The date_range() function is particularly useful for a wide range of use cases in Python. Whenever we want to iterate over a date range we can use the date_range() function which permits us to perform some particular tasks on every date within the range. This can be useful for generating the reports and for performing the calculations which are based on the date intervals. Using this function we can filter data within a particular date range which makes it ready for querying databases. Also, it plays an important role in scheduling the events for generating the recurring events in a calendar format.
In this article, we will look at a few ways to generate a date range using the Pandas date_range() function. Let’s get started.
Also Read: Create a Pandas DataFrame from Lists
Pandas date_range() Method
In Python, the date_range() function allows us to generate a sequence of dates within a specified range. It is a powerful tool for working with time-based data and performing date-specific operations.
Syntax:
pandas.date_range(start=None, end=None, periods=None, freq=None, tz=None, normalize=False, name=None, closed=None)
Parameters:
- start: The start date of the date range. Can be a string, datetime, or Timestamp object. If not specified, it defaults to None.
- end: The end date of the date range. Can be a string, datetime, or Timestamp object. If not specified, it defaults to None.
- periods: The number of periods to generate. If start and end are not provided, periods must be specified. The date range will be generated using equally spaced intervals between the start and end.
- freq: The frequency of the date range. It can be a string or a DateOffset object, specifying the intervals between dates. Common values include ‘D’ for daily, ‘W’ for weekly, ‘M’ for monthly, ‘Y’ for yearly, etc.
- tz: The time zone to be used for the date range.
- normalize: If True, the date range will be normalized to midnight. If False, the date range will include the exactly specified time.
- name: A name to assign to the generated DatetimeIndex.
- closed: Specify which side of the date range interval should be closed (left, right, both, or neither).
Creating a Date Range Using Pandas date_range() Method
The pandas.date_range function allows us to create date ranges in various ways which few ways are as follows:
- By specifying the start and end
- By specifying start, end, and freq
- By specifying start, periods, and freq
- By specifying start, periods, and tz
- By specifying start and periods
1. By specifying the start and end
This will generate a range of dates from start to end with a by-default freq.
Example:
import pandas as pd
dateRange = pd.date_range(start = "07-01-2022", end = "07-31-2022")
print(dateRange)
First of all, we have imported the pandas library as pd. Then we have provided the starting position and our ending position means start date and end date in pd.date_range( ) function and stored it into the dateRange variable. Then we printed the dateRange variable.
Output:
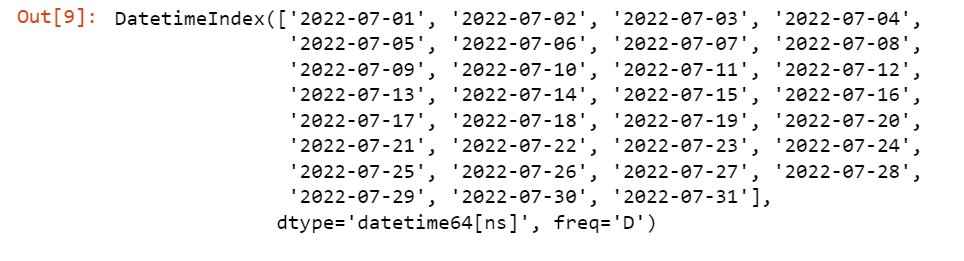
We can see in the output that the date_range( ) function has printed all the dates between the specific dates and we can also see that by default frequency is D.
2. By specifying start, end, and freq
This will generate a range of dates from start to end with a specified freq.
Example:
import pandas as pd
dateRange = pd.date_range(start ="01-01-2022", end ="12-31-2022", freq ="M")
print(dateRange)
Output:

Here we got the last days of a respective month.
3. By specifying start, periods, and freq
This will generate a range of dates from the start with a fixed number of periods and with the specified freq.
Example:
import pandas as pd
dateRange = pd.date_range(start ="01-01-2022", periods=6, freq ="2M")
print(dateRange)
Output:

Here we got the specific date based on a specific period and frequency.
4. By specifying start, periods and tz
This will generate a range of dates from the start with a fixed number of periods and with the specified timezone tz.
Example:
import pandas as pd
dateRange = pd.date_range(start ="01-01-2022", periods=6, tz="Asia/Tokyo")
print(dateRange)
Output:

5. By specifying start and periods
This will generate a range of dates from the start with a fixed number of periods.
Example:
import pandas as pd
dateRange = pd.date_range(start ="2022-1-1",periods=7)
print(dateRange)
Output:

Conclusion
Different libraries offer different features and functionalities for date range generation. But, the pandas.date_range() function in the Pandas library provides robust tools for creating date ranges with various frequencies and handling date-related operations efficiently. In this article, we have discussed five ways of generating a date range using it with examples. After reading this article, we hope you can easily generate a date range in Python.
Reference
https://stackoverflow.com/questions/13445174/date-ranges-in-pandas