There are many useful APIs for Node.js available as modules and libraries. The best part is that they are open source, meaning anyone can use them in their projects as well as contribute to them. Let me provide you with a list of the 10 best Node.js open-source APIs.
List of Node.js Open Source APIs
A good open-source project will be popular, have good documentation, be actively maintained, and have well-organized code. Let’s see them and their implementation.
1. SheetJS
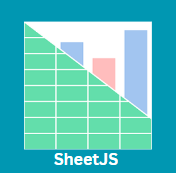
When we work with Excel files, SheetJS can be proved efficient for manipulation. It helps a lot with spreadsheets. It allows us to create, export, and edit Excel files, and convert HTML tables, arrays, or JSON into downloadable .xlsx files. There are two versions: Community (free) and Pro (paid, with more features).
Example Usage:
Let’s see a basic example to use this, let’s try to read an Excel file content.
To install SheetJS we run:
npm install xlsx
We will read the content of the following sheet:
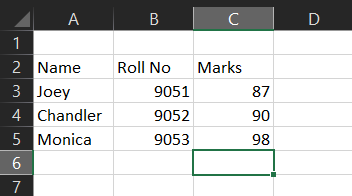
const XLSX = require('xlsx');
const workbook = XLSX.readFile('C:/Users/ACER/OneDrive/Desktop/Example.xlsx');
const sheet_name_list = workbook.SheetNames;
const sheet = workbook.Sheets[sheet_name_list[0]];
const data = XLSX.utils.sheet_to_json(sheet);
console.log(data);
This code will access the contents of the given file and log it into the console:
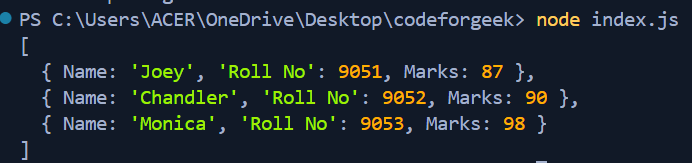
Here is the link to the official website: https://docs.sheetjs.com and GitHub: SheetJS GitHub
2. Axios
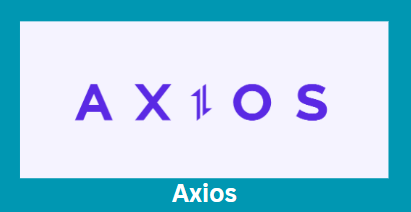
Axios is a promise-based HTTP client, which means that it can help us with HTTP requests using the Promise API. It can easily intercept, transform, and cancel requests. What makes it stand out from others is that it comes with extensive documentation and a strong community, which provokes developers to work with it.
Example Usage:
Let’s see an example to use this. We will show how to make a GET request to an external API and log the response.
To install Axios we run:
npm install axios
Let us fetch and display the details of ‘charizard’ (a Pokemon) using the Pokemon API. This is how we do it:
const axios = require('axios');
axios.get('https://pokeapi.co/api/v2/pokemon/charizard')
.then(response => {
const pokemon = response.data;
console.log(`Name: ${pokemon.name}`);
console.log(`Height: ${pokemon.height}`);
console.log(`Weight: ${pokemon.weight}`);
console.log(`Base experience: ${pokemon.base_experience}`);
})
.catch(error => {
console.error('Error making the request:', error);
});
This code will fetch the details of Charizard from the Pokemon dictionary in the API and then display them on the console:

Are you curious to study more about this API? Then do check out: Axios in Node.js: For Making HTTP Requests
3. Express.js
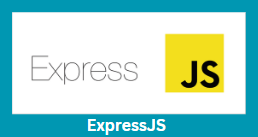
It is the most used library in Node.js. This module helps us handle HTTP requests, which adds a huge value to several side applications. It also gets included in the MEAN and MERN stacks, where E stands for express. Now, you can imagine how much importance it holds. It implements URL-based routing.
Example Usage:
Let’s create a server displaying a message.
To install express.js we run:
npm install express
We will create a server by adding the following code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Good morning Folks!');
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
This code will create a server and display the greeting on port 3000 when accessed:

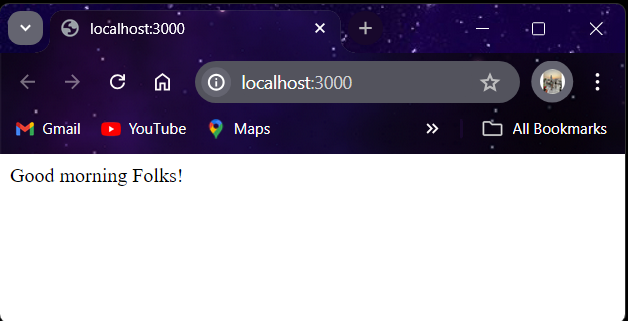
Detailed information about this library and its use is given in this article – Express Tutorial for Beginners
Official documentation: https://expressjs.com/.
4. Cytoscape.js
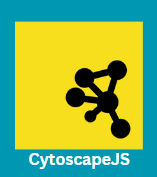
If you are a data science enthusiast, then this is the perfect library for you. It is highly focused on graph theory and visualization. It is capable of displaying beautiful and interactive graphs. But yes, one thing to note is that it is a little bit complex so here we cannot show you a basic example of how to use it.
If you are interested in playing with this library, you can check out their official website: https://js.cytoscape.org and Github: https://github.com/cytoscape/cytoscape.js
5. Strapi
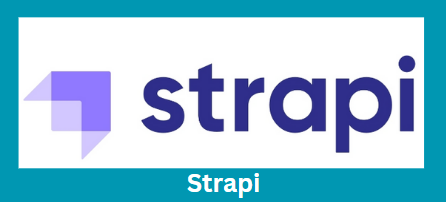
Strapi is an open-source headless CMS (content management system) that uses RESTful APIs. Its job is to deliver content across devices. It offers features like file upload, built-in email, JWT authentication, and auto-generated documentation. It has a flexible content structure, which means you can create and reuse content groups and use customizable APIs.
Here is the link to the official website: https://strapi.io and Github: https://github.com/strapi/strapi
6. NestJS
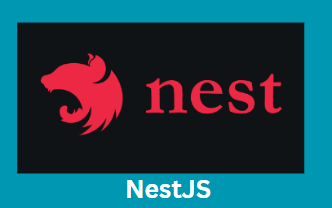
NestJs is a node.js framework that enables us to build scalable server-side applications using TypeScript. It collectively fuses object-oriented programming and functional programming. Not only does it use Express.js behind the scenes, but it also supports other libraries. It has good documentation and a contribution guide.
Official documentation: https://docs.nestjs.com
7. Cube.js
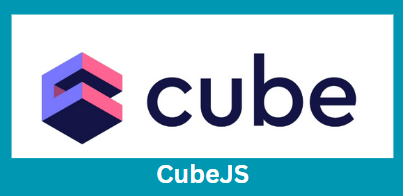
This open-source framework enables us to construct analytical web applications. It’s great for making business intelligence tools and adding analytics features to existing apps. It works with services like AWS Athena and Google BigQuery to handle huge amounts of data. Cube.js is made of modules that each do one thing well, including data transformation, querying, caching, managing APIs, and building user interfaces.
This library is not as simple as it seems, it requires deep knowledge, which you can gain by its official docs.
8. ESlint
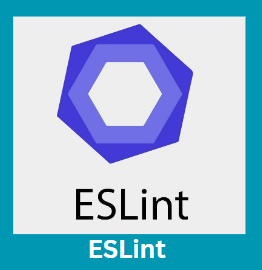
This API will prove itself very handy as it helps us figure out and fix our code errors and issues. It analyses errors, patterns, and style issues based on guidelines. It also checks its reliability before running. ESLint has default rules that you can customize. Major companies like Facebook, Netflix, and PayPal use ESLint. It has well-maintained documentation and community support.
Here is the official documentation to see and learn more about ESLint and its implementation in real-life projects.
9. Socket.IO
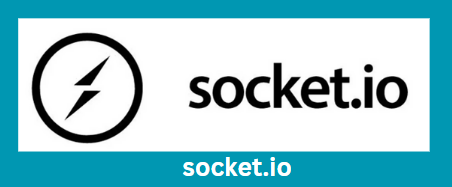
If you are in search of real-time, two-way communication, then Socket.IO is the best choice for you. It works across platforms, browsers, and devices, with great emphasis on reliability and speed. For example, in a chat app using Socket.IO, messages are instantly sent from server to client without continuous requests.
If you are more into this library, we recommend checking its official documentation.
10. Meteor.js
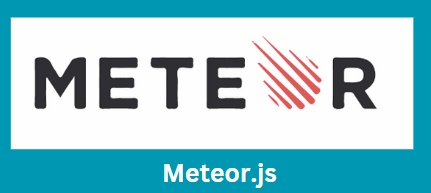
Meteor is a platform for building both web and mobile apps using JavaScript. It supports popular frameworks like React, Vue, and Angular. One big advantage is you can use the same code across different devices. Meteor comes with many pre-built modules that speed up development. There’s a large community that shares reusable packages. Big companies like IKEA and Mazda use Meteor. It has a great website and documentation, making it a solid choice for developers wanting to contribute.
You will find ample details about this great library here.
Conclusion
As you read today, many amazing APIs exist that you can use in Node.js to uplift the quality of your codebase and also add interesting features to it. In summary, choose a project that interests you and fits your skills, check its documentation and recent activity, and look for a well-organized codebase with tests. Also, if you find it fun to read about such Node.js tools, I recommend you check out this article as well: Awesome Node.js Tools, Libraries, and Resources.
Reference
https://stackoverflow.com/questions/10361027/robust-open-source-node-js-based-projects-for-learning