NodeJS globals can be directly called inside any project without importing them. There are many built-in globals that provide different functions such as getting the directory name, and file name, printing different values in the console, setting timers, exporting and importing modules, etc. These globals can be a function, string, object, etc.
Different Node.js built-in Globals:
- __dirname
- __filename
- console
- process
- buffer
- setImmediate
- clearImmediate
- setInterval
- clearInterval
- setTimeout
- clearTimeout
- export
- require
__dirname
This global object is used to get the current working directory.
Example:
console.log("Current Directory is: " + __dirname);
Output:
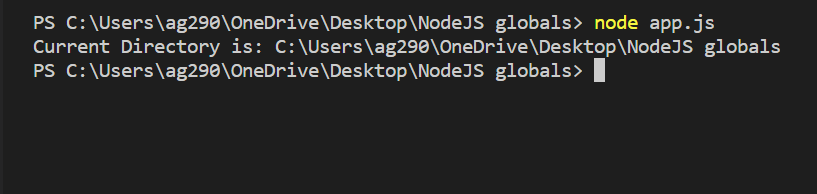
__filename
This global object is used to get the current working file name path.
Example:
console.log("Current File Path is: " +__filename);
Output:

console
This global object is used to print different types of values in the console.
Example:
console.log("Hello World!");
Output:
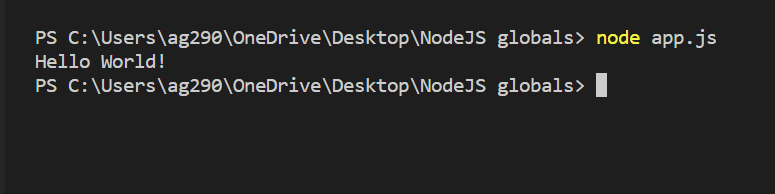
process
This global object is used to handle the currently running processes. The process object provides us with various methods such as process.exit, process.stdout, process.stdin, etc.
Example:
process.stdout.write("Hello World!");
Output:
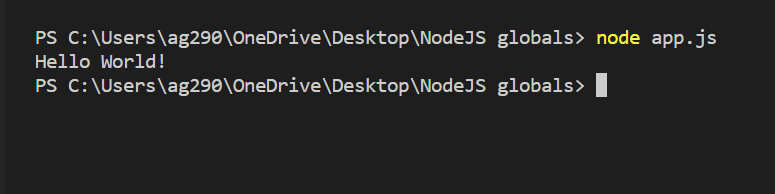
buffer
This global object is used to handle streams of binary data.
Example:
In general, a character takes a byte of space, so if we pass a string of 5 characters “hello” the buffer.byteLenght method will return 5 as output.
const bytes = Buffer.byteLength("hello");
console.log(bytes);
Output:
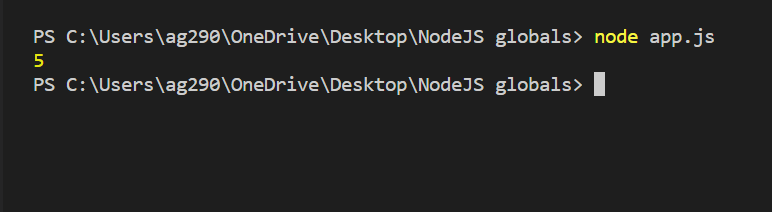
setImmediate
This global method is used to define a callback function that runs immediate after the execution.
Syntax:
setImmediate(callback)
Example:
const fun = setImmediate(function() { console.log("Immediate"); });
Here we define a callback function that uses the console global object to print a string immediately when the application runs.
Output:
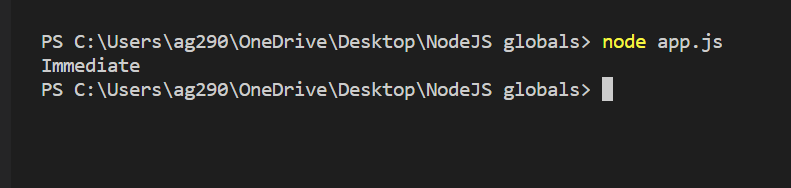
clearImmediate
This global method is used to terminate the execution of the callback function defined by the setImmediate method.
Syntax:
clearImmediate(immediateObject)
Example:
const fun = setImmediate(function() { console.log("Immediate"); });
clearImmediate(fun);
The clearImmediate method will stop the execution of setImmediate so we get nothing as an output.
Output:
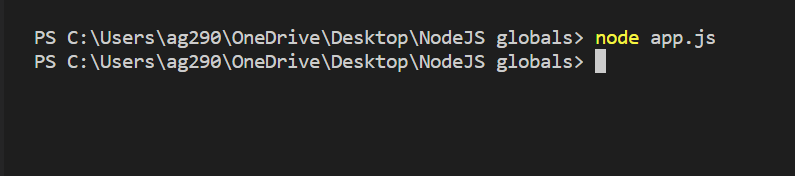
setInterval
This global method is used to define a callback function that runs at a definite interval until its termination.
Syntax:
setInterval(callback, delay)
Example:
setInterval(function() {
console.log("Running in interval of 1 millisecond!");
}, 1000);
Here we define a callback function that uses the console global object to print a string at an interval of 1 millisecond.
Output:
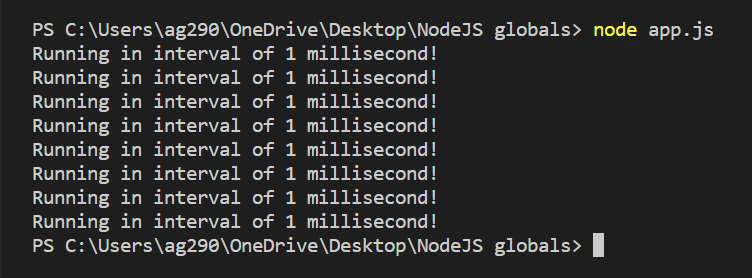
clearInterval
This global method is used to stop the execution of the callback function defined by the setInterval method.
Syntax:
clearInterval(intervalObject)
Example:
var x = 0;
const fun = setInterval(function() {
x = x + 1;
console.log(x);
if(x == 5) {
clearInterval(fun);
};
}, 1000);
Here we use the setInterval method to change the value of a variable x and print it and if the value becomes 5 then the clearInterval method stops its execution.
Output:
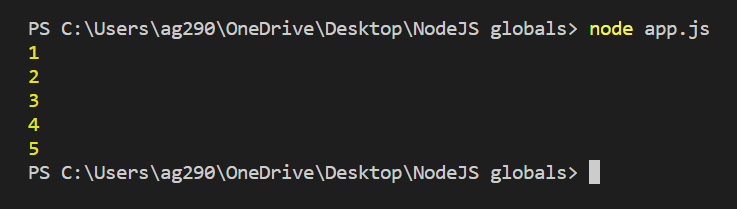
setTimeout
This global method is used to define a callback function that runs after a definite time.
Syntax:
setTimeout(callback, delay)
Example:
setTimeout(function() {
console.log("1 millisecond has passed!");
}, 1000);
Here we define a callback function that uses the console global object to print a string after 1 millisecond.
Output:
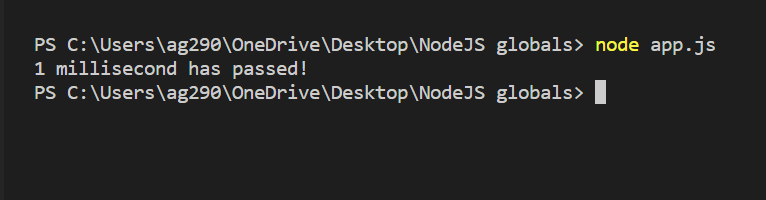
clearTimeout
This global method is used to stop the execution of the callback function defined by the setTimeout method.
Syntax:
clearTimeout(timeoutObject)
Example:
var x = true;
const fun = setTimeout(function() {
console.log("1 millisecond has passed!");
}, 1000);
if(x){
console.log("X is true!");
clearTimeout(fun);
}
Here we use the setTimeout method to print a string after 1 millisecond, but if the value of a variable we define is true it will stop it so that it will not able to print the value after 1 millisecond.
Output:
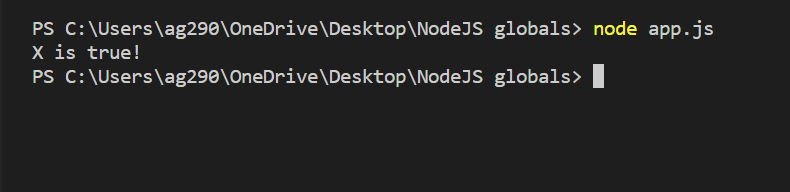
export
This global object is used to export a module or method in order to use it inside another file.
Example:
module.exports = "Hello World!";
We have a file hello.js in which we are exporting a string later on we will import it using require.
require
This is used to import a module or method by passing the location of that module or method as an argument to this method.
Example:
const value = require("./hello.js");
console.log(value);
Here we are importing the string export by hello.js file.
Output:
Hello World!
Summary
The global object is used to perform the different operations, they can directly call without importing them. We hope this tutorial helped you understand the concept of globals in Node.js.
Reference
https://nodejs.org/api/globals.html