Hello Python lovers! Welcome to another informative content bunch where we will discover about finding versions of Python modules.
Why Checking Python Module Versions Matters
In Python modules, a version check means finding out the version number of a module installed in your Python setup.
Various versions of a module may offer different features or APIs, and they might bring changes that could affect existing code. Checking the module version helps ensure your code works smoothly with the specific version you’re using.
New module versions typically come with bug fixes, performance boosts, and extra features. Knowing your module’s version helps you see if you can enjoy these upgrades and decide if upgrading to a newer version is beneficial.
Python projects often rely on third-party modules, which themselves may rely on other modules. By checking the versions of all your project’s modules, you can manage dependencies better, preventing conflicts or unexpected issues.
Getting Python Module Versions
Now that we understand the importance of performing version checks, let’s explore the methods to do so.
1. Using pip show Command
pip show is a Python command-line tool for showing detailed information about a specific package installed in your environment. It reveals metadata like the package version, dependencies, location, and other details.
Open your terminal or command prompt and enter the following command:
pip show <name_of_module>
Replace <name_of_module> with the name of the Python module you wish to check.
For example:
pip show Pillow
This command will display information about the Pillow module, including its version number. The output might appear as follows:
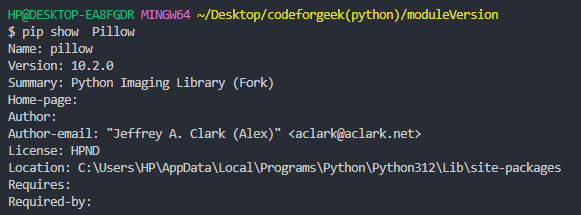
From the output, you can see that the installed version of the Pillow module in this environment is 10.20.0.
2. Using Import Statement
Another common and simple method to check the version of installed Python modules is by using the import statement and Python’s attribute access. This method directly accesses the version attribute of the module, where version information is usually stored. However, it may not work for every module as not all follow this convention.
To check the version of a Python module, create a Python script, import the desired module, access the version attribute of the module, and print it.
For example:
To check the version of the matplotlib module, you would run:
import matplotlib
print(matplotlib.__version__)
This code will display the version number of the matplotlib module. The output might appear like this:

In this case, it is 3.8.3.
3. Using pkg_resources Module
The pkg_resources module is a part of the setuptools package, commonly used for packaging and distributing Python projects. It allows interaction with installed distributions, including querying information about installed packages, like their version numbers.
Let’s see how to use the pkg_resources module to check the version of an installed Python module:
import pkg_resources
print(pkg_resources.get_distribution("DateTime").version)
Firstly, we import the pkg_resources module. Then, we use the get_distribution() function to retrieve information about the installed distribution of the DateTime module and print the version number of the DateTime module.
This code will display the version number of the DateTime module installed.

4. Using pip freeze Command
pip freeze is another way to check installed Python module versions. This command lists all installed packages in your Python environment with their versions.
To check versions using pip freeze, open your terminal or command prompt and run the following command:
pip freeze
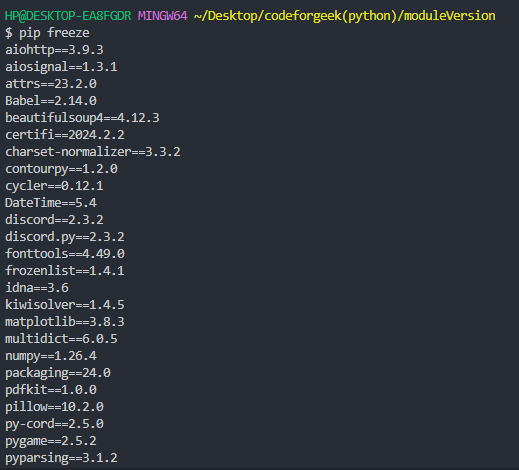
This command will display a list of all installed Python packages along with their versions. You can go through the list to find the module you want, or you can use tools like grep (on Unix-like systems) or findstr (on Windows) to filter the results.
For example:
If you want to check the version of the pygame module, you can use:
pip freeze | grep pygame

This will give you the version, which in this case is 2.5.2.
5. Using pip list Command
pip list is another command-line tool for viewing all installed Python packages and their versions. It’s like pip freeze but offers a more concise output.
To check the version of installed Python modules using pip list, open your terminal or command prompt and run the following command:
pip list
This command will display a list of all installed Python packages with their versions. Each line in the output usually shows the package name followed by its version number.
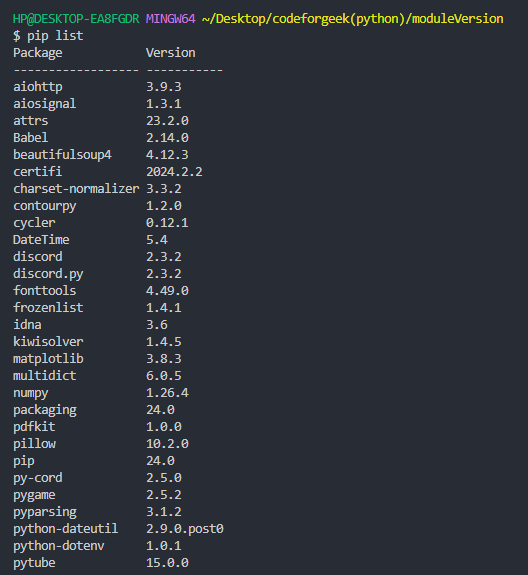
From the output, you can see the names and versions of all installed Python packages in your environment.
Conclusion
That is all for this tutorial. We hope that you now have a clear understanding of version checking methods. It is crucial to thoroughly check the versions of all packages to reduce errors resulting from version mismatches.
If you enjoyed reading this, continue reading –
Reference
https://stackoverflow.com/questions/20180543/how-do-i-check-the-versions-of-python-modules