Python is a very popular programming language, one of the reasons for its popularity is its rich library ecosystem. There are libraries available for almost everything you can think of, from web development to data science to machine learning. Some of the most popular libraries are NumPy, Pandas, Matplotlib, TensorFlow, PyTorch, Django, SciPy, Flask and many more.
Libraries are powerful tools that can help you save time, and effort, and improve the quality of your code by providing many functions that can operate on the required data efficiently.
The emoji module in Python is a library that allows you to use and print emojis in your Python programs. It is not an in-built module, so you will need to install it first.
Installing Emoji Module
To Install the emoji module, open your terminal and run the following command. If you are using Jupyter Notebook or Google Colab, you can even run the same command there in a cell.
Syntax:
pip install emoji
Output:
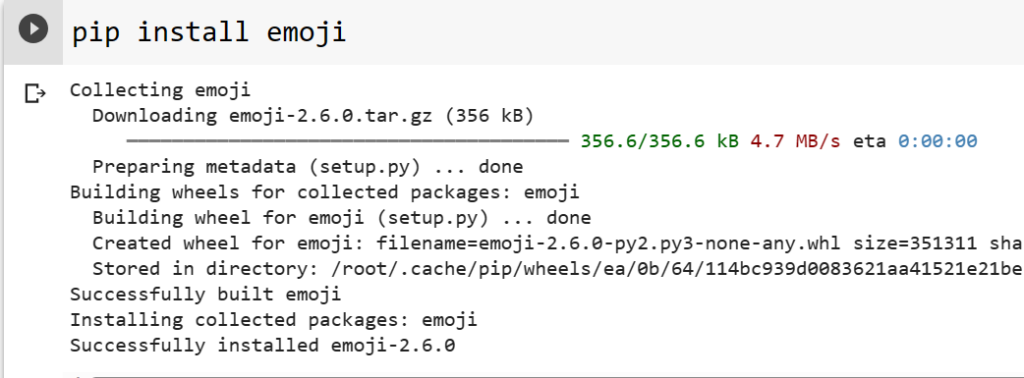
If you have already installed and want to update it, you can run the following command in the terminal or in the cell of the Notebook you are using.
pip install emoji --upgrade
Importing Emoji Module in Python
Once the module is installed and updated, you can import it into your code and use it to print emojis in your Python programs. Let us consider an example to understand it better. The following code shows how to import and use this module.
Example:
import emoji
print(emoji.emojize('I :red_heart: Python'))
Output:

Printing Emoji in Python Using Emoji Module
The entire set of Emoji codes is defined by the Unicode consortium with a particular Unicode assigned to every emoji. We can also use the aliases names of the emojis to refer to them or the Unicode to print the emojis, let us consider an example to understand it better.
Example:
import emoji
print(emoji.emojize("\U0001F604"))
print(emoji.emojize(':grinning_face_with_smiling_eyes:'))
print("😄")
Output:
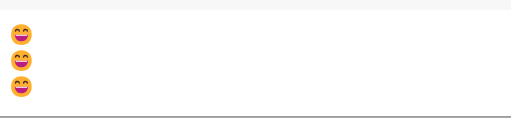
The above code shows three ways of printing emojis. Here the Unicode of grinning face with smiling eyes is U+1F604 here we need to replace the ‘+’ with ‘000’ to satisfy the length of Unicode, so it becomes ‘U0001F604’.
Emoji Module Functions
Emoji Module has many functions to access emojis in different ways. They are emojize(), demojize() and is_emoji() functions. Let us understand these functions one by one.
emojize() Function
The emojize() function takes a string as input and returns the string with the emojis replaced by their corresponding Unicode characters. For example, if you pass the string “smiley face” to the emojize() function, it will return the string “😀”.
demojize() Function
The demojize() function takes a string as input and returns the string with the Unicode characters replaced by their corresponding emoji names. For example, if you pass the string “😀” to the demojize() function, it will return the string “smiley face”.
is_emoji() Function
The is_emoji() function is quite different, it takes an argument and returns True or False indicating that the passed argument is an emoji or not.
Example:
Let us now understand these functions with an example.
import emoji
text = "This is a smiley face 😀"
print(emoji.emojize(text))
print(emoji.demojize(text))
print(emoji.is_emoji('😀'))
Output:

Language Attribute in emojize() and demojize() Functions
By default, the language of the alias name of the emoji is English (language = ‘en’) but also supported languages are Spanish (‘es’), Portuguese (‘pt’), Italian (‘it’), French (‘fr’), German (‘de’), Farsi/Persian (‘fa’).
Example:
import emoji
print(emoji.demojize('Python es 😄', language='es'))
Output:

In the above code, the demojize() function replaces the emoji with its corresponding alias name in the specified language mentioned.
Conclusion
Emojis can help to convey emotions and feelings in a way that words cannot, which is very important in text-based communication, where it can be difficult to interpret the tone of a message. Emojis can help to make communication more playful and fun and they can help to break up text and make it more visually appealing.
If you want to use emojis in your application, you can use the Python emoji library. Hope after reading this article you have got enough information about it.