In machine learning, one ought to deal with a lot of data for analysis. Handling such gargantuan amounts of data might be a bit tricky but with the right functions, it is just a matter of time before we end up right where we want to be.
In this article, we shall explore one such function that will help the user to find whether the set of given values falls in between two given inputs. The function of interest is the between( ) function from the pandas library in Python & we shall have a look at it in great detail in the following sections.
- Syntax of Pandas Series.between( ) Function
- Using between( ) Function on a Series
- Exercising the inclusive Option in between( ) Function
Before moving any further let us import the pandas library using the below code:
import pandas as pd
Syntax of Pandas Series.between() Function
One can understand the workings of the between( ) function through its syntax which is given below. It contains all the basic constituents required for its effective functioning.
Syntax:
Series.between(left, right, inclusive = ‘both’)
Parameters:
- left – input list or scalar entity which is declared to be one end of the boundary
- right – input list or scalar entity which is declared to be the other end of the boundary
- inclusive – an optional construct set to both by default and is used to include or exclude the boundary values before comparing the inputs against the boundaries
Using between() Function on a Series
In this section, we shall construct a series against which we shall apply the between( ) function to find which amongst the values in the series fall between the selected boundaries.
Given below is the code to create a series using the pandas library in Python:
sr1 = pd.Series([12, 36, 71, 99, 34, 28])
Once done, the above series is then passed through the between( ) function as shown below:
R = sr1.between(2,50)
print(R)
Output:
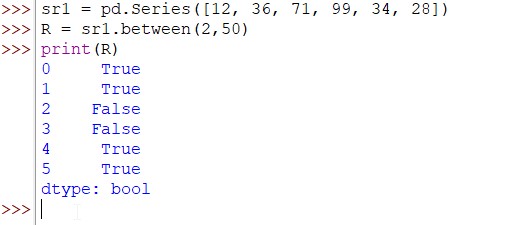
As seen in the above output, the between( ) function returns the Boolean value as a result for each entity in the series by comparing whether or not it lies within the specified boundaries.
Exercising the inclusive Option in between() Function
In this section, we shall make use of the optional construct in the syntax of the between( ) function to demonstrate its different variations.
The inclusive option in this function can be deployed in three different ways, each of which is listed below:
- Both – Both the boundary values are considered while analysing the input series
- Neither – Both the boundary values are not considered while analysing the input series
- Left – Only the left side boundary value is considered while ignoring the right side boundary value while analysing the input series
- Right – Only the right side boundary value is considered leaving aside the left side boundary value while analysing the input series
Let us now have a look at examples for different types to understand the inclusive option better.
Example 1:
sr2 = pd.Series([5, 12, 36, 71, 99, 50, 34, 28])
R = sr2.between(5,50, inclusive = 'both')
print(R)
Output:
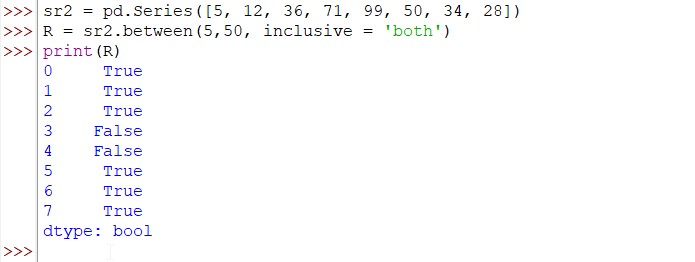
From the above result, it is evident that both boundary values 5 & 50 are included in the search thereby returning True for the 0th and 5th positions of the input series.
Example 2:
Let us now set the option as neither and see what happens.
R = sr2.between(5,50, inclusive = 'neither')
print(R)
Output:
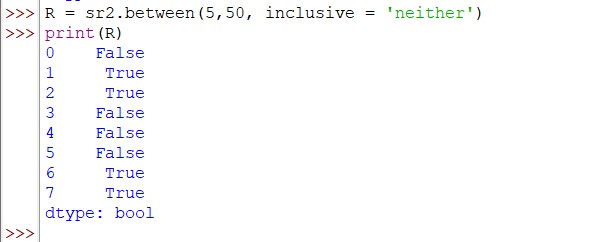
Contrary to the previous results, it could be seen that the results are returned as False in both the 0th & 5th positions of the input series since neither of the boundary values are being considered in this case. The same can be expected for the leftmost boundary value is not considered when the left option is deployed or vice versa in the case of the right being deployed.
If you’re new: Indentation in Python
Conclusion
Now that we have reached the end of this article, hope it has elaborated on the different ways of using the between( ) function from the pandas library. Here’s another article that explains how to use the sqrt( ) function from the numpy library in Python. There are numerous other enjoyable & equally informative articles in CodeforGeek that might be of great help to those who are looking to level up in Python.
Reference
https://pandas.pydata.org/docs/reference/api/pandas.Series.between.html