The numpy.full_like() function in NumPy is designed to create a new array with the same shape and data type as a given input array with all elements set to a specified fill value. This function is beneficial when we want to initialize an array with a constant value and want the new array to have the same shape and data type as an existing array.
The numpy.full_like() function is further detailed through each of the following sections:
- Syntax of numpy.full_like() function
- Examples of numpy.full_like() function
- Difference between numpy.full() and numpy.full_like() function
Syntax of numpy.full_like() Function
The syntax is similar to numpy.zeros() but allows us to specify a fill value other than zero. It provides a convenient way to maintain consistency in array structure while initializing elements with a specific constant value.
Syntax:
numpy.full_like(a, fill_value, dtype=None, order='K', subok=True, shape=None)
Parameters:
- a: The input array whose shape and datatype will be used to create the new array.
- fill_value: The constant value to fill the array with.
- dtype (optional): The desired datatype for the new array. If not specified, the datatype of the input array is used.
- order (optional): Specifies the memory layout of the result. The default is ‘K, ‘ meaning the input array’s memory layout determines the order.
- subok (optional): If True, sub-classes will be passed through. If False, the returned array will always be a base-class array.
- shape (optional): Deprecated parameter. If present, it overrides the shape of the input array.
Return:
A new array with the same shape and data type as the input array, filled with the specified constant value.
Examples of numpy.full_like() Function
Let us now look at some examples to demonstrate the use of numpy.full_like() function in Python.
Example 1: Creating a 1-D Array Filled with Constant Value Using numpy.full_like() function
Let’s start by passing the one-dimensional array with a fill_value which is a constant value that will present in the output array.
import numpy as np
A = np.array([2,45,11,6])
B=np.full_like(A,7)
print("Input Array: ",A)
print("Output Array: ",B)
Here we first imported the NumPy library as np then using it we created a one-dimensional array and saved it into a variable called A. Then we passed the array A with a constant value of 7 into the numpy.full_like() function which means we will get a new array of the same shape and data type as array A where 7 will be written at each position in the new array created.
Output:

We got a one-dimensional array where 7 is a constant value at each position in the output array with the same shape and integer data type as array A.
Example 2: Creating a 3-D Array Filled with Negative Value and Integer Data Type
In this example we will try to create a three-dimensional array where the constant value will be negative, with this we will also try to change the default data type to an integer data type as by default the data type of the output array is the same as the data type of the input array.
import numpy as np
from numpy import random
C=np.random.randn(2,3,4)
D=np.full_like(C,-71,dtype=int)
print("Input Array: ",C)
print("Output Array: ",D)
Here we have additionally imported the random module from the NumPy library so that we can create a three-dimensional random array using the randn function. Inside the np.full_like function, we passed a negative constant value -71 with this random array C and dtype as int to convert the data type of the output array from float to integer.
Output:
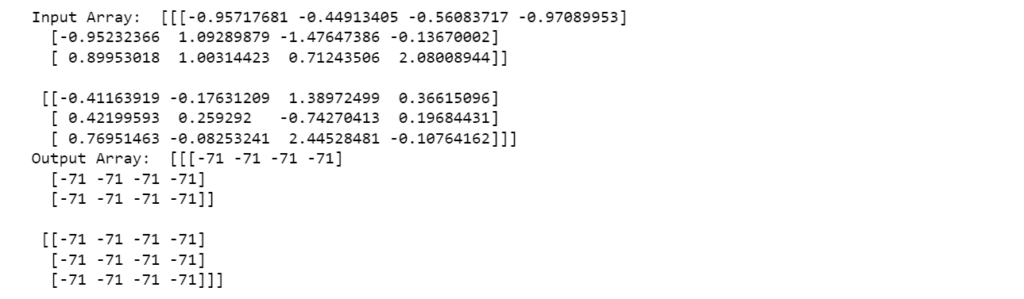
We can see that the input array C is of data type float and the output array data type got changed to an integer.
Difference Between numpy.full() and numpy.full_like() Functions
The numpy.full() function creates a new array with a specified shape and fills it with a constant value (fill_value) while the numpy.full_like() function creates a new array with the same shape and data type as the input array and fills it with a constant value (fill_value).
The numpy.full() function requires us to explicitly specify the shape of the new array while numpy.full_like() uses the shape and data type of the input array to create a new array.
Now when we talk about the shape specification, the numpy.full() function directly takes the shape as its first parameter while numpy.full_like() function extracts the shape from the input array but allows us to override it using the shape parameter as well.
Summary
In this tutorial, we have discussed numpy.full_like() function provided by Python’s NumPy library. We have explored how to create a single and multi-dimensional full array using numpy.full_like() function. With this, we have also understood the difference between numpy.full() function and numpy.full_like() function. CodeForGeek has many other entertaining and equally informative articles that can be of great help to those who want to advance in Python, so be sure to check them out as well.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.full_like.html