In web development, Node.js provides us with a runtime environment such that we can run JavaScript codes outside the web browser. It follows a non-blocking and event-driven architecture. Now the question is, is Node.js frontend or backend-oriented?
Is Node.js Frontend or Backend?
Before we study which side Node.js inclines on the most, let’s understand what we mean by backend and frontend.
Assume that we went to watch a movie. Now the actors, visuals, sets, and background are the parts that we see directly on the screen: this is the frontend, and the working of crew members behind the scenes is the backend.
In this analogy, Node.js can be compared to a director who is more focused on the workings of the backend. The director (Node.js) ensures that when the actor interacts with a prop, the correct action happens, like fetching or updating data in the database.
Also if you are curious to know more about Node.js, Click here!
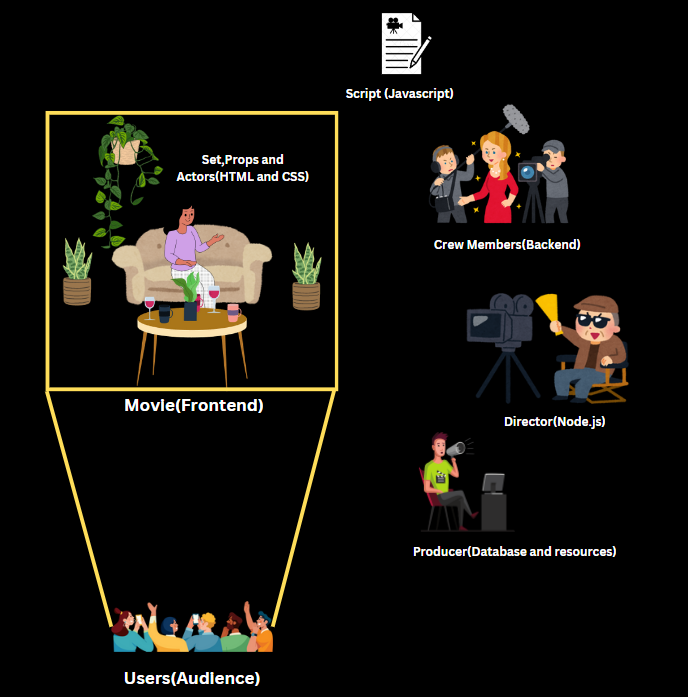
Now, back to technical terms, Node.js is server-side, which is purely the backend, but it is written in Javascript, which is a frontend language. Node.js is not exactly built for the frontend, but it can be used to work with it.
When we use packages like Express.js, React.js, and Angular.js, we are actually implementing Node.js in the frontend, like generating and sending HTML pages based on certain conditions. When we talk about Node.js in the backend, it is used to build server-side applications that manage network requests, process data, and interact with databases. Also, it provides web sockets and a strong API.
This makes it clear that Node.js can be played in both the frontend and the backend. It means that it is a full-stack-supporting technology because it is versatile enough to handle both server-side and client-side servers.
Now to prove my point, I will walk you through some examples where we will see how node.js works in the frontend and backend.
How Node.js Works as Both Frontend and Backend?
We will try to understand the functioning of Node.js as frontend and backend with some examples to make things more clear.
Node.js for Frontend:
What we will do here is use a Webpack with Node.js to bundle JavaScript files. A Webpack helps us with bundling and module management. Here is the stepwise procedure of the example project.
Step 1: We create a Node.js project using the given command.
npm init -y
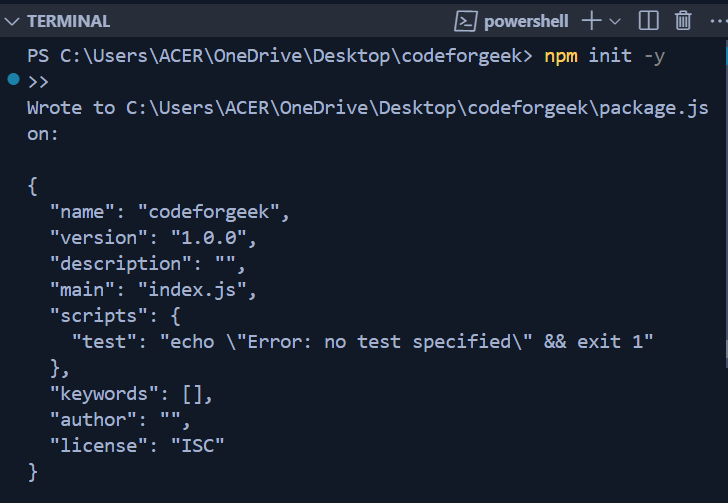
Step 2: Then we install the Webpack and its CLI tool.
npm install webpack webpack-cli --save-dev
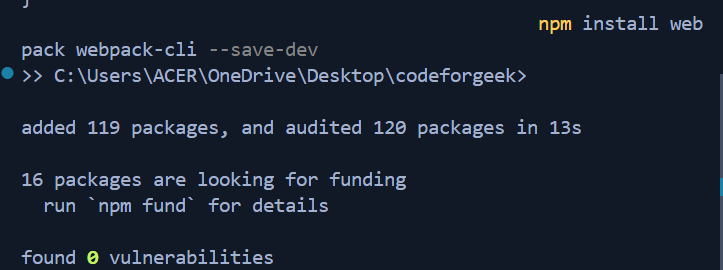
Step 3: Now we will make a JavaScript file named index.js for frontend. It will contain the following content:
function hello() {
const element = document.createElement('div');
element.innerText = 'Hello, Webpack and Node.js!';
document.body.appendChild(element);
}
hello();
This function will print a hello message on our webpage.
Step 4: Then we form a Webpack configuration file that contains the following code:
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist')
},
mode: 'development'
};
Your File structure should look like this:
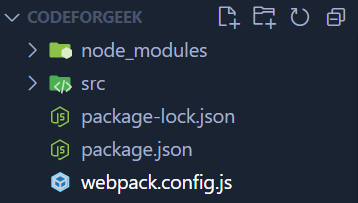
Step 5: Now we will bundle the JavaScript file using Webpack, by running this in the terminal:
npx webpack
Here, Node.js provides the runtime environment needed to implement Webpack since it is a Node.js based tool. When we run Webpack from the command line using npx webpack, Node.js executes the Webpack CLI (webpack-cli), which in turn bundles the JavaScript files.

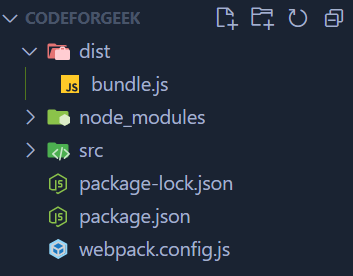
Step 6: Now we should make an HTML file to use our bundled Javascript file. So in the dist folder, we will add index.html with the given code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Webpack and Node.js Example</title>
</head>
<body>
<script src="bundle.js"></script>
</body>
</html>
Step 7: Next we need to run this HTML file with Node.js HTTP Server. We can do so by running the following command:
http-server
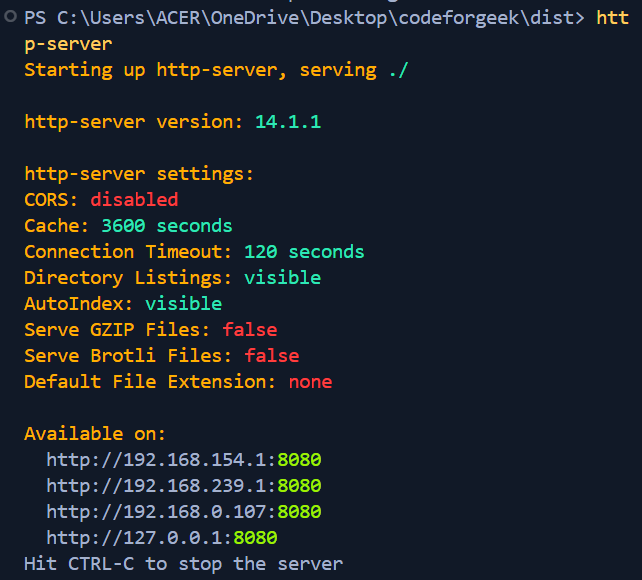
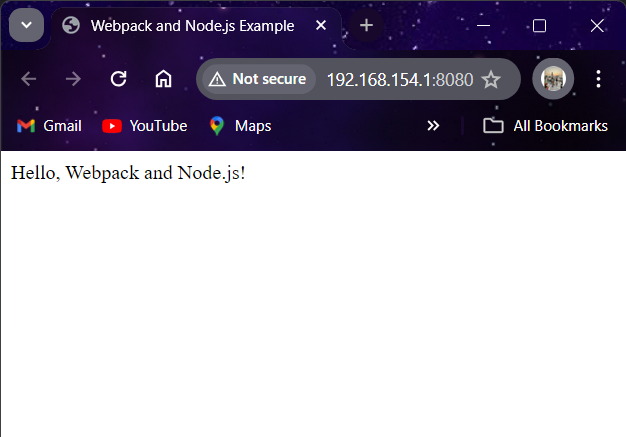
And there we go! Our bundled Javascript file runs successfully with the HTML file when we initiate a server within the dist folder, and the success message “Hello, Webpack and Node.js!” gets displayed on the browser. This example proves the efficiency of Node.js in frontend projects.
Node.js for Backend:
Node.js can be used to create a backend server, let us understand with an example project. We will create a server that can handle HTTP requests and send responses. Here is the stepwise procedure:
Step 1: We create a Node.js project using the given command.
npm init -y
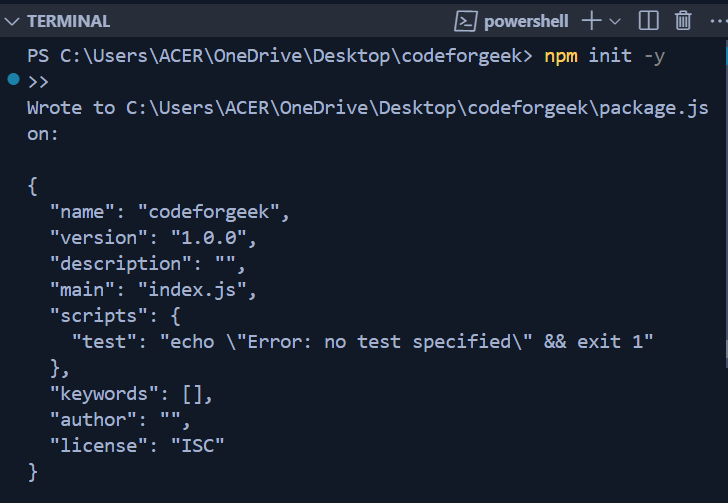
Step 2: Now we make a JavaScript file named server.js with the following content:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello from the Node.js backend!');
});
const port = 3000;
server.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
In this code, we first import the http module, then create a server and set a response message. We also specify the port number where our server would run. Lastly, we add a command to listen and display the running server message.
Step 3: We can test our server to see whether it is running or not by using the given command:
node server.js

This will display our server being run at localhost:3000.
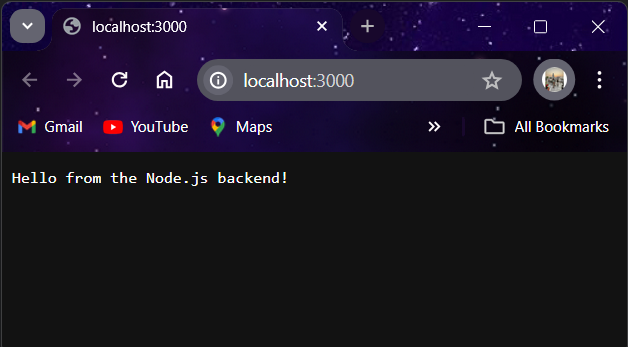
And yes! This is how Node.js helps us in backend. If you wish to learn more about HTTP and requests, Click Here!
Conclusion
Well, there are endless projects and examples where Node.js is implemented in full-stack. Here, we have used very basic and simple demonstrations to display the functionality of Node.js in both the frontend and backend. It should be noted that Node.js is primarily used in backend projects to help with servers and APIs and there are fewer cases of its use in the frontend, but that doesn’t defy the fact that it can be implemented with both to provide the user with ease of coding on the web.
Reference
https://stackoverflow.com/questions/69716356/fundamental-understanding-of-node-js-in-the-front-end