Hello folks! In today’s guide, we will be learning about NumPy’s nanmax function. The nanmax function is helpful because it finds the biggest number in a dataset while ignoring any missing or undefined values. This ensures that missing data doesn’t affect our results, making calculations more accurate, especially in science and statistics where missing data is common.
Also Read: How to Find the Product of Array Elements in Python (3 Easy Ways)
Introducing numpy.nanmax
NumPy is the best option in Python for the mathematical computation of arrays. It helps with big arrays of numbers and does lots of math quickly. One of the functions it has is called “nanmax“.
nanmax means “NaN maximum“. It finds the biggest number in an array but ignores any NaN (Not a Number) values. NaN values usually show where data is missing or doesn’t make sense in math. This function is helpful when you’re dealing with data that has missing numbers.
Syntax of numpy.nanmax
The root syntax for the Numpy nanmax looks like this:
numpy.nanmax(array, axis=None, keepdims=False)
Let’s understand what each parameter means:
- array: It is the list of numbers we’re looking at.
- axis (optional): Tells us which way to look for the biggest number. If not given, it looks for the biggest number in the whole list.
- keepdims (optional): If we say “True” the size of the result will match the size of the original list, with any reduced parts being just 1 long.
How numpy.nanmax Works
As we discussed earlier, this function ignores NaN values and returns the maximum number. It treats NaN values as if they don’t exist for the purpose of finding the maximum. For example, we have an array [1, 2, NaN, 4, NaN, 6, NaN, 8, 9]. With only the array parameter provided, 9 will be returned as the maximum number.
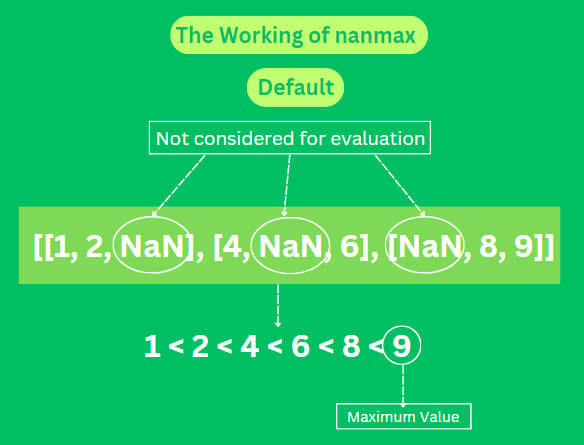
If the axis parameter is provided, nanmax computes the maximum value along the specified axis. If we specify axis=0, nanmax will find the maximum value along the columns (down the rows). In this case, the maximum values along the columns are [4., 8., 9.]
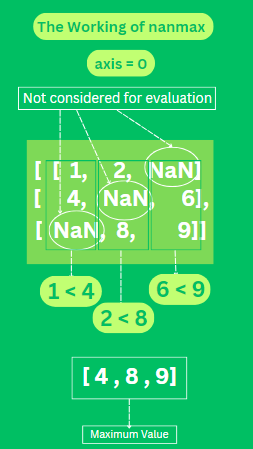
If we specify axis=1, nanmax will find the maximum value along the rows (across the columns). In this case, the maximum values along the rows are [2., 6., 9.]
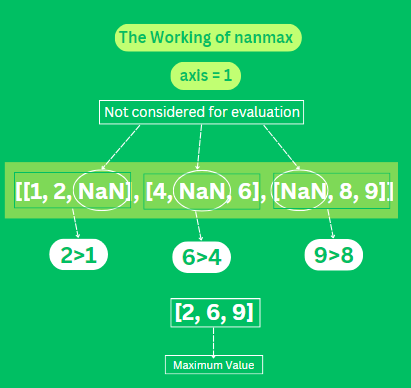
Using numpy.nanmax in Python
Now that we know how this function works, let’s see its code implementation.
Example 1: Let’s check the default working of this function.
import numpy as np
arr = np.array([[1, 2, np.nan],
[4, np.nan, 6],
[np.nan, 8, 9]])
max_val = np.nanmax(arr)
print("Maximum value ignoring NaN:", max_val)
Output:
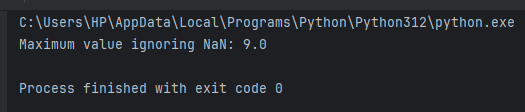
Example 2: Let’s use the axis parameter with this function.
import numpy as np
arr = np.array([[1, 2, np.nan],
[4, np.nan, 6],
[np.nan, 8, 9]])
max_col = np.nanmax(arr, axis=0)
print("Maximum value along columns:", max_col)
max_row = np.nanmax(arr, axis=1)
print("Maximum value along rows:", max_row)
Output:
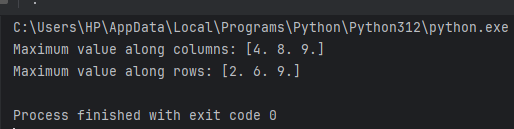
Example 3: If the entire array consists of NaN values, nanmax returns NaN.
import numpy as np
arr = np.array([[np.nan, np.nan, np.nan],
[np.nan, np.nan, np.nan],
[np.nan, np.nan, np.nan]])
max_val = np.nanmax(arr)
print("Maximum value ignoring NaN:", max_val)
Output:

Example 4: Let’s test it with Positive and Negative Numbers:
import numpy as np
data = np.array([5, -5, np.nan, 10, -1])
max_value = np.nanmax(data)
print("Maximum value (ignoring NaN values):", max_value)
nanmax considers all numerical values, including negatives, and returns the maximum value while disregarding any NaNs.
Output:
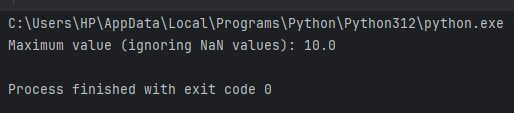
Example 5: Let’s see what happens with an Empty Array:
import numpy as np
data = np.array([])
max_value = np.nanmax(data)
print("Maximum value in an empty array:", max_value)
This code will show an error since there are no values to check on.
Output:
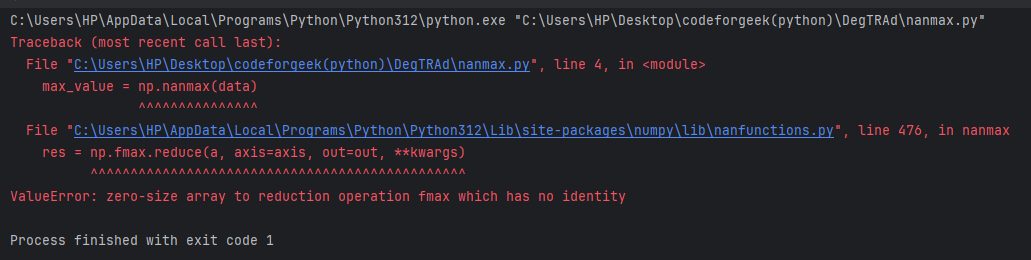
Summary
So that’s it for this article. I hope that now you know how you can utilize the nanmax() function efficiently. In this article, we discussed its importance, and how it works, along with a bunch of useful and good examples that would have clarified your concepts even more. Now it’s your time to experiment with this NumPy function in your code and explore its benefits.
Did you know NumPy has more functions to check maximum and minimum values like fmax() and fmin()? If not, do check them out!
Reference
https://numpy.org/doc/stable/reference/generated/numpy.nanmax.html