NODE_GYP_MODULE_NAME, Initialize)
2. Create a Binding File (binding.gyp)
Create a file named binding.gyp in your project folder:
{
"targets": [
{
"target_name": "addon",
"sources": [ "addon.cpp" ]
}
]
}
3. Build the Module
Run the following commands in your terminal:
node-gyp configure
The run was successful as ok is printed on the last line.
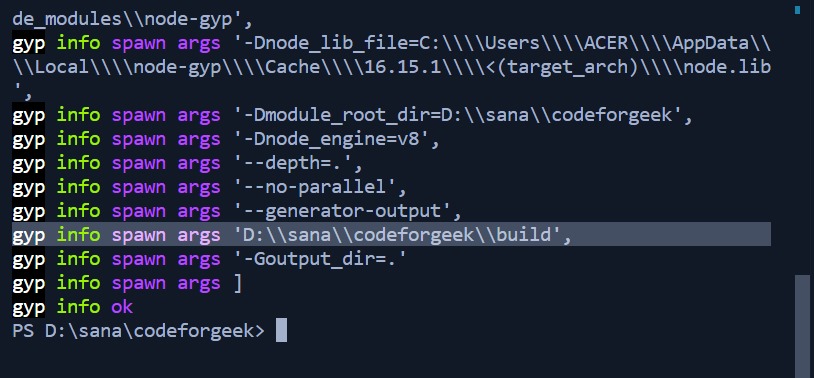
node-gyp build
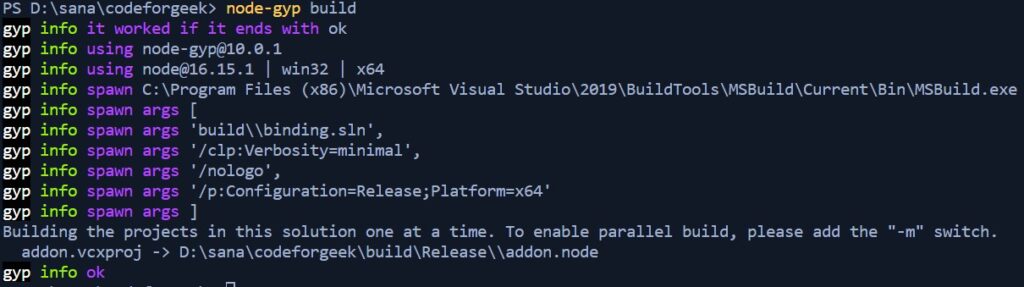
This will compile your C++ code into a binary addon.
4. Create Node.js Script (app.js)
Create a file named app.js in your project folder and write the given code:
const addon = require('./build/Release/addon');
const result = addon.add(5, 3);
console.log('Result:', result);
5. Run the Node.js Script
In your terminal, run this command:
node app.js
You should see the result of adding 5 and 3, which is 8. The one like this:

Conclusion
In a nutshell, Node-gyp is like our friendly sidekick helping us blend C and C++ languages into our JavaScript world. It’s the go-to tool for adding extra powers (native modules) to boost Node.js. With a breezy installation and simple commands, Node-gyp acts as our handy builder, making the whole process feel like a walk in the park. Give it a shot with the example, and hope you find it all crystal clear and fun to play with!
Also if you had fun reading this article, we have more in store for you:
- Executing Command Line Binaries with Node.js: A Detailed Guide
- How to Downgrade Node Version: 3 Easy Ways
- How to Check If a File Exists in Node.js
Reference
https://stackoverflow.com/questions/39739626/what-is-node-gyp