Python is a language of wonders, which comes with a list of many brilliant modules that make it easy to use for any user. One such very useful library is the Pyperclip library. In this page of Python content, we will explore clipboard operations in Python using Pyperclip. We will see its installation, features, and basic usage, and also build some applications using the library. Sounds interesting? Let’s get started.
Pyperclip Module in Python
Pyperclip is a Python library that makes it easy for developers to work with the clipboard in their Python scripts or applications. It provides a straightforward way to access clipboard functionality on various operating systems.
With Pyperclip, developers can easily copy text to the clipboard, paste text from it, and check its current contents. Additionally, Pyperclip offers text editing features, allowing developers to modify the text before or after using the clipboard.
Installation Guide
You can use pip, the Python package manager, to install Pyperclip. After opening your terminal or command prompt, just type the command below and hit enter:
pip install pyperclip
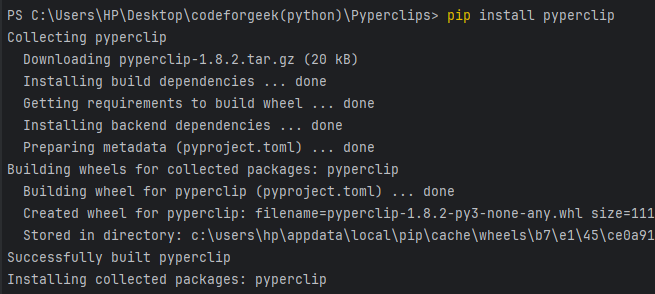
After installing, check if Pyperclip is installed correctly by opening a Python interpreter and attempting to import the module:
import pyperclip
If there are no errors, Pyperclip is successfully installed, and you can begin using it in your Python scripts.
Using Pyperclip Module in Python
Now that Pyperclip is installed, let’s explore how we can use this useful library in our projects. We’ll start with some basic uses and then move on to creating slightly more advanced applications.
Example 1:
In this example, we will see how we can implement a simple copy and paste for certain text using Pyperclip. Take a look at the provided code:
import pyperclip
# Copy text to clipboard
Random_text = "We will see how to use basic copy and paste"
pyperclip.copy(Random_text)
print("Copied text:", Random_text)
# Paste text from clipboard
pasted_text = pyperclip.paste()
print("Pasted text:", pasted_text)
- The code imports the pyperclip module in order to work with the clipboard.
- It copies the string “We will see how to use basic copy and paste” to the clipboard using pyperclip.copy() and also prints the copied text.
- It then pastes the content of the clipboard into a variable named pasted_text using pyperclip.paste(), and prints the pasted text.
Output:
We will see the copied text and pasted text printed in the console.
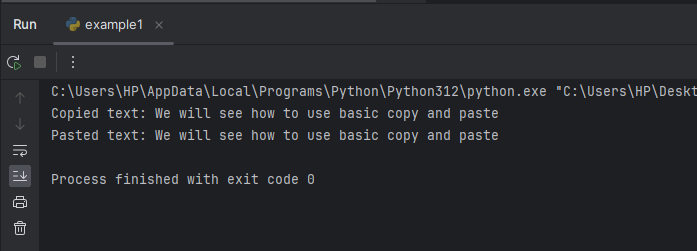
Example 2:
In this example, we will see how we can check whether the clipboard is empty or it contains some text. Take a look at the provided code:
import pyperclip
if pyperclip.paste():
print("Clipboard contains text.")
else:
print("Clipboard is empty.")
- The code imports the pyperclip module.
- It checks if there is any text content in the clipboard using pyperclip.paste().
- It prints “Clipboard contains text.” if there is text, otherwise prints “Clipboard is empty.“.
Output:
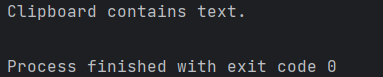
Creating a Bookmark Manager Using Pyperclip in Python
Now that we know how to use the library in simple cases, let’s advance to some more complex project.
Let’s learn how we can create a basic bookmark manager using the given library. Take a look at the provided code.
import pyperclip
bookmarks = {}
def add_bookmark(name, url):
bookmarks[name] = url
def open_bookmark(name):
if name in bookmarks:
pyperclip.copy(bookmarks[name])
print(f"Opening bookmark '{name}': {bookmarks[name]}")
else:
print(f"Bookmark '{name}' not found.")
add_bookmark("Google", "https://www.google.com")
add_bookmark("GitHub", "https://github.com")
# Open bookmark and copy URL to clipboard
open_bookmark("Google")
Let’s break down the given code step by step-
Step 1: First, we will import the Pyperclip module by using the following code.
import pyperclip
Step 2: Now we will initialize an empty dictionary named bookmarks. This dictionary will store the bookmark names as keys and their corresponding URLs as values.
bookmarks = {}
Step 3: Now we will define a function called add_bookmark(), which takes two parameters: name (the name of the bookmark) and url (the URL associated with the bookmark). Inside the function, it adds an entry to the bookmarks dictionary with name as the key and url as the value.
def add_bookmark(name, url):
bookmarks[name] = url
Step 4: Next, we’ll define the open_bookmark() function, which takes a parameter, name, representing the bookmark’s name to open. This function checks if the given name exists in the bookmarks dictionary. If found, it copies the associated URL to the clipboard using pyperclip.copy() and prints a message confirming the opening along with the URL. If the name doesn’t exist in the dictionary, it prints a message indicating that the bookmark wasn’t found.
def open_bookmark(name):
if name in bookmarks:
pyperclip.copy(bookmarks[name])
print(f"Opening bookmark '{name}': {bookmarks[name]}")
else:
print(f"Bookmark '{name}' not found.")
Step 5: Then we will call the add_bookmark() function twice to add two bookmarks: “Google” with the URL “https://www.google.com” and “GitHub” with the URL “https://github.com“.
add_bookmark("Google", "https://www.google.com")
add_bookmark("GitHub", "https://github.com")
Step 6: Now we will call the open_bookmark() function with the argument “Google”.
open_bookmark("Google")
Output:
Since “Google” is a valid bookmark name, the function copies the associated URL to the clipboard and prints a message confirming the opening along with its URL.
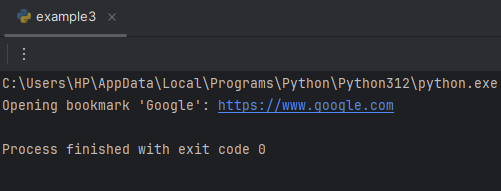
Conclusion
It seems that we have reached the end of this article. I hope that you have learned a lot about the Pyperclip library. It helped us copy and paste stuff easily. We learned how to install it, and what it could do, and even made some neat projects. From basic copy-paste to making a simple bookmark keeper, Pyperclip has made Python even more awesome.
If you liked this one, consider reading –
Reference
https://pyperclip.readthedocs.io/en/latest/