Node.js is an asynchronous event-driven Javascript runtime used to build different kinds of applications. It helps us use JavaScript for behind-the-scenes tasks like implementing server-side logic, building APIs, handling database operations, handling requests etc. Using Node.js we can also make HTTP post requests.
HTTP stands for Hyper Text Transfer Protocol, this is a request-response protocol within the client-server model. In Node.js HTTP requests enables clients to request data over the web to help interact with servers. Requests are a way to exchange information between clients and servers in which the client first makes a request to the server asking for data or information as a response from it.
This article guides you through making POST requests in Node.js. We will be using both the built-in HTTP module and the third-party library called request for this.
Making POST Requests with HTTP Module
The HTTP module in Node.js is a built-in library used to make HTTP requests. Using this we can develop a server that will listen for requests and also will be able to make requests.
To simplify the process of making POST requests with the HTTP module let us break the syntax into steps:
Step 1: Require the HTTP module in the server file (such as app.js)
const http = require("http");
Step 2: Create a body object to make the POST request
let body = JSON.stringify({
title: "Make a request with Node's http module"
})
Step 3: Specify the configurations for the request
let options = {
hostname: "your-url-here",
path: "/post",
method: "POST",
headers: {
"Content-Type": "application/json",
"Content-Length": Buffer.byteLength(body)
}
}
Step 4: Create a request with the HTTP modules’s request method and handle the response and error
http.request(options, res => {
let data = ""
res.on("data", d => {
data += d
})
res.on("end", () => {
console.log(data)
})
})
.on("error", console.error)
.end(body)
Example of Making POST Requests using HTTP Module
Let’s now modify the code with some real API and data for testing.
const http = require("http")
let body = JSON.stringify({
title: "Make a request with Node's http module"
})
let options = {
hostname: "postman-echo.com",
path: "/post",
method: "POST",
headers: {
"Content-Type": "application/json",
"Content-Length": Buffer.byteLength(body)
}
}
http
.request(options, res => {
let data = ""
res.on("data", d => {
data += d
})
res.on("end", () => {
console.log(data)
})
})
.on("error", console.error)
.end(body)
Output:
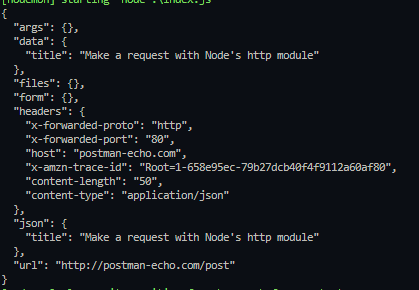
Making POST Requests with NPM Request Module
The request module is an external library/dependency used to make HTTP requests to the server. It is one of the simplest ways to make HTTP calls in Node.js. The request is a third-party package that can be installed using NPM.
NPM (Node Package Manager) is an ecosystem that contains a lot of libraries and dependencies that help us level up our Node.js applications.
Let us dive deep into it and understand the working, installation & implementation of the request module:
Step 1: Install the request module
npm install request
Step 2: Require “request” module
const request = require("request");
Step 3: Use the post() method in the request module for making POST requests
request.post(
// The first parameter is to be your API_URL
'http://your_api_here.com',
// The second parameter here has to be the data to be posted
{
json : {
name: "YOUR_DATA",
movies: ["data1", "data2"]
}
},
// The third parameter here has to be a callback function
function (error, response, body){
// printing body if no errors encountered
if(!error && response.statusCode == 201){
console.log(body)
}
}
);
Example of Making POST Requests using Request Module
Let’s now modify the code with some real API and data for testing.
const request = require('request');
request.post(
// The first parameter is to be your API_URL
'https://reqres.in/api/users',
// The second parameter here has to be the data to be posted
{
json : {
name: "Ronak A. Bhanushali",
movies: ["Interstellar", "Inception"]
}
},
// The third parameter here has to be a callback function
function (error, response, body){
// printing body if no errors encountered
if(!error && response.statusCode == 201){
console.log(body)
}
}
);
Output:
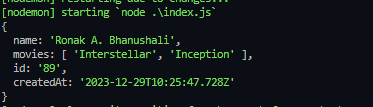
Summary
In this article, we were introduced to making POST requests in Node.js where we also went through the Node.js basics along with knowing about HTTP requests, further, we understood how to make POST requests and then implemented the same in 2 different ways using HTTP and request module.
We hope you enjoyed reading the content. If you want to delve more deeply into this concept and learn how to handle GET and POST requests, you can read our other article: Handle GET and POST Request in Express